Question
Overview You will write a program that simulates the act of moving around windows on your computer. For example, you may have an internet browser,
Overview
You will write a program that simulates the act of moving around windows on your computer. For example, you may have an internet browser, Eclipse, and iTunes each open in separate windows, and you can click on each window to bring that window to the front and you can drag the windows around the screen. A single "window" in this case will be a single rectangle.
You will utilize an ArrayList to store the windows. The order of the windows in the list implies the order in which they would display on the screen. This is sometimes referred to as the "z-order". Each window in the list has what is called a "z-index", which corresponds to where it is in order of visibility. That is, the window at z-index 0 should be at the back and the window at the last z-index in the list should be at the front. When a user clicks on a window, it will move to the top. When windows overlap, only the top window among the overlapping windows at the location the user clicked is considered to have been clicked.
Classes
Your project should have three classes: Window, WindowDisplay, and WindowDriver. Window will be an inner class inside of WindowDisplay, so you will only be defining two .java files. You will also need to include the Draw and DrawListener classes in your project. Simply download the .java files and place them in the same package as the .java files you define.
Draw.java (Links to an external site.)Links to an external site.
DrawListener.java (Links to an external site.)Links to an external site.
In order for Draw.java to work, you will still need to add StdDraw to your project as we've been doing throughout the quarter. However, you will not be calling methods on StdDraw in this project. You will use the same methods as before, but on an instance of Draw.
Draw.java is very similar to StdDraw. If you check the documentation (Links to an external site.)Links to an external site., you will find that they have many variables and methods in common. The key difference is that StdDraw contains only static methods, so we call methods on the StdDraw class. Draw.java, on the other hand, requires you to create an instance of Draw in order to call the analogous methods like filledRectangle() and setPenColor() (meaning these methods are not static). Also, Draw.java comes with DrawListener, which we are using to handle mouse and keyboard input.
WindowDisplay
The WindowDisplay class will implement DrawListener and will contain an inner class, Window. It will keep a list of all windows and update the list when the mouse is clicked on a specific window.
Instance variables:
-
A private ArrayList of windows
- A private instance of Draw
Methods:
-
A constructor that takes two arguments, the x-scale and y-scale of the Draw canvas. The constructor should initialize a new Draw object and should create an empty list. It should also set the Draw canvas size and the x- and y-scale.
-
An addWindow() method that takes in 5 parameters, each corresponding to the instance variables needed to create a Window object. The addWindow method should create a new window and add it to the list of windows.
-
A draw() method that will draw all the windows.
- A reset() method that will set all windows back to their original position, including their original relative order.
It should also implement methods from the DrawListener interface so that the display responds in the following way:
- When a window is clicked, it moves to the top.
- A window can also be dragged.
- When the 'R' key is pressed, the windows reset.
- When the 'Q' key is pressed, the program quits.
You may find that you do not need some of the methods in DrawListener. That is fine. Those methods definitions will simply contain no code.
Window
The inner class Window will represent a single window. It should contain the following:
Instance variables:
-
Two private instance variables of type double for the x- and y-coordinates of the center of the window.
-
Two private instance variables of type double for the width and height of the window.
-
A private variable of type Color for the color of the window. (You will need to import java.awt.Color.)
Methods:
-
A constructor that takes in x- and y-coordinates, width, height, and color of the window. This constructor should use each parameter to set the instance variables.
-
A method that displays (draws) a single window.
-
A method that has two parameters, an xcoordinate and a y-coordinate. This method should return true if the x-coordinate and y-coordinate are located within the window and false if they are not.
The instance variables and methods for Window and WindowDisplay listed above are what you need at a minimum in order to implement this program properly. You will likely find that you need to define additional variables and methods in one of or both classes.
WindowDriver
The Driver should create a new display, add some windows of varying size and color, then draw the display.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
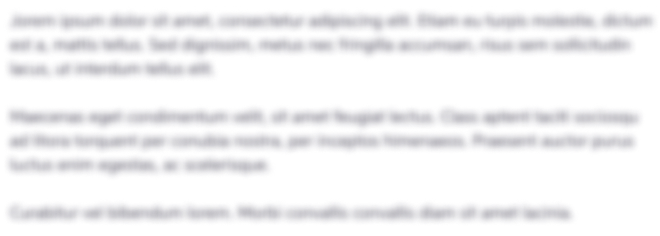
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started