Answered step by step
Verified Expert Solution
Question
1 Approved Answer
package a5; import javax.sound.sampled.Clip; import java.net.MalformedURLException; import java.io.File; import java.io.ByteArrayInputStream; import java.io.InputStream; import java.io.IOException; import java.net.URL; import javax.sound.sampled.AudioFileFormat; import javax.sound.sampled.AudioFormat; import javax.sound.sampled.AudioInputStream; import javax.sound.sampled.AudioSystem; import
package a5; import javax.sound.sampled.Clip; import java.net.MalformedURLException; import java.io.File; import java.io.ByteArrayInputStream; import java.io.InputStream; import java.io.IOException; import java.net.URL; import javax.sound.sampled.AudioFileFormat; import javax.sound.sampled.AudioFormat; import javax.sound.sampled.AudioInputStream; import javax.sound.sampled.AudioSystem; import javax.sound.sampled.DataLine; import javax.sound.sampled.LineUnavailableException; import javax.sound.sampled.SourceDataLine; import javax.sound.sampled.UnsupportedAudioFileException; public final class StdAudio { public static final int SAMPLE_RATE = 11025;//44100; private static final int BYTES_PER_SAMPLE = 2; // 16-bit audio private static final int BITS_PER_SAMPLE = 16; // 16-bit audio private static final double MAX_16_BIT = Short.MAX_VALUE; // 32,767 private static final int SAMPLE_BUFFER_SIZE = 4096; private static SourceDataLine line; // to play the sound private static byte[] buffer; // our internal buffer private static int bufferSize = 0; // number of samples currently in internal buffer private StdAudio() { // can not instantiate } // static initializer static { init(); } // open up an audio stream private static void init() { try { // 44,100 samples per second, 16-bit audio, mono, signed PCM, little Endian AudioFormat format = new AudioFormat((float) SAMPLE_RATE, BITS_PER_SAMPLE, 1, true, false); DataLine.Info info = new DataLine.Info(SourceDataLine.class, format); line = (SourceDataLine) AudioSystem.getLine(info); line.open(format, SAMPLE_BUFFER_SIZE * BYTES_PER_SAMPLE); buffer = new byte[SAMPLE_BUFFER_SIZE * BYTES_PER_SAMPLE/3]; } catch (LineUnavailableException e) { System.out.println(e.getMessage()); } // no sound gets made before this call line.start(); } public static void close() { line.drain(); line.stop(); } public static void play(double sample) { // clip if outside [-1, +1] if (Double.isNaN(sample)) throw new IllegalArgumentException("sample is NaN"); if (sample +1.0) sample = +1.0; // convert to bytes short s = (short) (MAX_16_BIT * sample); buffer[bufferSize++] = (byte) s; buffer[bufferSize++] = (byte) (s >> 8); // little Endian // send to sound card if buffer is full if (bufferSize >= buffer.length) { line.write(buffer, 0, buffer.length); bufferSize = 0; } } public static void play(double[] samples) { if (samples == null) throw new IllegalArgumentException("argument to play() is null"); for (int i = 0; i > 8); } // now save the file try { ByteArrayInputStream bais = new ByteArrayInputStream(data); AudioInputStream ais = new AudioInputStream(bais, format, samples.length); if (filename.endsWith(".wav") || filename.endsWith(".WAV")) { AudioSystem.write(ais, AudioFileFormat.Type.WAVE, new File(filename)); } else if (filename.endsWith(".au") || filename.endsWith(".AU")) { AudioSystem.write(ais, AudioFileFormat.Type.AU, new File(filename)); } else { throw new IllegalArgumentException("unsupported audio format: '" + filename + "'"); } } catch (IOException ioe) { throw new IllegalArgumentException("unable to save file '" + filename + "'", ioe); } } public static synchronized void play(final String filename) { if (filename == null) throw new IllegalArgumentException(); InputStream is = StdAudio.class.getResourceAsStream(filename); if (is == null) { throw new IllegalArgumentException("could not read '" + filename + "'"); } // code adapted from: http://stackoverflow.com/questions/26305/how-can-i-play-sound-in-java try { AudioSystem.getAudioInputStream(is); new Thread(new Runnable() { @Override public void run() { stream(filename); } }).start(); } // let's try Applet.newAudioClip() instead catch (UnsupportedAudioFileException e) { // playApplet(filename); return; } // something else went wrong catch (IOException ioe) { throw new IllegalArgumentException("could not play '" + filename + "'", ioe); } } private static void stream(String filename) { SourceDataLine line = null; int BUFFER_SIZE = 4096; // 4K buffer try { InputStream is = StdAudio.class.getResourceAsStream(filename); AudioInputStream ais = AudioSystem.getAudioInputStream(is); AudioFormat audioFormat = ais.getFormat(); DataLine.Info info = new DataLine.Info(SourceDataLine.class, audioFormat); line = (SourceDataLine) AudioSystem.getLine(info); line.open(audioFormat); line.start(); byte[] samples = new byte[BUFFER_SIZE]; int count = 0; while ((count = ais.read(samples, 0, BUFFER_SIZE)) != -1) { line.write(samples, 0, count); } } catch (IOException e) { e.printStackTrace(); } catch (UnsupportedAudioFileException e) { e.printStackTrace(); } catch (LineUnavailableException e) { e.printStackTrace(); } finally { if (line != null) { line.drain(); line.close(); } } } public static synchronized void loop(String filename) { if (filename == null) throw new IllegalArgumentException(); try { Clip clip = AudioSystem.getClip(); InputStream is = StdAudio.class.getResourceAsStream(filename); AudioInputStream ais = AudioSystem.getAudioInputStream(is); clip.open(ais); clip.loop(Clip.LOOP_CONTINUOUSLY); } catch (UnsupportedAudioFileException e) { throw new IllegalArgumentException("unsupported audio format: '" + filename + "'", e); } catch (LineUnavailableException e) { throw new IllegalArgumentException("could not play '" + filename + "'", e); } catch (IOException e) { throw new IllegalArgumentException("could not play '" + filename + "'", e); } } private static double[] note(double hz, double duration, double amplitude) { int n = (int) (StdAudio.SAMPLE_RATE * duration); double[] a = new double[n+1]; for (int i = 0; iFor this assignment you will practice using arrays to solve a variety of problems. Then you will see how sound can be represented as large arrays. The sound can be manipulated by changing the values in the arrays Starting 1. In Eclipse, create a new project or use an assignments one. Add a package a5, and a class ArraysAndSound to that package 2. Download this StdAudio.java file e and add it to the a5 package. It is somewhat like the Picture.java, except it is for audio rather than imagery. 3. Download this audio file and add it to the package. 4. In your class, implement the static methods specified below 5. All methods you write should have a Javadoc comment with a description of what the method does and a @param for each parameter and a @return describing what information is returned. 6. Add a main method with tests for each method. Your tests should cover possible return values (such as true or false) as well as special cases (for example, empty strings). Your tests should print what was expected as well as the actual result from the method in a neatly formatted way. Note that you can test the sound processing methods without playing the sound - your code can send the method a small array of values and then can examine the produced array of sound samples to see if they are as expected 7. You do not need to test for cases that are prohibited in the description. If the description says that there is at least X in the parameter, then you do not need to test for the truth of that constraint. For all of the methods, you can assume a null value is not used as an argument to the method. 8. None of your methods except for main may print anything to the console, load image or sound files, or show or 9. Ensure you have consistent spacing and indenting in your file, proper and consistent indenting, and a Javadoc Required Methods play images or sounds comment about the start of the class definition with your name, assignment number and class. You must implement each of the described methods with method name, return type and parameters carefully checked against the specification. The post-condition is what happens to the parameters after the method executes. When parameters are specified you must use the order they are listed in. You may implement additional methods if they aid your problem-solving. You may not use Java methods that solve the entire problem for you 1. Method name: clearArray Parameter(s): An int array. Return value: Nothing. The method return type is void Post-Condition: The array parameter has every element in the array set to 0 Example: After calling clearArray([1, 2]), the array has its elements changed to [0,0] 2. Method name: arrayToString Parameter(s): An int array Return value: A String containing a textual representation of the parameter. The return String should start with a curly brace, then have all the numbers in the array with a comma and space between numbers, and then ending with an end curly brace. Arrays.toString or its variants should not be used in this method Post-Condition: The array parameter is not changed Example: Calling arrayToString([1, 2]) should return "[1, 2)". 3. Method name: containsDuplicate Parameter(s): A String array Return value: Returns boolean true if one element of the array is equal() to another element of the array and false otherwise Post-Condition: The array parameter is not changed Example: Calling containsDuplicate('David", "Joe") should return false. Calling containsDuplicate(l"a", "b", "a"] should return true 4. Method name: averageArrayValues Parameter(s): An int array of at least length 1 Return value: Returns a double value that is the sum of the array elements divided by the array length. The requirement of at least one value is to prevent a divide by zero possibility Post-Condition: The array parameter is not changed Example: Calling averageArrayValues([1,2]) should return 1.5 5. MethodName: frequencyCount Parameter(s): An int array. The elements of the array can only be numbers from 0 to 9 Return value: A new int array of 10 integers. The value in the new array at index n is the number of times value n appears in the parameter array Post-Condition: The array parameter is not changed Example: Calling frequencyCount([0,0,1,1,1,7]) would return [2,3,0,0,0,0,0,1,0,0] since there are 2 zeroes and 3 ones and 1 seven, and no other digits in the parameter array 6. Method name: reverseSound Parameter(s): A double array Return value: Returns a double array that has its elements in reversed order from the parameter array Post-Condition: The array parameter is not changed. Example: Calling reverseSound(.1, 02, 03, 0.5]) should return a new array [O.5. O.3, 0.2. O.1] 7. Method name: scaleSound Parameter(s): A double array and a double value Return value: Returns a double array that has as its elements each of the parameter array elements scaled by the second parameter. Post-Condition: The array parameter is not changed. Example: Caling scaleSound ([O.0, -0.1, 0.3], 2.0) should return a new array [0.0,-0.2,0.6] Note: The play method for sounds keeps all values in the required -1.0 to 1.0 range, you do not need to test for this like you did in the Picture brightening 8. Method name: echoSound Parameter(s): A double array, an int specifying how many samples offset the echo starts at, and a double giving a weight to the echo. The double array will have at least as many values as the offset. Return value: A double array as long as the array parameter plus the offset parameter. The first offset samples will be the same as the first offset samples as the parameter array. The last offset samples will be the same as the last offset samples from the parameter array scaled by the weight parameter. The middle samples will be the sample from the parameter array plus the sample from the parameter array back the offset amount scaled by the weight parameter. Here is the logic of it. An echo is a diminished sound delayed from bouncing off a distance object. So if you yell, I first hear your original signal. Then when the bounce comes back, I hear your original signal plus a scaled down signal from back in time. Then, when you stop, I only hear the scaled down signal from back in time. Post-Condition: The array parameter is not changed. Example: Calling echoSound([O.1, 0.2, 0.3, 0.4], 1, 0.5) should return a new array of length 5 (the original array plus the offset). The new array is [0.1, 0.25, 0.4, 0.55, 0.2]. The 0.1 is before the echo starts and is unchanged. The 0.25 comes from 0.2(0.1 0.5). The 0.4 comes from 0.3+(0.2 0.5). The 0.55 comes from 0.4 (0.3 0.5). The last value is all echo, and is 0.4* 0.5 9. Method name: smoothSound Parameter(s): A double array with at least 3 elements in it. Return value: Returns a double array that has as its elements a sliding average of the parameter array elements. More precisely: 1. The first and last elements of the new array are the same as the first and last elements of the parameter array, respectively 2. For the other elements of the new array, for an element at index i, it is equal to the average of elements from the parameter array at indices i-1,i, and i+1 Post-Condition: The array parameter is not changed Example: Calling smoothSound([0.0, 0.2, 0.7, 0.2]) should return a new array [0.0, 0.3, 0.3666..,.0.2]. When played, noise in the sound should be reduced If you want to hear the effect of these sound methods, you can add code in main like this double] samples StdAudio.readC"asyouwish2.wav); StdAudio.play (samples); double[] reversed -reverseSound(samples) StdAudio.play(reversed); Note that this isn't really a test - it is to help you appreciate the kinds of effects we can generate pretty easily in code. A test has an expected output for a given input to a method Submitting Submit your ArraysAndSound.java to gradescope. You will see some tests to help you understand if you are meeting the specification. Issues with StdAudio? A couple of people have had some issues using the "StdAudio.java" file The main issue seems to be eclipse complaining about importing javax.sound. Basically eclipse has underlined a bunch of lines of code in red and for some people running the main method produces an error import javaM.sound.sampled..CLR; If you are having issues, the fix below seems to be working OK: In the main menu bar navigate to project> properties > Java Build Path. Double click JRE System Library to open up a new window. Change the execution from CDC-1.0 to JavaSE-11 which is located at the bottom For this assignment you will practice using arrays to solve a variety of problems. Then you will see how sound can be represented as large arrays. The sound can be manipulated by changing the values in the arrays Starting 1. In Eclipse, create a new project or use an assignments one. Add a package a5, and a class ArraysAndSound to that package 2. Download this StdAudio.java file e and add it to the a5 package. It is somewhat like the Picture.java, except it is for audio rather than imagery. 3. Download this audio file and add it to the package. 4. In your class, implement the static methods specified below 5. All methods you write should have a Javadoc comment with a description of what the method does and a @param for each parameter and a @return describing what information is returned. 6. Add a main method with tests for each method. Your tests should cover possible return values (such as true or false) as well as special cases (for example, empty strings). Your tests should print what was expected as well as the actual result from the method in a neatly formatted way. Note that you can test the sound processing methods without playing the sound - your code can send the method a small array of values and then can examine the produced array of sound samples to see if they are as expected 7. You do not need to test for cases that are prohibited in the description. If the description says that there is at least X in the parameter, then you do not need to test for the truth of that constraint. For all of the methods, you can assume a null value is not used as an argument to the method. 8. None of your methods except for main may print anything to the console, load image or sound files, or show or 9. Ensure you have consistent spacing and indenting in your file, proper and consistent indenting, and a Javadoc Required Methods play images or sounds comment about the start of the class definition with your name, assignment number and class. You must implement each of the described methods with method name, return type and parameters carefully checked against the specification. The post-condition is what happens to the parameters after the method executes. When parameters are specified you must use the order they are listed in. You may implement additional methods if they aid your problem-solving. You may not use Java methods that solve the entire problem for you 1. Method name: clearArray Parameter(s): An int array. Return value: Nothing. The method return type is void Post-Condition: The array parameter has every element in the array set to 0 Example: After calling clearArray([1, 2]), the array has its elements changed to [0,0] 2. Method name: arrayToString Parameter(s): An int array Return value: A String containing a textual representation of the parameter. The return String should start with a curly brace, then have all the numbers in the array with a comma and space between numbers, and then ending with an end curly brace. Arrays.toString or its variants should not be used in this method Post-Condition: The array parameter is not changed Example: Calling arrayToString([1, 2]) should return "[1, 2)". 3. Method name: containsDuplicate Parameter(s): A String array Return value: Returns boolean true if one element of the array is equal() to another element of the array and false otherwise Post-Condition: The array parameter is not changed Example: Calling containsDuplicate('David", "Joe") should return false. Calling containsDuplicate(l"a", "b", "a"] should return true 4. Method name: averageArrayValues Parameter(s): An int array of at least length 1 Return value: Returns a double value that is the sum of the array elements divided by the array length. The requirement of at least one value is to prevent a divide by zero possibility Post-Condition: The array parameter is not changed Example: Calling averageArrayValues([1,2]) should return 1.5 5. MethodName: frequencyCount Parameter(s): An int array. The elements of the array can only be numbers from 0 to 9 Return value: A new int array of 10 integers. The value in the new array at index n is the number of times value n appears in the parameter array Post-Condition: The array parameter is not changed Example: Calling frequencyCount([0,0,1,1,1,7]) would return [2,3,0,0,0,0,0,1,0,0] since there are 2 zeroes and 3 ones and 1 seven, and no other digits in the parameter array 6. Method name: reverseSound Parameter(s): A double array Return value: Returns a double array that has its elements in reversed order from the parameter array Post-Condition: The array parameter is not changed. Example: Calling reverseSound(.1, 02, 03, 0.5]) should return a new array [O.5. O.3, 0.2. O.1] 7. Method name: scaleSound Parameter(s): A double array and a double value Return value: Returns a double array that has as its elements each of the parameter array elements scaled by the second parameter. Post-Condition: The array parameter is not changed. Example: Caling scaleSound ([O.0, -0.1, 0.3], 2.0) should return a new array [0.0,-0.2,0.6] Note: The play method for sounds keeps all values in the required -1.0 to 1.0 range, you do not need to test for this like you did in the Picture brightening 8. Method name: echoSound Parameter(s): A double array, an int specifying how many samples offset the echo starts at, and a double giving a weight to the echo. The double array will have at least as many values as the offset. Return value: A double array as long as the array parameter plus the offset parameter. The first offset samples will be the same as the first offset samples as the parameter array. The last offset samples will be the same as the last offset samples from the parameter array scaled by the weight parameter. The middle samples will be the sample from the parameter array plus the sample from the parameter array back the offset amount scaled by the weight parameter. Here is the logic of it. An echo is a diminished sound delayed from bouncing off a distance object. So if you yell, I first hear your original signal. Then when the bounce comes back, I hear your original signal plus a scaled down signal from back in time. Then, when you stop, I only hear the scaled down signal from back in time. Post-Condition: The array parameter is not changed. Example: Calling echoSound([O.1, 0.2, 0.3, 0.4], 1, 0.5) should return a new array of length 5 (the original array plus the offset). The new array is [0.1, 0.25, 0.4, 0.55, 0.2]. The 0.1 is before the echo starts and is unchanged. The 0.25 comes from 0.2(0.1 0.5). The 0.4 comes from 0.3+(0.2 0.5). The 0.55 comes from 0.4 (0.3 0.5). The last value is all echo, and is 0.4* 0.5 9. Method name: smoothSound Parameter(s): A double array with at least 3 elements in it. Return value: Returns a double array that has as its elements a sliding average of the parameter array elements. More precisely: 1. The first and last elements of the new array are the same as the first and last elements of the parameter array, respectively 2. For the other elements of the new array, for an element at index i, it is equal to the average of elements from the parameter array at indices i-1,i, and i+1 Post-Condition: The array parameter is not changed Example: Calling smoothSound([0.0, 0.2, 0.7, 0.2]) should return a new array [0.0, 0.3, 0.3666..,.0.2]. When played, noise in the sound should be reduced If you want to hear the effect of these sound methods, you can add code in main like this double] samples StdAudio.readC"asyouwish2.wav); StdAudio.play (samples); double[] reversed -reverseSound(samples) StdAudio.play(reversed); Note that this isn't really a test - it is to help you appreciate the kinds of effects we can generate pretty easily in code. A test has an expected output for a given input to a method Submitting Submit your ArraysAndSound.java to gradescope. You will see some tests to help you understand if you are meeting the specification. Issues with StdAudio? A couple of people have had some issues using the "StdAudio.java" file The main issue seems to be eclipse complaining about importing javax.sound. Basically eclipse has underlined a bunch of lines of code in red and for some people running the main method produces an error import javaM.sound.sampled..CLR; If you are having issues, the fix below seems to be working OK: In the main menu bar navigate to project> properties > Java Build Path. Double click JRE System Library to open up a new window. Change the execution from CDC-1.0 to JavaSE-11 which is located at the bottom
Step by Step Solution
There are 3 Steps involved in it
Step: 1
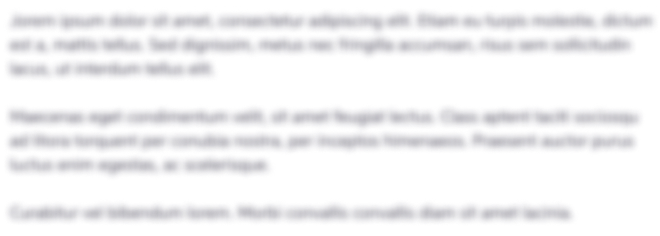
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started