Question
package Apps; import java.util.Comparator; /** * * @author ITSC 2214 Q */ public class Vote implements Comparable { String name; String state; int ID; String
package Apps; import java.util.Comparator; /** * * @author ITSC 2214 Q */ public class Vote implements Comparable { String name; String state; int ID; String whom2vote; public Vote(String name, String state, int ID, String whom2vote) { this.name = name; this.state = state; this.ID = ID; this.whom2vote = whom2vote; } @Override public String toString() { return name + ":" + state + ":" + ID + ":" + whom2vote; } /** * TODO compare two vote objects based on voting registration ID * You could use Comparators.ID.compare method which needs two * arguments. * @param o * @return */ @Override public int compareTo(Vote o) { //TODO compares two vote objects based on voting registration //ID. Please use Comparators.ID.compare method which needs //two arguments } public static class Comparators { /** * sort by state Votes will be sorted by states, ignoring * cases. If two votes came from the same state, these two * votes should be sorted based on vote registration ID. */ public static Comparator STATE = new Comparator() { @Override public int compare(Vote o1, Vote o2) { int i = o1.state.compareToIgnoreCase(o2.state); if (i == 0) { return o1.ID - o2.ID; } return i; } }; /** * sort by state Votes will be sorted by vote registration ID. */ public static Comparator ID = new Comparator() { @Override public int compare(Vote o1, Vote o2) { return o1.ID - o2.ID; } }; /** * TODO Sort by whom to vote. Votes will be sorted by the information of whom to * vote, ignoring cases. If two votes would like to vote the * same person, these two votes should be sorted based on * voting states; if two votes came from the same state, these * two votes should be sorted based on vote registration ID. */ public static Comparator WHOM2VOTE = new Comparator() { @Override public int compare(Vote o1, Vote o2) { //TODO //compare the values of whom2vote from o1 and o2 //if the value difference is not zero retur value difference //or else compare the values of state from o1 and o2 //if the value difference is not zero retur value difference //or else return the vote registration id difference } }; } }
1. int compare(Vote o1, Vote o2)
Fill missing statements in the anonymous class defined in the who2vote Comparator object statement. The comparator object is to help compare two votes by whom to vote.
Reminder:Votes will be sorted by the information of whom to vote (not case-sensitive). If two votes are for the same candidate, these two votes should be sorted based on the voting state; if two votes came from the same state, these two votes should be sorted based on vote registration ID.
To view a completed example refer to this method implementation: public static Comparator STATE = new Comparator() {...}
Note: Write in plain words how this method works. Feel free to use pseudocode in addition to or throughout your explanation. Explanations should not be entirely pseudocode, but will likely be a mix of both everyday language and coding syntax.
2. int compareTo(Vote o);
Fill missing statements in the compareTo method, which compares two vote objects based on voting registration ID. Please use Comparators.ID.compare method which needs two arguments.
Note: Write in your submission sheet how this method works.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Here are the missing statements for both parts compare method in the WHO2VOTE comparator public stat...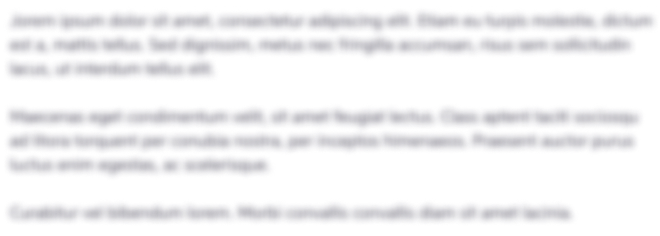
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started