Question
package edu.ser222.m02_02; /** * Implements various divide and conquer algorithms. * * Last updated 4/2/2022. * * Completion time: (your completion time) * * @author
package edu.ser222.m02_02;
/**
* Implements various divide and conquer algorithms.
*
* Last updated 4/2/2022.
*
* Completion time: (your completion time)
*
* @author (your name), Acuna, Sedgewick and Wayne
* @verison (version)
*/
import java.util.Random;
public class CompletedMerging implements MergingAlgorithms {
//TODO: implement interface methods.
@Override
public
//TODO: implement this!
return null;
}
@Override
public void sort(Comparable[] a) {
//TODO: implement this!
}
@Override
public Comparable[] mergesort(Comparable[] a) {
//TODO: implement this!
return null;
}
@Override
public Comparable[] merge(Comparable[] a, Comparable[] b) {
//TODO: implement this!
return null;
}
@Override
public void shuffle(Object[] a) {
//TODO: implement this!
}
/**
* entry point for sample output.
*
* @param args the command line arguments
*/
public static void main(String[] args) {
Queue
Queue
Queue
Queue
MergingAlgorithms ma = new CompletedMerging();
//Q1 - sample test cases
Queue merged1 = ma.mergeQueues(q1, q2);
System.out.println(merged1.toString());
Queue merged2 = ma.mergeQueues(q3, q4);
System.out.println(merged2.toString());
//Q2 - sample test cases
String[] a = {"S", "O", "R", "T", "E", "X", "A", "M", "P", "L", "E"};
ma.sort(a);
assert isSorted(a);
show(a);
//Q3 - sample test cases
String[] b = {"S", "O", "R", "T", "E", "X", "A", "M", "P", "L", "E"};
ma.shuffle(b);
show(b);
ma.shuffle(b);
show(b);
}
//below are utilities functions, please do not change them.
//sorting helper from text
private static boolean less(Comparable v, Comparable w) {
return v.compareTo(w) < 0;
}
//sorting helper from text
private static void show(Comparable[] a) {
for (Comparable a1 : a)
System.out.print(a1 + " ");
System.out.println();
}
//sorting helper from text
public static boolean isSorted(Comparable[] a) {
for (int i = 1; i < a.length; i++)
if (less(a[i], a[i-1]))
return false;
return true;
}
}
Please implement anything that says TODO
Step by Step Solution
There are 3 Steps involved in it
Step: 1
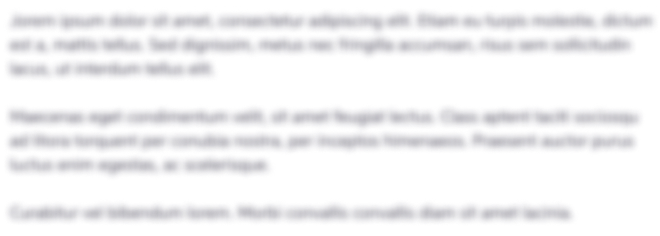
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started