Answered step by step
Verified Expert Solution
Question
1 Approved Answer
package grade _ book _ 8 ; / / Fig. 7 . 1 8 : GradeBook.java / / Grade book using two - dimensional array
package gradebook;
Fig. : GradeBook.java
Grade book using twodimensional array to store grades.
public class GradeBook
private String courseName; name of course this grade book represents
private int grades; twodimensional array of student grades
twoargument constructor initializes courseName and grades array
public GradeBook String name, int gradesArray
courseName name; initialize courseName
grades gradesArray; store grades
end twoargument GradeBook constructor
method to set the course name
public void setCourseName String name
courseName name; store the course name
end method setCourseName
method to retrieve the course name
method to retrieve the course name
public String getCourseName
return courseName;
end method getCourseName
display a welcome message to the GradeBook user
public void displayMessage
getCourseName gets the name of the course
System.out.printf "Welcome to the grade book for
s
getCourseName;
end method displayMessage
perform various operations on the data
public void processGrades
output grades array
outputGrades;
call methods getMinimum and getMaximum
System.out.printf
s d
s d
"Lowest grade in the grade book is getMinimum
"Highest grade in the grade book is getMaximum;
output grade distribution chart of all grades on all tests
outputBarChart;
end method processGrades
find minimum grade
public int getMinimum
assume first element of grades array is smallest
int lowGrade grades;
loop through rows of grades array
for int studentGrades : grades
loop through columns of current row
for int grade : studentGrades
if grade less than lowGrade, assign it to lowGrade
if grade lowGrade
lowGrade grade;
end inner for
end outer for
return lowGrade; return lowest grade
end method getMinimum
find maximum grade
public int getMaximum
assume first element of grades array is largest
int highGrade grades;
loop through rows of grades array
for int studentGrades : grades
loop through columns of current row
for int grade : studentGrades
if grade greater than highGrade, assign it to highGrade
if grade highGrade
highGrade grade;
end inner for
end outer for
return highGrade; return highest grade
end method getMaximum
determine average grade for particular student or set of grades
public double getAverage int setOfGrades
int total ; initialize total
sum grades for one student
for int grade : setOfGrades
total grade;
return average of grades
return double total setOfGrades.length;
end method getAverage
output bar chart displaying overall grade distribution
public void outputBarChart
System.out.println "Overall grade distribution:" ;
stores frequency of grades in each range of grades
int frequency new int;
for each grade in GradeBook, increment the appropriate frequency
for int studentGrades : grades
for int grade : studentGrades
frequency grade ;
end outer for
for each grade frequency, print bar in chart
for int count ; count frequency.length; count
output bar label : : :
if count
System.out.printfd: ;
else
System.out.printfdd:
count count ;
print bar of asterisks
for int stars ; stars frequency count ; stars
System.out.print;
System.out.println; start a new line of output
end outer for
end method outputBarChart
output the contents of the grades array
public void outputGrades
System.out.println "The grades are:
;
System.out.print; align column heads
create a column heading for each of the tests
for int test ; test gradeslength; test
System.out.printf "Test d test ;
System.out.println "Average" ; student average column heading
create rowscolumns of text representing array grades
for int student ; student grades.length; student
System.out.printf "Student d student ;
for int test : grades student output student's grades
System.out.printfd test ;
call method getAverage to calculate student's average grade;
pass row of grades as the argument to getAverage
double average getAverage grades student ;
System.out.printff
average ;
end outer for
end method outputGrades
end class GradeBook
package gradebook;
Fig. : GradeBookTest.java
Creates GradeBook object using a two
Step by Step Solution
There are 3 Steps involved in it
Step: 1
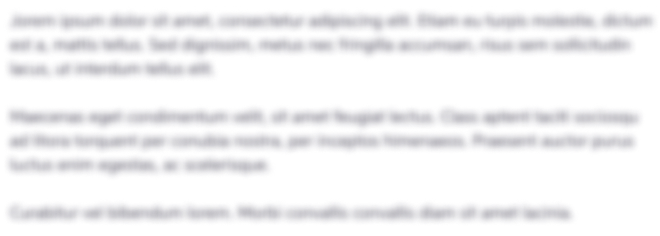
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started