Question
package Lab11; public class BinaryTreeNode { BinaryTreeNode left, right; int data; public BinaryTreeNode(){ left=null; right=null; data=0; } public BinaryTreeNode(int value){ left=null; right=null; data=value; } public
package Lab11;
public class BinaryTreeNode {
BinaryTreeNode left, right;
int data;
public BinaryTreeNode(){
left=null;
right=null;
data=0;
}
public BinaryTreeNode(int value){
left=null;
right=null;
data=value;
}
public void setLeft(BinaryTreeNode node){
left=node;
}
public void setRight(BinaryTreeNode node){
right=node;
}
public void setData(int value){
data=value;
}
public BinaryTreeNode getLeft(){
return left;
}
public BinaryTreeNode getRight(){
return right;
}
public int getData(){
return data;
}
}
package Lab11;
public class BinaryTree {
private BinaryTreeNode root;
public BinaryTree(){
root=null;
}
public boolean isEmpty(){
return root==null;
}
public BinaryTreeNode insert(BinaryTreeNode node, int data)
{
if(node==null){
node=new BinaryTreeNode(data);
}
else
{
if(node.getLeft()==null)
node.left=insert(node.left,data);
else
node.right=insert(node.right,data);
}
return node;
}
public void insert(int data){
root=insert(root, data);
}
private int countNodes(BinaryTreeNode r){
if(r==null)
return 0;
else
{
int number=1;
number+=countNodes(r.getLeft());
number+=countNodes(r.getRight());
return number;
}
}
public int countNodes(){
return countNodes(root);
}
public boolean search(BinaryTreeNode r, int data){
if(r.getData()==data)
return true;
if(r.getLeft()!=null)
if(search(r.getLeft(),data))
return true;
if(r.getRight()!=null)
if(search(r.getRight(),data))
return true;
return false;
}
public boolean search(int val){
return search(root, val);
}
}
package Lab11;
import java.util.Scanner;
public class BinaryTreeTest {
public static void main(String[] args){
BinaryTree binaryTree=new BinaryTree();
/*Scanner scan =new Scanner(System.in);
System.out.println("Enter 10 integers to insert");
*/
int[] a={50, 76,21, 4, 32, 64, 15, 52, 14, 100, 83, 2, 3, 70, 87,80};
for(int i=0;i
binaryTree.insert(a[i]);
}
/* Display tree */
System.out.print(" Postorder : ");
binaryTree.postorder();
System.out.print(" Preorder : ");
binaryTree.preorder();
System.out.print(" Inorder : ");
binaryTree.inorder();
/* test search method */
int searchKey=50;
System.out.println("Search result : "+ binaryTree.search(searchKey));
/* display the node number in a tree */
System.out.println("Nodes = "+ binaryTree.countNodes());
/* test the tree is empty or not*/
System.out.println("Empty status = "+ binaryTree.isEmpty());}
}
whole Java code,thanks!
Data Structures. 1. Task: Implement preorder, inordex, postorder methods. Put them in the class of BinaryTree and then test their correctness in the class BinarvTreeTest class. 2. Procedure: download the attached files and fulfil the task, run your codes. * 3. Submission: Submit your code via word file along with the snapshots of your resultsStep by Step Solution
There are 3 Steps involved in it
Step: 1
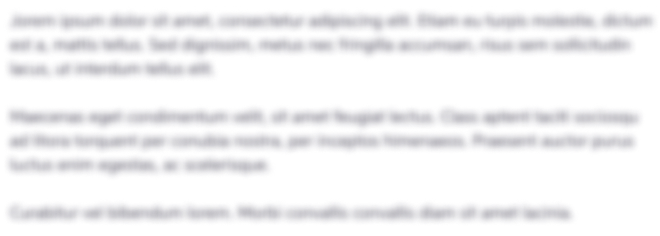
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started