Question
package murach.app; import murach.business.*; import murach.database.ProductDB; import murach.presentation.Console; public class LineItemApp { public static void main(String args[]) { // display a welcome message System.out.println(Welcome to
package murach.app;
import murach.business.*; import murach.database.ProductDB; import murach.presentation.Console;
public class LineItemApp {
public static void main(String args[]) { // display a welcome message System.out.println("Welcome to the Line Item Calculator"); System.out.println();
// perform 1 or more calculations String choice = "y"; while (choice.equalsIgnoreCase("y")) { // get the input from the user String productCode = Console.getString("Enter product code: "); int quantity = Console.getInt("Enter quantity: ", 0, 1000);
// use the ProductDB class to get the Product object Product product = ProductDB.getProduct(productCode);
// create the LineItem object LineItem lineItem = new LineItem(); lineItem.setProduct(product); lineItem.setQuantity(quantity);
// display the output System.out.println(); System.out.println("LINE ITEM"); System.out.println("Code: " + product.getCode()); System.out.println("Description: " + product.getDescription()); System.out.println("Price: " + product.getPriceFormatted()); System.out.println("Quantity: " + lineItem.getQuantity()); System.out.println("Total: " + lineItem.getTotalFormatted() + " ");
// see if the user wants to continue choice = Console.getString("Continue? (y): "); System.out.println(); } } }
space
space
package murach.business;
import java.text.NumberFormat;
/** * The LineItem
class represents a line item and is used by the * Product
class. */ public class LineItem {
private Product product; private int quantity; private double total;
public LineItem() { this.product = null; this.quantity = 0; this.total = 0; }
public LineItem(Product product, int quantity) { this.product = product; this.quantity = quantity; }
public void setProduct(Product product) { this.product = product; }
public Product getProduct() { return product; }
public int getQuantity() { return quantity; }
public void setQuantity(int quantity) { this.quantity = quantity; }
public double getTotal() { total = quantity * product.getPrice(); return total; }
public String getTotalFormatted() { NumberFormat currency = NumberFormat.getCurrencyInstance(); return currency.format(this.getTotal()); } }
space
space
package murach.business;
import java.text.NumberFormat;
/** * The Product
class represents a product and is used by the * LineItem
class. * @author Joel Murach * @version 1.0.0 */ public class Product {
private String code; private String description; private double price;
/** * Creates a Product
with default values. */ public Product() { code = ""; description = ""; price = 0; }
/** * Sets the product's code. * @param code a String
for the product's code */ public void setCode(String code) { this.code = code; }
/** * Gets the product's code. * @return a String
for the product's code */ public String getCode() { return code; }
/** * Sets the product's description. * @param description a String
for the product's description */ public void setDescription(String description) { this.description = description; }
/** * Gets the product's description. * @return a String
for the product's description */ public String getDescription() { return description; }
/** * Sets the product's price. * @param price a double
value for the product's price */ public void setPrice(double price) { this.price = price; }
/** * Gets a double
value for the product's price. * @return a double
value that represents the product's price */ public double getPrice() { return price; }
/** * Gets a String
for the product's price with * standard currency formatting * @return a String
for the product's price with * standard currency formatting applied ($1,000.00). */ public String getPriceFormatted() { NumberFormat currency = NumberFormat.getCurrencyInstance(); return currency.format(price); } }
space
space
package murach.database;
import murach.business.*;
public class ProductDB {
public static Product getProduct(String productCode) { // In a more realistic application, this code would // get the data for the product from a file or database // For now, this code just uses if/else statements // to return the correct product
// create the Product object Product product = new Product();
// fill the Product object with data product.setCode(productCode); if (productCode.equalsIgnoreCase("java")) { product.setDescription("Murach's Java Programming"); product.setPrice(57.50); } else if (productCode.equalsIgnoreCase("jsp")) { product.setDescription("Murach's Java Servlets and JSP"); product.setPrice(57.50); } else if (productCode.equalsIgnoreCase("mysql")) { product.setDescription("Murach's MySQL"); product.setPrice(54.50); } else { product.setDescription("Unknown"); } return product; } }
space
space
package murach.presentation;
import java.util.Scanner;
public class Console {
private static Scanner sc = new Scanner(System.in); public static String getString(String prompt) { String s = ""; boolean isValid = false; while (!isValid) { System.out.print(prompt); if (sc.hasNext()) { s = sc.nextLine(); // read entire line isValid = true; } else { System.out.println("Error! Invalid string value. Try again."); } } return s; }
public static double getDouble(String prompt) { double d = 0; boolean isValid = false; while (!isValid) { System.out.print(prompt); if (sc.hasNextDouble()) { d = sc.nextDouble(); isValid = true; } else { sc.next(); // discard the incorrectly entered double System.out.println("Error! Invalid decimal value. Try again."); } sc.nextLine(); // discard any other data entered on the line } return d; }
public static double getDouble(String prompt, double min, double max) { double d = 0; boolean isValid = false; while (!isValid) { d = Console.getDouble(prompt); if (d = max) { System.out.println( "Error! Number must be less than " + max + "."); } else { isValid = true; } } return d; }
public static int getInt(String prompt) { boolean isValid = false; int i = 0; while (!isValid) { System.out.print(prompt); if (sc.hasNextInt()) { i = sc.nextInt(); isValid = true; } else { sc.next(); // discard invalid data System.out.println("Error! Invalid integer value. Try again."); } sc.nextLine(); // discard any other data entered on the line } return i; }
public static int getInt(String prompt, int min, int max) { int i = 0; boolean isValid = false; while (!isValid) { i = Console.getInt(prompt); if (i = max) { System.out.println( "Error! Number must be less than " + max + "."); } else { isValid = true; } } return i; } }
Work with documentation 2. Exercise 10-2 This exercise guides you through the process of using NetBeans to add javadoc comments to the Console class and to generate the API documentation for all the murach packages. 1. Open the project named ch10_ex2_LineItem that's stored in the ex_starts directory Open the Product class that's in the murach.business package. Then, view the javadoc comments that have been added to this class. Note that these comments don't include the @param or @return tags. 3. Open the LineItem class that's in the murach.business package. Note that a single javadoc comment has been added at the beginning of this class. 4. Open the Console class that's in the murach.presentation package. Then, add javadoc comments to this class and each of its methods. Make sure to include @param and @return tags for all of its methods. 5. Generate the documentation for the project. This should automatically open the documentation in a web browser. 6. View the documention for the LineItem class so you can see the documentation that's generated for a class by default. 7. View the documentation for the Product class. Note that the details for the methods don't include a description of the parameters or return values. 8. View the documentation for the Console class. Note that the details for the methods include the descriptions of the parameters and return values. Then, close your browser. 9. Navigate to the distljavadoc directory for the project and view the files for this directory. Then, open the index.html page in your browser. Note that it displays the documentation for the project. Work with documentation 2. Exercise 10-2 This exercise guides you through the process of using NetBeans to add javadoc comments to the Console class and to generate the API documentation for all the murach packages. 1. Open the project named ch10_ex2_LineItem that's stored in the ex_starts directory Open the Product class that's in the murach.business package. Then, view the javadoc comments that have been added to this class. Note that these comments don't include the @param or @return tags. 3. Open the LineItem class that's in the murach.business package. Note that a single javadoc comment has been added at the beginning of this class. 4. Open the Console class that's in the murach.presentation package. Then, add javadoc comments to this class and each of its methods. Make sure to include @param and @return tags for all of its methods. 5. Generate the documentation for the project. This should automatically open the documentation in a web browser. 6. View the documention for the LineItem class so you can see the documentation that's generated for a class by default. 7. View the documentation for the Product class. Note that the details for the methods don't include a description of the parameters or return values. 8. View the documentation for the Console class. Note that the details for the methods include the descriptions of the parameters and return values. Then, close your browser. 9. Navigate to the distljavadoc directory for the project and view the files for this directory. Then, open the index.html page in your browser. Note that it displays the documentation for the projectStep by Step Solution
There are 3 Steps involved in it
Step: 1
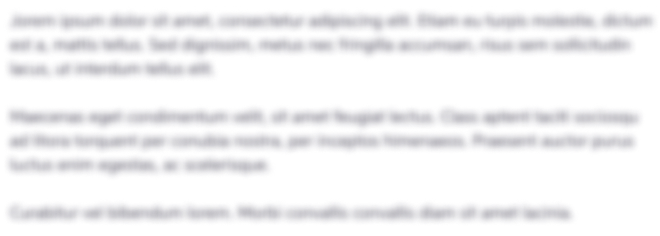
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started