Question
package newSet; public class newSet { private String[] set; private int size; public newSet(int N) { this.set = new String[N]; this.size = 0; } public
package newSet;
public class newSet { private String[] set; private int size;
public newSet(int N) { this.set = new String[N]; this.size = 0; }
public void setAdd(String new_val) { if (this.size == this.set.length) return ; else if (contains(new_val) == 0) { this.set[size] = new_val; (this.size)++; } }
public int contains(String val) { for (int i = 0; i
return 0; }
public void SetPrint() { for (int i = 0; i
public newSet SetsInter(newSet set1) { int N1 = this.size; int N2 = set1.size;
int N = Math.min(N1, N2);
newSet set_IN = new newSet(N);
for (int i = 0; i
if (set1.contains(checking) == 1) { set_IN.setAdd(checking); } }
return set_IN; }
public int subset(newSet set1) { // To be implemented return 0; }
public int isEmpty() { // To be implemented return 0; }
public int equals(newSet set1) { // To be implemented return 0; }
public newSet SetsUnion(newSet set1) { // To be implemented return null; }
public newSet SetsDiff(newSet set1) { // To be implemented return null; }
public newSet SetRemove(String val) { // To be implemented return null; }
}
package newSet; public class testSet { public static void main(String[] args) { // code from lectures newSet set0 = new newSet(10); set0.setAdd("Hello"); set0.setAdd("World"); set0.setAdd("Hello"); set0.setAdd("hi"); System.out.print("set0 = "); set0.SetPrint(); newSet set1 = new newSet(10); set1.setAdd("Hello"); set1.setAdd("World"); set1.setAdd("DREW"); set1.setAdd("hi"); System.out.print("set1 = "); set1.SetPrint(); newSet set_IN = set0.SetsInter(set1); System.out.print("Intersection: "); set_IN.SetPrint(); // new code below // checking if empty // test1 System.out.print("set0 is empty: "); int empty = set0.isEmpty(); if (empty == 1) System.out.print("true"); else System.out.print("false"); System.out.println(); // test2 System.out.print("set2 is empty: "); newSet set2 = new newSet(5); empty = set2.isEmpty(); if (empty == 1) System.out.print("true"); else System.out.print("false"); System.out.println(); // checking subset int flag = set1.subset(set0); System.out.print("set0 is subset of set1: "); if (flag == 1) System.out.print("true"); else System.out.print("false"); System.out.println(); // checking for equal System.out.print("set0 = set1: "); int same = set0.equals(set1); if (same == 1) System.out.print("true"); else System.out.print("false"); System.out.println(); // checking for removal and equal newSet set3 = set1.SetRemove("DREW"); System.out.print("set0 = set3: "); same = set0.equals(set3); if (same == 1) System.out.print("true"); else System.out.print("false"); System.out.println(); // checkig for union newSet set_UN = set0.SetsUnion(set1); System.out.print("Union: "); set_UN.SetPrint(); // checkig for difference newSet set_DIFF = set0.SetsDiff(set1); System.out.print("Difference: "); set_UN.SetPrint(); } }
Java Data Structure question.
Please help with the blank in the class newSet and make sure it can run. Thanks!
Please read before you proceed: - Given the newSet class provided below, finish the implementation of the following methods. - To test your implementation, run the provided testSet.java class. You can also add your own test code. - Per all the programming assignments from any course, a sufficient amount of comment is required in order for you to get the full credits. Methods to implement: 1. (2 points) public int isEmpty() or public boolean isEmpty(): the method that checks whether the caller set is empty or not. Depending on your return type, if empty then return 1 or true; otherwise, return 0 or false. 2. (3 points) public int subset(newSet set1) or public boolean subset(newSet set1): the method that checks whether set1 is the subset of this. If set1 is indeed the subset of this, then return 1 or true; otherwise, return 0 or false. 3. (3 points) public int equals(newSet set1) or public boolean equals(newSet set1): the method that checks whether this and set1 are the same set. If set1 is indeed the same as this, then return 1 or true; otherwise, return 0 or false. Recall how sets equal can be defined with sets subset. 4. (4 points) public newSet SetsUnion(newSet set1): the method that creates a new set that is the union of this and set1. 5. (4 points) public newSet SetsDiff(newSet set1): the method that creates a new set that is the set difference of this with set1. More specifically, subtracting set1 from this (i.e., this - set1). 6. (4 points) public newSet SetRemove(String val): the method that creates a new set that is this having element val removed, if val is in this; otherwise, does nothingStep by Step Solution
There are 3 Steps involved in it
Step: 1
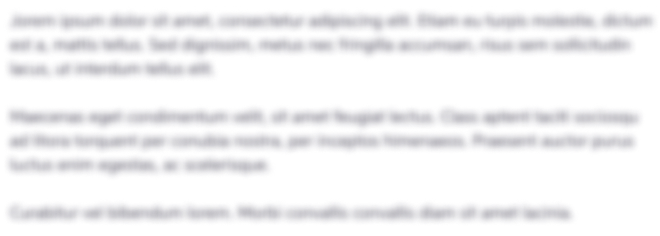
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started