Question
package Shopping; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; /** * * */ public class ShoppingListArrayList implements ShoppingListADT { private ArrayList shoppingList; /** * Default constructor
package Shopping;
import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner;
/** * * */ public class ShoppingListArrayList implements ShoppingListADT {
private ArrayList
/** * Default constructor of ShoppingArray object. */ public ShoppingListArrayList() { this.shoppingList = new ArrayList<>(); }
/** * Constructor of ShoppingArray object that parses from a file. * * @param filename the name of the file to parse * @throws FileNotFoundException if an error occurs when parsing the file */ public ShoppingListArrayList(String filename) throws FileNotFoundException { this.shoppingList = new ArrayList<>(); scanFile(filename); }
/** * Method to add a new entry. Only new entries can be added. Combines * quantities if entry already exists. * * @param entry the entry to be added */ @Override public void add(Grocery entry) { if (entry == null) { return; } // Check if this item already exists if (this.contains(entry)) { //Merge the quantity of new entry into existing entry combineQuantity(entry); return; }
shoppingList.add(entry); }
/** * Method to remove an entry. * * @param ent to be removed * @return true when entry was removed * @throws DataStructures.ElementNotFoundException */ @Override public boolean remove(Grocery ent) { // the boolean found describes whether or not we find the // entry to remove boolean found = false;
// TODO search in the shoppingList, if find ent in the // list remove it, set the value of `found' // It is okay to directly use methods from the Java Build-in // ArrayList class: It removes the first occurrence of the specified // element from this list, if it is present. If the list does // not contain the element, it is unchanged. It returns true // if this list contained the specified element found = this.shoppingList.remove(ent); // Return false if not found return found; }
/** * Method to find an entry at the given index of the array list . * * @param index to find * @return the entry if found * @throws Exceptions.EmptyCollectionException */ @Override public Grocery find(int index) throws IndexOutOfBoundsException, EmptyCollectionException { if (this.isEmpty()) { throw new EmptyCollectionException("ECE - find"); } // TODO check whether or not the input index number is legal // for example, < 0 or falls outside of the size. // If it is not legal, throw an IndexOutOfBoundsException if (index < 0 || index > this.shoppingList.size()) { throw new IndexOutOfBoundsException("ArrayList - find"); } // return the corresponding entry in the shoppingList // need to change the return value // return null; return (Grocery)this.shoppingList.get(index); }
/** * Method to locate the index of an entry. * * @param ent to find the index * @return the index of the entry * @throws ElementNotFoundException if no entry was found */ @Override public int indexOf(Grocery ent) throws ElementNotFoundException { if (ent != null) { for (int i = 0; i < shoppingList.size(); i++) { if (shoppingList.get(i).compareTo(ent) == 0) { return i; } } } throw new ElementNotFoundException("indexOf"); }
/** * Method to determine whether the object contains an entry. * * @param ent to find * @return true if and only if the entry is found */ @Override public boolean contains(Grocery ent) { boolean hasItem = false; if (ent != null) { // TODO go through the shoppingList and try to find the // item in the list. If found, return true. //hasItem = this.shoppingList.contains(ent); for (int i = 0; i < shoppingList.size(); i++) { if (shoppingList.get(i).compareTo(ent) == 0) { return true; } } } return hasItem; }
/** * Gets the size of the collection. * * @return the size of the collection */ @Override public int size() { return shoppingList.size(); }
/** * Gets whether the collection is empty. * * @return true if and only if the collection is empty */ @Override public boolean isEmpty() { return shoppingList.isEmpty(); }
/** * Returns a string representing this object. * * @return a string representation of this object */ @Override public String toString() { StringBuilder s = new StringBuilder(); s.append(String.format("%-25s", "NAME")); s.append(String.format("%-18s", "CATEGORY")); s.append(String.format("%-10s", "AISLE")); s.append(String.format("%-10s", "QUANTITY")); s.append(String.format("%-10s", "PRICE")); s.append(' '); s.append("------------------------------------------------------------" + "-------------"); s.append(' '); for (int i = 0; i < shoppingList.size(); i++) { s.append(String.format("%-25s", shoppingList.get(i).getName())); s.append(String.format("%-18s", shoppingList.get(i).getCategory())); s.append(String.format("%-10s", shoppingList.get(i).getAisle())); s.append(String.format("%-10s", shoppingList.get(i).getQuantity())); s.append(String.format("%-10s", shoppingList.get(i).getPrice())); s.append(' '); s.append("--------------------------------------------------------" + "-----------------"); s.append(' '); }
return s.toString(); }
/** * Add the quantity of a duplicate entry into the existing * * @param entry duplicate */ private void combineQuantity(Grocery entry) { try { int index = this.indexOf(entry); shoppingList.get(index).setQuantity( shoppingList.get(index).getQuantity() + entry.getQuantity()); } catch (ElementNotFoundException e) { System.out.println("combineQuantity - ECE"); }
}
/** * Scans the specified file to add items to the collection. * * @param filename the name of the file to scan * @throws FileNotFoundException if the file is not found */ private void scanFile(String filename) throws FileNotFoundException { Scanner scanner = new Scanner(getClass().getResourceAsStream(filename)) .useDelimiter("(,| )");
while (scanner.hasNext()) { Grocery temp = new Grocery(scanner.next(), scanner.next(), Integer.parseInt(scanner.next()), Float.parseFloat(scanner.next()), Integer.parseInt(scanner.next())); add(temp); } }
} Please do the TODOs correctly, please. Thank you.
package Shopping;
import DataStructures.*;
/** * @version Spring 2019 * @author Paul Franklin, Kyle Kiefer */ public interface ShoppingListADT { /** * Method to add a new entry. Only new entries can be added. Combines * quantities if entry already exists. * * @param entry the entry to be added */ public void add(Grocery entry); /** * Method to remove an entry. * * @param ent to be removed * @return true when entry was removed */ public boolean remove(Grocery ent); /** * Method to find an entry. * * @param index to find * @return the entry if found * @throws Exceptions.EmptyCollectionException */ public Grocery find(int index) throws IndexOutOfBoundsException, EmptyCollectionException; /** * Method to locate the index of an entry. * * @param ent to find the index * @return the index of the entry * @throws ElementNotFoundException if no entry was found */ public int indexOf(Grocery ent) throws ElementNotFoundException; /** * Method to determine whether the object contains an entry. * * @param ent to find * @return true if and only if the entry is found */ public boolean contains(Grocery ent); /** * Gets the size of the collection. * * @return the size of the collection */ public int size(); /** * Gets whether the collection is empty. * * @return true if and only if the collection is empty */ public boolean isEmpty(); /** * Returns a string representing this object. * * @return a string representation of this object */ @Override public String toString(); } /////////////////////////////////////
package Shopping;
/** * @version Spring 2019 * @author Paul Franklin, Kyle Kiefer */ public class Grocery implements Comparable
private String name; private String category; private int aisle; private float price; private int quantity;
/** * Constructor of Entry object. Objects that match name and * category are considered identical * * @param name the name of the item in the entry * @param category the category of the item in the entry */ public Grocery(String name, String category) { setName(name); setCategory(category); setAisle(-1); setPrice(0.0f); setQuantity(0);
}
/** * Constructor of Entry object. * * @param name the name of the item in the entry * @param category the category of the item in the entry * @param aisle the aisle where the item in the entry is located * @param price the price of the item in the entry * @param quantity the quantity of the item to purchase */ public Grocery(String name, String category, int aisle, float price, int quantity) { this(name, category); setAisle(aisle); setPrice(price); setQuantity(quantity); } /** * Copy constructor * * @param another An existing grocery object to copy */ public Grocery(Grocery another) { setName(another.getName()); setCategory(another.getCategory()); setAisle(another.getAisle()); setPrice(another.getPrice()); setQuantity(another.getQuantity()); } /** * Gets the name of the item. * * @return the name of the item */ public String getName() { return name; }
/** * Sets the name of the item. * * @param name the name of the item */ public void setName(String name) { this.name = name; }
/** * Gets the category of the item. * * @return the category of the item */ public String getCategory() { return category; }
/** * Sets the category of the item. * * @param category the category of the item */ public void setCategory(String category) { this.category = category; }
/** * Gets the aisle of the item. * * @return the aisle of the item */ public int getAisle() { return aisle; }
/** * Sets the aisle of the item. * * @param aisle the aisle of the item */ public void setAisle(int aisle) { this.aisle = aisle; }
/** * Gets the price of the item. * * @return the price of the item */ public float getPrice() { return price; }
/** * Sets the price of the item. * * @param price the price of the item */ public void setPrice(float price) { this.price = price; }
/** * Gets the quantity of the item. * * @return the quantity of the item */ public int getQuantity() { return quantity; }
/** * Sets the quantity of the item. * * @param quantity the quantity of the item */ public void setQuantity(int quantity) { this.quantity = quantity; }
/** * Returns a string representing this object. * * @return a string representation of this object */ @Override public String toString() { return "Entry{" + "name=" + name + ", category=" + category + ", aisle=" + aisle + ", price=" + price + ", quantity=" + quantity + '}'; }
/** * Compare the object itself with the input parameter object * Returns zero if both are logically equal * Returns 1 if the object itself is logically larger than * input parameter object * Returns -1 if the object itself is logically smaller than * input parameter object * @param Grocery t * An object to compare * @return an int 0, 1, or -1 */ @Override public int compareTo(Grocery t) { if (this.getName().compareToIgnoreCase(t.getName()) == 0) { return this.getCategory(). compareToIgnoreCase(t.getCategory()); } return this.getName().compareToIgnoreCase(t.getName()); }
/** * Evaluate whether the object itself is logically equal to * the input parameter object * Returns true if the object itself is logically equal to the * input parameter object. Or else return false * @param Object o * An object to compare * @return a boolean value: true or false */ @Override public boolean equals(Object o) { // If the object is compared with itself then return true if (o == this) { return true; } /* Check if o is an instance of Complex or not "null instanceof [type]" also returns false */ if (!(o instanceof Grocery)) { return false; } Grocery temp = (Grocery) o; return this.compareTo(temp) == 0; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
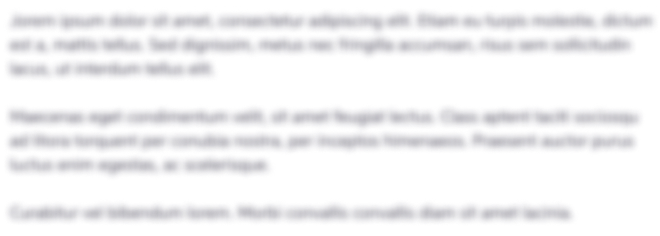
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started