Answered step by step
Verified Expert Solution
Question
1 Approved Answer
package week_5; import java.io.File; import java.io.FileWriter; import java.io.IOException; /** * **The short version**: Write the name of the operating system that's running this code, to
package week_5; import java.io.File; import java.io.FileWriter; import java.io.IOException; /** * **The short version**: Write the name of the operating system that's running this code, to a file called `os.txt`. os.txt should be saved in the `data` directory of this project. You can get find out how to get the name of your operating system by reading http://docs.oracle.com/javase/tutorial/essential/environment/sysprop.html The /data/ directory has been created for you in the root directory of this project. The file called `placeholder.file` in the data directory may be ignored, and should not affect this assignment. The text in the file should explain why it's there. Make sure you catch and handle any exceptions. Test your program on a Windows and a Mac or Linux computer. There are Macs and PCs in the hallway outside T3050. **The long version**: All of the code so far has assumed that we are working with a file in the root directory of your project. But, a file could be anywhere on your system, so Java needs to support reading files anywhere on your computer (and even on remote computers). For example, your program want to work with many files, and want to organize them into directories. Or, you've got different types of files, (maybe images, and text data files) and you'd like to organize these files into an images directory, and a data directory. For this question, write a program that creates and stores a file in the data directory. The file should be called `os.txt` and should contain your computer's operating system name. FileWriter can take a filename as an argument. The filename can only be a file in your current directory. So this doesn't work: new FileWriter fw = new FileWriter(data/testing.txt); // Error But, FileWriter can also take a File object as an argument, and a File object can store a path to a file elsewhere on your system (or even on a remote system). Linux, Max, and Unix-based computers use / to separate path components, e.g. /Bob/Documents/homework1.doc Windows uses backslashes \ to separate path components, e.g. \Bob\Documents\homework1.doc Heres one way to create a File object on a Mac, Linux, Unix etc. system; using / as a path separator, for example, File f = new File(mydirectory/mysubdirectory/textfile.txt); Or on a PC, which uses backslash as the path separator, for example, File f = new File(mydirectory\mysubdirectory\textfile.txt); Assuming these directories exist, you can create and write `textfile.txt` file in this location. The location is relative to the root directory of your project. But, there's a problem. If you write a forward slash, or backslash, your program will work fine on one system, but crash on the other. Java is architecture-neutral and the same code is supposed to run in the same way on PC and Mac and Linux, and anything else a JVM can be installed on, like a TV, Android device, refrigerator, car, toaster... Until we got to files, all of our code will run the same on any device, and we'd like to maintain this advantage as we work with files. There's two ways to handle this. 1. Java code can get information about the system it running on - system properties - which include the file path separator for the current system, the name and version of the operating system, and various others. You can get the path separator and use it to build the file path String. 2. Or, a cleaner approach: create a File object with the File(parentDirectory, filename) constructor. For example, File f = new File("mydirectory", "myfilename"); Java will check the system properties for you, and construct the correct path for the computer. For more information, see the Java File documentation. https://docs.oracle.com/javase/8/docs/api/java/io/File.html ( Note: In general, it is possible, but **not recommended**, to use absolute path names, for example C:\program files\java\textfile.txt on Windows, or /users/admin/Documents/textfile.txt on a Unix-based system like Linux or Mac, but if you do that, your program will almost certainly not work on anyone else's computer. And, the tests will fail. ) */ public class Question_8_Write_Operating_System_Info_To_File_In_Data_Directory { public static void main(String[] args) { Question_8_Write_Operating_System_Info_To_File_In_Data_Directory q8 = new Question_8_Write_Operating_System_Info_To_File_In_Data_Directory(); q8.writeOSName(); } public void writeOSName() { // TODO write the name of the operating system running this code to a file /data/os.txt // The file has to be written in the data directory of this project // Use system properties to get the operating system name. // Remember this exact same code needs to work on a Mac and Windows computer without any modifications. // Test your code on both types of operating systems: Windows, and Mac/Linux. String OS = System.getProperty("os.name").toLowerCase(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
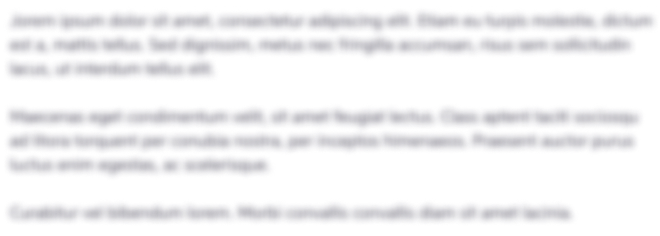
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started