Question
Parallel arrays Passing arrays to functions. You are asked to write a program that helps grading a multiple-choice test. We have two input files for
Parallel arrays Passing arrays to functions. You are asked to write a program that helps grading a multiple-choice test. We have two input files for this program: key.ext: This file contains the correct answers to a 10 multiple-choice questions. An example of this file could be as follows: A ACDBB ADCD student-answers.ext: This file contains the first names of exactly 10 students followed by their answers. An example segment of this file could be as follows: Rebecca A A CDBB ACCD Joseph A A B D B A ACCC Here are the arrays you need for this assignment: names: The string array of size 10 that will store students' names in each of its elements. correctAs: The char array of size 10 that you populate via the input file key.txt. studentAs: The char array of size 10 that will be used and reused in storing each individual student's answers listed in input file student-answers.txt. grades: This integer array of size 10 will be used to store each individual student's grade in the proper index. It will be used in parallel with the names array in the sense that the grade stored in index 0 of array grades belongs to the name stored in index 0 of array names. statArray: This array will be used to work as a tally array keeping track of number of correct answers per question on this test. As you can see there are exactly 10 questions on this test. Each cell in the statArray could be used to represent the stat regarding each question. Notice you have to find a way to map index 0 to question #1, etc. After successful tallying, the number stored in index 0 of this array will indicate the number of correct answers given for question #1, etc. The functions that you will need for this assignment: what Grade: This function receives correctAs array, studentAs array and their size, it computes their grade and returns it. The prototype of this function is: int what Grade (char(), char[], int); statcollect: This function receives correctas, studentAs, statArray array and their size, it keeps the count of the student's correct answers and update the statArray. The stat for question I goes to index 0 of the statArray. As you can see this function receives the statArray, and modifies it. We know that functions that alter the arrays they receive as parameters modify them permanently, and this is exactly what we want to achieve here. The prototype of this function is: void statcollect (char[], char[], int[], int); topStudentsName: This function receives the grades array as well as names array and their size. Looks for the highest grade, uses its index to look up and return the name associated with that grade/index. This function will be used in menu option 2. The following is its prototype: string topStudentName( int[], string[], int); Here is the to do list of major steps to take to write this program: Populate the correctAs array by reading the data in key.txt file. Set up a loop to iterate 10 times to read the contents of student-answers.txt. On each iteration: Read the first name into names array, Set up an inner loop and read the 10 char answers that follow the first name, into the array studentAs. Back to the outer loop, call the what Grade function and pass the correctAs and studentAs arrays to it. This function will return an integer representing the grade for that student, store that grade in your grades array in the proper index. Also call the statcollect function and pass correctas, studentAs, and statArray to it. Let it modify the statArray based on the count of the correct answers given by the student per question. Loop back again: read a new name, and reuse your studentAs array, and repeat the above steps until you finish reading data from the file. Now you are ready to display the following menu, and let the user use the data you have collected and processed: Look up a student's grade Please enter the first name. What is the name of the student with the highest grade? How many questions were answered correctly by ALL students? Exit Make sure you submit a program that compiles. An incomplete program that compiles could possibly eam partial points, a non-compiling program will be considered void. Attend the online lab assistance sessions to seek help when needed. Send a message to the lab assistant.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
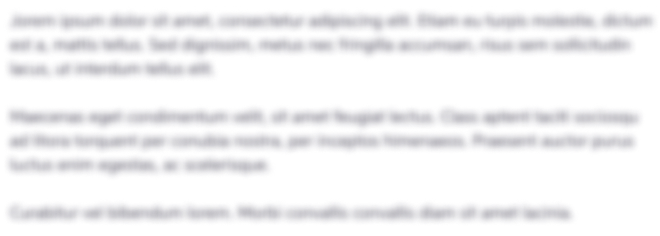
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started