Question
Parking Ticket Simulation For this programming assignment you will write a parking ticket simulation. This assignment is similar to your second lab, so do refer
Parking Ticket Simulation
For this programming assignment you will write a parking ticket simulation. This assignment is similar to your second lab, so do refer to it for hints and help. You will write several classes, and then make objects of those classes using information from text files. Read this document to the end, to get a good understanding of the entire program. Take a look at the UMLs and the relationship between classes. Take a look at the text files and the sample output.
Car.Txt File
Meter.txt file
Here's my code don't know how to finish the simulator class. but I think everything else is good to go but please check.
1 public class ParkedCar { 2 3 private String make; 4 private String model; 5 private String color; 6 private String licensePlate; 7 private int minutesParked; 8 9 public ParkedCar(String mk, String md, String col, String lic, int minParked) { 10 this.make = make; 11 this.model = model; 12 this.color = color; 13 this.licensePlate = licensePlate; 14 this.minutesParked = minutesParked; 15 } 16 17 public ParkedCar(ParkedCar car){ 18 this.make = car.make; 19 this.model = car.model; 20 this.color = car.color; 21 this.licensePlate = car.licensePlate; 22 this.minutesParked = car.minutesParked; 23 } 24 25 public String toString(){ 26 String str = "Make: " + model + " Model: " + model + " Color: " + color 27 + " License Number: " + licensePlate + " Minutes Parked" + minutesParked; 28 return str; 29 } 30 public void setMake(String mk){ 31 this.make = mk; 32 } 33 public String getMake(){ 34 return this.make; 35 } 36 37 public void setModel(String md){ 38 this.model = md; 39 } 40 public String getModel(){ 41 return this.model; 42 } 43 44 public void setColor(String col){ 45 this.color = col; 46 } 47 public String getColor(){ 48 return this.color; 49 } 50 51 public void setLincensePlate(String lic){ 52 this.licensePlate = lic; 53 } 54 public String getLicensePlate(){ 55 return this.licensePlate; 56 } 57 58 public void setMinutesParked(int minParked){ 59 this.minutesParked = minParked; 60 } 61 public int getMinutesParked(){ 62 return minutesParked; 63 } 64 65 66 } 67
1 public class ParkingMeter{ 2 3 // declaring varaible 4 private int minutesPurchased; 5 6 // creating constructor class 7 public ParkingMeter(int minPurchased){ 8 this.minutesPurchased = minutesPurchased; 9 } 10 11 public String toString(){ 12 String str = "Minutes Purchased" + minutesPurchased; 13 return str; 14 } 15 public void setMinutesPurchased(int minPurchased){ 16 this.minutesPurchased = minPurchased; 17 } 18 public int getMinutesPurchased(){ 19 return minutesPurchased; 20 } 21 }
1 public class PoliceOfficer{ 2 private String name; 3 private String badgeNumber; 4 5 public PoliceOfficer(String name, String badge){ 6 this.name = name; 7 badgeNumber = badge; 8 9 } 10 public PoliceOfficer(PoliceOfficer officer){ 11 this.name = officer.name; 12 this.badgeNumber = officer.badgeNumber; 13 } 14 15 public String toString(){ 16 String str = "Officer Data: " + " Name: " + name + " Badge Number: " + badgeNumber; 17 return str; 18 } 19 public void setName(String name){ 20 this.name = name; 21 } 22 public String getName(){ 23 return this.name; 24 } 25 public void setBadgeNumber(String badge){ 26 badgeNumber = badge; 27 } 28 public String getBadgeNumber(){ 29 return badgeNumber; 30 } 31 public ParkingTicket patrol(ParkedCar car, ParkingMeter meter){ 32 ParkingTicket parkingTicket = null; 33 if(car.getMinutesParked() > meter.getMinutesPurchased()){ 34 parkingTicket = new ParkingTicket(car, this, car.getMinutesParked() - meter.getMinutesPurchased()); 35 } 36 return parkingTicket; 37 } 38 }
1 public class ParkingTicket{ 2 3 private ParkedCar car; 4 private PoliceOfficer officer; 5 private double fine; 6 private int minutes; 7 public double baseFine = 25.0; 8 public double hourlyFine = 10.0; 9 10 public ParkingTicket(ParkedCar car, PoliceOfficer officer, int meterMins){ 11 this.car = car; 12 this.officer = officer; 13 minutes = meterMins; 14 } 15 public ParkingTicket(ParkingTicket ticket){ 16 17 } 18 public void calculateFine(){ 19 int extraHours = (this.car.getMinutesParked() - 20 this.minutes) / 60; 21 if(extraHours
1 import java.util.Scanner; 2 import java.io.*; 3 4 public class Simulation{ 5 6 public static void main(String[] args)throws IOException{ 7 8 File carData = new File("CarData.txt"); 9 File meterData = new File("MeterData.txt"); 10 Scanner carScan = new Scanner(carData); 11 Scanner meterScan = new Scanner(meterData); 12 13 ParkedCar[] parkedCar = new ParkedCar[1000]; 14 ParkingMeter[] parkingMeter = new ParkingMeter[1000]; 15 ParkingTicket[] parkingTicket = new ParkingTicket[1000]; 16 PoliceOfficer policeOfficer = new PoliceOfficer("Monster Truck", "1337"); 17 18 for(int i = 0 ; i
I. ParkedCar Class Implement the ParkedCar class according to the UML diagram below. This class is meant to hold information about a car. It has a cars make, model, color, license plate and how long the car has been parked. Make sure your fields have the correct access modifiers. Include setter and getter methods for all of the fields, even if they are not in the UML. ParkedCar - make : String - model: String - color : String - licensePlate : String - minutesParked : int + ParkedCar( mk:String, md:String, col:String, lic:String, minParked:int) + ParkedCar( car:ParkedCar) + toString(): String add setter and getter methods II. ParkingMeter Class Implement the ParkingMeter class according to the UML diagram below. The Parking Meter class is meant to hold information about the number of minutes that have been purchased. Include a toString() method, a setter and a getter method for the field. ParkingMeter - minutesPurchased : int + ParkingMeter( minPurchased : int) + toString(): String add setter and getter methods III. Police Officer Class Implement the Police Officer class according to the UML diagram below. This class is meant to hold information about the police officer. Police officers name and badge number. Besides the constructors, toString and setter/getter methods, there is one more method named patrol(.The met minutes a car has been parked and the number of minutes purchased on the parking meter. If the minutes parked is greater than the minutes purchased on the parking meter, then a Parking Ticket object is created and returned; otherwise this methods returns null. Later in the simulation class, once you have created objects of type ParkedCar and ParkingMeter, you will call this method by passing into it a ParkedCar object and a ParkingMeter object. Compare their times and either return a Parking Ticket object or null. Police Officer name: String badgeNumber : String + PoliceOfficer( name:String, badge:String) + PoliceOfficer(officer : Police Officer) + patrol(car:ParkedCar, meter:ParkingMeter) : Parking Ticket + toString() : String add setter and getter methods IV. ParkingTicket Class Implement the Parking Ticket class according to the UML diagram below. This class is meant to hold information about the parking ticket. Parking Ticket class is an aggregate class, it contains instance fields that are reference variables to ParkedCar and Police Officer objects. There are two constructors, one of them is a copy constructor. There is also a method called calculate Fine(), this method calculates the fine based on how many minutes the car has been parked illegally. For the first hour or part of the hour that the car has been parked illegally, there is a base fee of $25. After that there is an additional $10 for each hour or part of an hour that the car is parked illegally. For example if the car is parked illegally for 35 minutes, 57 minutes or 60 minutes, then there is only the base fine of $25. If the car is parked 61 minutes illegally than the fine is the base amount plus $10 for the following partial hour. Totaling at $35. ParkingTicket car : ParkedCar officer : Police Officer fine : double minutes : int base_fine : double = 25.0 hourly_fine : double = 10.0 + + Parking Ticket(car:ParkedCar, officer:Police Officer, meterMins:int) + Parking Ticket( ticket : ParkingTicket ) + calculate Fine(): void + toString(): String add setter and getter methods V. Simulation Class This class will be the driver class with the main method. There are several things you need to write in this class. First download the text files CarData.txt" and MeterData.txt". Inspect the files, notice how in CarData.txt" one line has all of the information needed to create an object of type ParkedCar. The text file is set up so that you can read it one line at a time and be able to create an instance of ParkedCar. Write a method which opens and reads the text file. Inside of the method create an array of type ParkedCar and populate it as you read the text file. This method should return a reference to an array of type ParkedCar. Similarly write another method which reads the other text file and returns a reference to an array of type ParkingMeter. Inside of the main method create array references to ParkedCar and ParkingMeter and assign to them the returning values from the methods which read the text files. Create a single instance of Police Officer. And an array of type Parking Ticket which should have null values in the beginning. In the text files provided there are exactly 1000 lines in each file. This means you will have 1000 objects of type ParkedCar, ParkingMeter and Parking Ticket. ParkedCar at index location O will have the ParkingMeter at index location "O" and a Parking Ticket at index location O. Once all of the objects are created, set up a loop and have the Police Officer patrol the cars and meters. Notice how the patrol method returns a Parking Ticket. After that loop through the tickets and count how many cars were parked illegally. Also print out any ParkingTicket which was issued to a Toyota and was over $30. And print out the total amount fines accumulated during the simulation. VI. Sample Output Sample output for this program can be seen below in Figure 1. Make: Toyota Model: Corolla Color: Lime License Number: VDE5469 Minutes Parked: 163 Officer Data: Name: Francis Llewellyn Poncherello Badge Number: 8600 Minutes illegally parked : 83 Fine: 35.0 Make: Toyota Model: 6500 Color: Red License Number: ZDV6758 Minutes Parked: 162 Officer Data: Name: Francis Llewellyn Poncherello Badge Number: 8600 Minutes illegally parked : 66 Fine: 35.0 Make: Toyota Model: Civic Color: Blue License Number: LOL5469 Minutes Parked: 171 Officer Data: Name: Francis Llewellyn Poncherello Badge Number: 8600 Minutes illegally parked: 87 Fine: 35.0 663 vehicles were parked legally. 337 vehicles were parked illegally. City got $8935.0 from parking tickets. ----GRASP: operation complete. Honda, Camry, Orange, LOL1233,116 Ferrari, G500, Red, MRE6758, 171 Ford, G500, Maroon, LOL 9543,132 Hyundai, Corolla,Black, KJH3456,62 Ford, Camry, Purple, MRE3456,146 Hyundai, Huracan, Green, KJH1233,71 Chevrolet, Viper, Maroon, GDF5469,65 Dodge, Viper, Yellow, KJH5469,138 Honda, Corolla, White, LOL0000, 161 Ford, Camry,White, VDE4355, 174 Toyota, Camry, Green, GDF9543,53 Mercedes, Genesis, Pink, VDE4355,53 Ferrari, Model S, Maroon, KJH9543,82 Chevrolet, Genesis, Blue, LOL 4355,106 Hyundai, Model S, Lime, KJH9543,106 BMW, Civic, Pink, KJH5469,100 Ferrari, 3500,Black, KJH5469,171 Carriage, Civic, Maroon, ZDV1233,173 Mercedes, G500, Blue, LOL5469,84 Ford, 335xi, Yellow, ZDV9543,83 Hyundai, Model S, Brown, GDF4355,83 Chevrolet, Model S, Pink, GDF6758,75 Ferrari, Huracan, White, ZDV3456, 75 BMW, Huracan, Lime, KJH6758, 100 Chevrolet, Civic, Green, ASD4355,54 Honda, 335xi, Maroon, NGH4355, 153 Hyundai, G500, White, MRE0000,168 Honda, Camry, Purple, MRE9543,60 Toyota, Genesis,Brown, VDE6758,53 Chevrolet, 335xi,White, LOL4355, 136 Honda, Corvette, Pink, LOL 4355, 102 BMW, Viper, Black, LOL3456,168 Mercedes, Viper, Yellow, ASD6758, 103 BMW, Corvette, Purple, GDF9543,55 Toyota, Camry, Black, ZDV9543,97 Honda, Corolla, Purple, KJH3456,173 Toyota, Civic,Blue, KJH5469,109 Sled, Genesis, White, KJH9543,49 Toyota, Viper,White, ZDV0000,80 Sled, Huracan, Brown, NGH6758,49 BMW, Viper, Maroon, GDF5469,163 Chevrolet, Civic, Brown, GDF9543,162 BMW, Model S, Brown, ASD5469, 111 Dodge, G500, Brown, LOL1233, 135 Honda, 335xi, Lime, VDE 9543,177 Carriage, Civic, Pink, GDF5469,50 BMW, Corolla, Brown, ASD4355, 74 Ferrari, 335xi, Green, GDF9543, 61 Ford, Corvette, Orange, NGH6758,85 Chevrolet, Camry, Red, NGH9543,135 Chevrolet, 3500, Lime, LOL0000, 60 Chevrolet, Corvette, Black, ZDV5469,66 120 101 122 177 190 118 176 199 155 82 156 109 114 113 114 198 156 100 195 188 101 135 177 117 145 185 157 89 155 86 174 97 177 119 135 182 130 196 128 116 178 180 78 183 155 89 147 136 80 129 110
Step by Step Solution
There are 3 Steps involved in it
Step: 1
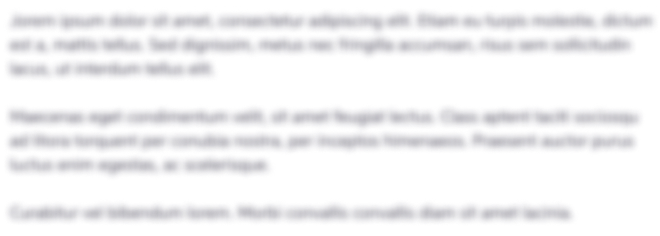
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started