Question
part 1 Hey can someone help me out by modifying my code based on the changes required and please ensure that all the test cases
part 1 Hey can someone help me out by modifying my code based on the changes required and please ensure that all the test cases meet the expected output. Please make sure that the updated code would pass all the test cases giving the appropriate output. Please don't provide a sample code or something similar I have wasted most of my questions by getting them please modify my code to get the expected this is a code for a game of go in python. the exercise description and expected output of the test cases will be provided below the code. please modify the code and provide the modified version without errors as I have tried as much as i can but i keep on messing it up from collections import deque def load_board(filename): result = " " with open(filename) as f: print(f) for index, line in enumerate(f): if index == 0: result += ' '+' '.join([chr(alphabets + 65) for alphabets in range(len(line) - 1)]) + ' ' # the alphabetical column heading result += f"{19 - index:2d}" result += ' ' + ' '.join(line.strip()) + ' ' return result
def save_board(filename, board): with open(filename, "wt") as f: f.write(str(board)) from string import ascii_uppercase as letters class Board: #Dictionary created for the colours and the respected symbols points = {'E': '.', 'B': '@', 'W': 'O'}
#Constructor def __init__(self,board,size=19,from_strings=None): assert 2 <= size <= 26, "Illegal board size: must be between 2 and 26." if from_strings is not None: if type(from_strings) != list: raise AssertionError("input is not a list") if len(from_strings) != size: raise AssertionError("length of input list does not match size") for i in range(size): if type(from_strings[i]) != str: raise AssertionError("row " + str(i) + " is not a string") if len(from_strings[i]) != size: raise AssertionError("length of row " + str(i) + " does not match size") for j in range(size): if from_strings[i][j] not in ".@O": raise AssertionError("invalid character in row " + str(i)) self.size = size self.board=board self.grid = [['E'] * size for _ in range(size)] self.from_strings = [] if from_strings is None else from_strings #self.board= Board #self.from_strings=from_strings
def get_size(self): #Returns the size of the grid created by the constructor return self.size
def __str__(self): # creating the grid padding = ' ' # Creating a variable with a space assigned so that it acts as a padding to the rows that have a single digit heading = ' ' + ' '.join(letters[:self.size]) # Alphabetical heading is created lines = [heading] # adding the alphabetical heading into a list named lines to which the rows will be added later for r, row in enumerate(self.grid): if len(self.grid) < 10: # for the grid with a size less than 10 to add the space to the start of the row for the single digits to be aligned if (self.from_strings): line = " " + f'{self.size - r} ' + ' '.join(self.from_strings[r]) else: line = " " + f'{self.size - r} ' + ' '.join(self.points[x] for x in row) lines.append(line) else: # for the grids that are larger than 9 if r > 9: # for rows 1 to 9 the single digits are aligned according to the first digit from the right of the two digit rows if (self.from_strings): line = f'{self.size - r} ' + ' '.join(self.from_strings[r]) else: line = f'{self.size - r} ' + ' '.join(self.points[x] for x in row) line = padding + line # adding the space using the variable padding to the row created lines.append(line) # adding the row to the list of rows else: # for the rows 10 onwards - as there is no requirement to add a padding it is not added here if (self.from_strings): line = f'{self.size - r} ' + ' '.join(self.from_strings[r]) else: line = f'{self.size - r} ' + ' '.join(self.points[x] for x in row) # creation of the row lines.append(line) # adding the newly created row to the list of rows return ' '.join(lines)
def _to_row_and_column(self, coords): # destructure coordinates like "B2" to "B" and 2 alpha, num = coords colnum = ord(alpha) - ord('A') + 1 rownum = self.size - int(num) + 1 assert 1 <= rownum <= self.size,"row out of range" assert 1 <= colnum <= self.size,'column out of range' return rownum, colnum
def set_colour(self, coords, colour_name): rownum, colnum = self._to_row_and_column(coords) assert len(coords)==2 or len(coords)==3, "invalid coordinates" assert colour_name in self.points,"invalid colour name" self.grid[rownum - 1][colnum - 1] = colour_name
def get_colour(self, coords): rownum, colnum = self._to_row_and_column(coords) return self.grid[rownum - 1][colnum - 1]
def to_strings(self): #padding = ' ' lines = [] for r, row in enumerate(self.grid): if self.from_strings: lines.append(''.join(self.from_strings[r])) else: lines.append(''.join(self.points[x] for x in row)) return lines
def to_integer(self): digit_colour="" for line in self.to_strings(): for character in line: if character=='.': character=0 elif character=='O': character=1 elif character=='@': character=2 character=str(character) digit_colour+=character return int(digit_colour,3)
# return ''.join(self.to_int[x] for line in self.grid for x in line)
def set_from_integer(self, integer_encoding): n = int(integer_encoding) list1 = [] p=[] m=[] t=[] while n != 0: rem = n % self.size #3 list1.append(rem) n = n // self.size#3 list1.reverse() list1=["." if item ==0 else item for item in list1] list1=["O" if item ==1 else item for item in list1] list1=["@" if item ==2 else item for item in list1] for i in range(0,len(list1),self.size):#3 p.append(list1[i:i+self.size])#3 #print(p) for x in p: m=''.join(map(str,x)) t.append(m) #print(Board(self.size,t)) self.from_strings=t
def fill_reaching(self,colour_name,reach_name, visited, r, c): new_list = [] new_list2 = [] for x in self.from_strings: for y in x: if y == ".": y = "E" elif y == "@": y = "B" elif y == "O": y = "W" new_list.append(y) for i in range(0, len(new_list), self.size): new_list2.append(new_list[i:i + self.size]) self.grid = new_list2 if r < 0 or r >= self.size or c < 0 or c >= self.size: #Checking whether the number of rows are within the size of the grid return False if visited[r][c] or self.grid[r][c] != colour_name:#Checking whether the number of columns are within the size of the grid return False if self.grid[r][c] == reach_name: return True visited[r][c] = True if self.fill_reaching(colour_name, reach_name, visited, r + 1, c): return True if self.fill_reaching(colour_name, reach_name, visited, r - 1, c): return True if self.fill_reaching(colour_name, reach_name, visited, r, c + 1): return True if self.fill_reaching(colour_name, reach_name, visited, r, c - 1): return True return False
def reaching_empty_matrix(self): # Create a matrix of the same size as the board, filled with False values matrix = [[False for i in range(self.size)] for j in range(self.size)] #false for the empty grid # Iterate over the points on the board print(self.grid[1][0]) for i in range(self.size): for j in range(self.size): # If the current point is empty or an "O" point, perform a BFS from this point if self.grid[i][j] == "." or self.grid[i][j] == "O": queue = deque([(i, j)]) matrix[i][j] = True while queue: r, c = queue.popleft() for dr, dc in ((1, 0), (-1, 0), (0, 1), (0, -1)): new_r = r + dr new_c = c + dc # If the new point is within the bounds of the board and has not been visited, add it to the queue and mark it as visited if 0 <= new_r < self.size and 0 <= new_c < self.size and not matrix[new_r][new_c]: matrix[new_r][new_c] = True queue.append((new_r, new_c)) return matrix
def is_legal(self): # Iterate over the points on the board for i in range(self.size): for j in range(self.size): # Skip empty points if self.board[i][j] == ".": continue # Check the surrounding points for any illegal positions for dr, dc in ((1, 0), (-1, 0), (0, 1), (0, -1)): new_r = i + dr new_c = j + dc # If the new point is out of bounds or empty, or if it has a different color than the current point, the position is illegal if not (0 <= new_r < self.size and 0 <= new_c < self.size) or self.board[new_r][new_c] == "." or self.board[new_r][new_c] != self.board[i][j]: return False #if we've checked all points and none of them were illegal the position is legal return True
#-------------------------------------TEST CASES------------------------------- #Task 1 b = Board(9) print(b) b = Board() print(b) print(b.get_size()) #Task 2 b = Board(5) b.set_colour("B1", "W") b.set_colour("C1", "B") b.set_colour("D1", "B") print(b) print(b.get_colour("C1")) b.set_colour("C1", "E") print(b) b.set_colour("C1", "G") #Task 3 b = Board(3, ["O.O", ".@.", "@O."]) print(b) print(b.to_strings()) c = Board(b.get_size(), b.to_strings()) print(str(b) == str(c)) #task 4 b = load_board("l19.txt") print(b) save_board("l19b.txt", b) #Task 5 b = Board(3, ["O.O", ".@.", "@O."]) print(b.to_integer()) l19 = load_board("l19.txt") print(l19.to_integer()) c = Board(3) c.set_from_integer(14676) print(c) d = Board(5) d.set_from_integer(1) print(d) #Task 6 b = Board(3, ["@O.", "OOO", ".O."]) print(b) visited = [[False] * 3 for i in range(3)] e = b.fill_reaching("W", "E", visited, 1, 1) print(e) print(visited) visited = [[False] * 3 for i in range(3)] e = b.fill_reaching("B", "E", visited, 0, 0) print(e) print(visited) visited = [[False] * 3 for i in range(3)] e = b.fill_reaching("W", "E", visited, 0, 0) print(e) print(visited) #Task 7 b = Board(3, ["O.O", ".@.", "@O."]) print(b.reaching_empty_matrix()) b = Board(3, ["O@O", ".O.", "@O."]) print(b.reaching_empty_matrix())
l19 = load_board("l19.txt") print(l19) e = l19.reaching_empty_matrix() for r in range(len(e)): for c in range(len(e[r])): if not e[r][c]: print((r, c)) #Task 8 l19 = load_board("l19.txt") print(l19) print(l19.is_legal()) l19.set_colour("E19", "E") print(l19.is_legal()) l19.set_colour("M9", "B") print(l19.is_legal()) print(l19) #Task 10 b = Board(9) b.set_from_integer(123480537448700274288778724742285115148) print(b) b.play_move("B", "D5") print(b) b.play_move("W", "E1") print(b) b.play_move("B", "E7") print(b) b.play_move("W", "E5") print(b)
The description
Task 1 In the file go.py, implement a class Board representing a square Go board. The constructor should take an optional integer parameter size with a default value of 19 to indicate the size of the board. The size must be between 2 and 26, inclusive. If this is not the case, raise an AssertionError with the error message "Illegal board size: must be between 2 and 26.". The constructor should create a square board of the given size with all points initialised as empty.
Implement the method get_size(self) that returns the size of the board.
Implement the method __str__(self) to convert a Board to a string that when printed gives an "ASCII art" representation of the board with coordinates marked with letters and numbers along the columns and rows. Click on the "Expand" button below for an example. Use the following characters to represent the points on the board:
empty: "."
black: "@"
white: "O"
Task 2 Implement a method set_colour(self, coords, colour_name) that changes the colour at the coordinates given by coords. coords must be a string consisting of an upper case letter specifying the column followed by an integer specifying the row. colour_name must be one of the strings "E" (for empty), "B" (for black), "W" (for white). If coords is not a string of length 2 or 3, raise an AssertionError with the string "invalid coordinates". If column or row are out of range, raise an AssertionError with the message "column out of range" or "row out of range", respectively. If the colour name is invalid, raise an AssertionError with the message "invalid colour name".
Implement a method get_colour(self, coords) that returns the colour of the point at the given coordinates. Coordinates and colour names are as described above. Check for valid coordinates as above.
-------------------------Expected output of test cases-------------------- Task 1 A B C D E F G H I 9 . . . . . . . . . 8 . . . . . . . . . 7 . . . . . . . . . 6 . . . . . . . . . 5 . . . . . . . . . 4 . . . . . . . . . 3 . . . . . . . . . 2 . . . . . . . . . 1 . . . . . . . . . A B C D E F G H I J K L M N O P Q R S 19 . . . . . . . . . . . . . . . . . . . 18 . . . . . . . . . . . . . . . . . . . 17 . . . . . . . . . . . . . . . . . . . 16 . . . . . . . . . . . . . . . . . . . 15 . . . . . . . . . . . . . . . . . . . 14 . . . . . . . . . . . . . . . . . . . 13 . . . . . . . . . . . . . . . . . . . 12 . . . . . . . . . . . . . . . . . . . 11 . . . . . . . . . . . . . . . . . . . 10 . . . . . . . . . . . . . . . . . . . 9 . . . . . . . . . . . . . . . . . . . 8 . . . . . . . . . . . . . . . . . . . 7 . . . . . . . . . . . . . . . . . . . 6 . . . . . . . . . . . . . . . . . . . 5 . . . . . . . . . . . . . . . . . . . 4 . . . . . . . . . . . . . . . . . . . 3 . . . . . . . . . . . . . . . . . . . 2 . . . . . . . . . . . . . . . . . . . 1 . . . . . . . . . . . . . . . . . . .
19
Task 2
A B C D E 5 . . . . . 4 . . . . . 3 . . . . . 2 . . . . . 1 . O @ @ .
B A B C D E 5 . . . . . 4 . . . . . 3 . . . . . 2 . . . . . 1 . O . @ .
Traceback (most recent call last): [...] AssertionError: invalid colour name
Step by Step Solution
There are 3 Steps involved in it
Step: 1
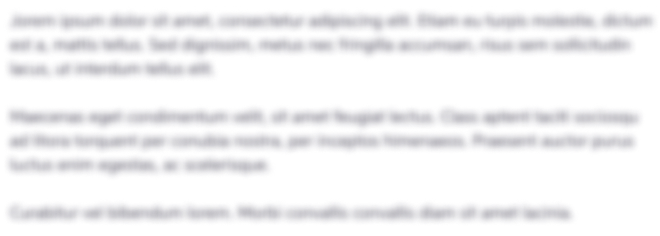
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started