Answered step by step
Verified Expert Solution
Question
1 Approved Answer
# Part 1 # In this part of the prep, you will implement the total _ pay method of the # Employee class. # #
# Part # In this part of the prep, you will implement the totalpay method of the # Employee class. # # You may add new PRIVATE instance attributes to the class to help store a # record of the payments. Private instance attributes are attributes whose # names start with and should not be accessed by code outside of the class. # # Document any new attributes in your class docstring, include type annotations # for them in the class body, and make sure you initialize them properly # in the Employee.init method. You can, and should, also update the # Employee.pay method implementation to update your new attributes # # You may assume as a precondition that the pay method is not called on the # same employee twice in the same month. # # NOTE: You only need to change the Employee class, and you should NOT change # its two subclasses they will simply inherit the Employee.totalpay method ################################################################################ @checkcontracts class Employee: An employee of a company. This is an abstract class. Only child classes should be instantiated. Public Attributes: id: This employee's ID number. name: This employee's name. Private Attributes: add yours here id: int name: str def initself id: int, name: str None: Initialize this employee. Note: This initializer is meant for internal use only; Employee is an abstract class and should not be instantiated directly. self.id id self.name name def getmonthlypaymentself float: Return the amount that this Employee should be paid in one month. Round the amount to the nearest cent. raise NotImplementedError def payself paydate: date None: Pay this Employee on the given date and record the payment. Assume this is called at most once per month. payment self.getmonthlypayment printfAn employee was paid payment on paydate def recordpaymentself float: Record each payment of a employee. cable SalariedEmployee 'cable', cable.paydate An employee was paid on cable.paydate An employee was paid on cable.recordpayment result if self.pay: result return result def totalpayself float: Return the total amount of pay this Employee has received. e SalariedEmployee 'Gilbert the cat', epaydate An employee was paid on epaydate An employee was paid on epaydate An employee was paid on etotalpay # TODO: implement this method! Youll need to add at least one # instance attribute to this class to complete this method. return selfgetmonthlypayment self.recordpayment
# Part
# In this part of the prep, you will implement the totalpay method of the
# Employee class.
#
# You may add new PRIVATE instance attributes to the class to help store a
# record of the payments. Private instance attributes are attributes whose
# names start with and should not be accessed by code outside of the class.
#
# Document any new attributes in your class docstring, include type annotations
# for them in the class body, and make sure you initialize them properly
# in the Employee.init method. You can, and should, also update the
# Employee.pay method implementation to update your new attributes
#
# You may assume as a precondition that the pay method is not called on the
# same employee twice in the same month.
#
# NOTE: You only need to change the Employee class, and you should NOT change
# its two subclasses they will simply inherit the Employee.totalpay method
################################################################################
@checkcontracts
class Employee:
An employee of a company.
This is an abstract class. Only child classes should be instantiated.
Public Attributes:
id: This employee's ID number.
name: This employee's name.
Private Attributes: add yours here
id: int
name: str
def initself id: int, name: str None:
Initialize this employee.
Note: This initializer is meant for internal use only;
Employee is an abstract class and should not be instantiated directly.
self.id id
self.name name
def getmonthlypaymentself float:
Return the amount that this Employee should be paid in one month.
Round the amount to the nearest cent.
raise NotImplementedError
def payself paydate: date None:
Pay this Employee on the given date and record the payment.
Assume this is called at most once per month.
payment self.getmonthlypayment
printfAn employee was paid payment on paydate
def recordpaymentself float:
Record each payment of a employee.
cable SalariedEmployee 'cable',
cable.paydate
An employee was paid on
cable.paydate
An employee was paid on
cable.recordpayment
result
if self.pay:
result
return result
def totalpayself float:
Return the total amount of pay this Employee has received.
e SalariedEmployee 'Gilbert the cat',
epaydate
An employee was paid on
epaydate
An employee was paid on
epaydate
An employee was paid on
etotalpay
# TODO: implement this method! Youll need to add at least one
# instance attribute to this class to complete this method.
return selfgetmonthlypayment self.recordpayment
Step by Step Solution
There are 3 Steps involved in it
Step: 1
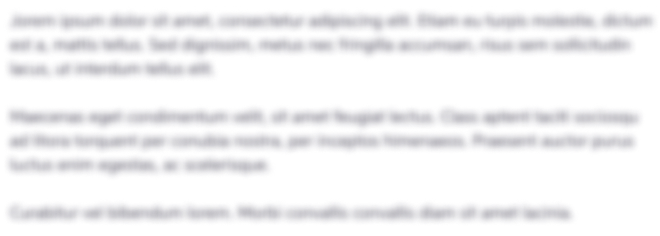
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started