Question
Part 1 Make an ArrayList of Letters, and allow User-Entered Phrase PLEASE BOLD AND PUT ALL NEW CODE INTO THIS CODE. The project contains a
Part 1 Make an ArrayList of Letters, and allow User-Entered Phrase
PLEASE BOLD AND PUT ALL NEW CODE INTO THIS CODE.
The project contains a Letter class that allows you to create letter objects. This stores the character, as well as some information, such as whether or not it is a space character, and whether or not it has been found in the game. Currently, the single phrase in the game (once upon a time), is hard-coded in. In the setupGame method, the letters and spaces in this phrase are used to generate Letter objects, which are then stored in the letter_array. This works fine, because we know there are 16 characters in the phrase. But, its not very useful if we want to let users enter the phrases, which could have any number of characters.
Create an ArrayList in WofFortuneGame to hold the Letter objects in a phrase (note that your ArrayList should NOT be named letter_array, it should be named something more appropriate). Then, change the code so that the once upon a time phrase is stored in this ArrayList and not stored in the letter_array (comment out the letter_array). You will need to adjust code throughout this class to reflect that the Letter objects are now stored in an ArrayList instead of an array. Your project should still run if you have done this properly. A good way to test that this is working is to comment out the letter_array.
Now, add code to the setupGame method (after the part that prints out the rules), to ask the user if they want to enter their own phrase. Then, if they say yes, prompt them to enter the phrase and save their string to the phrase field. If you do this properly, the last part of this method should then add the letters and spaces in the user-entered phrase into the ArrayList you created. Test your code to make sure it works. If the user says no then the default once upon a time phrase should still be used. To do this part, youll need to use the Scanner class. Note that there are already many examples of scanner usage throughout the project, so look at those if you dont remember how to create and use a scanner to get input.
Since this assignment builds up, once you get Part1 working, we suggest you make a backup copy of this assignment before you start in on Part 2. One way to do this is to select the project, right-click and then select Copy then in the dialog box that appears save this project as WofFortune_Project_Part1Done. Then go back and keep working on the original project. CODE FOR THE PROJECT: I am using netbeans
WOFFORTUNEGAME:
package woffortune;
import java.util.Scanner; import java.util.ArrayList;
/** * WofFortuneGame class * Contains all logistics to run the game * @author */ public class WofFortuneGame {
private boolean puzzleSolved = false;
private Wheel wheel; private Player player1; private String phrase = "Once upon a time"; private Letter[] letter_array = new Letter[16];
/** * Constructor * @param wheel Wheel * @throws InterruptedException */ public WofFortuneGame(Wheel wheel) throws InterruptedException { // get the wheel this.wheel = wheel; // do all the initialization for the game setUpGame();
} /** * Plays the game * @throws InterruptedException */ public void playGame() throws InterruptedException { // while the puzzle isn't solved, keep going while (!puzzleSolved){ // let the current player play playTurn(player1); } } /** * Sets up all necessary information to run the game */ private void setUpGame() { // create a single player player1 = new Player("Player1"); // print out the rules System.out.println("RULES!"); System.out.println("Each player gets to spin the wheel, to get a number value"); System.out.println("Each player then gets to guess a letter. If that letter is in the phrase, "); System.out.println(" the player will get the amount from the wheel for each occurence of the letter"); System.out.println("If you have found a letter, you will also get a chance to guess at the phrase"); System.out.println("Each player only has three guesses, once you have used up your three guesses, "); System.out.println("you can still guess letters, but no longer solve the puzzle."); System.out.println(); // for each character in the phrase, create a letter and add to letters array for (int i = 0; i < phrase.length(); i++) { letter_array[i] = new Letter(phrase.charAt(i)); } // setup done } /** * One player's turn in the game * Spin wheel, pick a letter, choose to solve puzzle if letter found * @param player * @throws InterruptedException */ private void playTurn(Player player) throws InterruptedException { int money = 0; Scanner sc = new Scanner(System.in); System.out.println(player.getName() + ", you have $" + player.getWinnings()); System.out.println("Spin the wheel! "); sc.nextLine(); System.out.println(""); Thread.sleep(200); Wheel.WedgeType type = wheel.spin(); System.out.print("The wheel landed on: "); switch (type) { case MONEY: money = wheel.getAmount(); System.out.println("$" + money); break; case LOSE_TURN: System.out.println("LOSE A TURN"); System.out.println("So sorry, you lose a turn."); return; // doesn't get to guess letter case BANKRUPT: System.out.println("BANKRUPT"); player.bankrupt(); return; // doesn't get to guess letter default: } System.out.println(""); System.out.println("Here is the puzzle:"); showPuzzle(); System.out.println(); System.out.println(player.getName() + ", please guess a letter."); //String guess = sc.next(); char letter = sc.next().charAt(0); if (!Character.isAlphabetic(letter)) { System.out.println("Sorry, but only alphabetic characters are allowed. You lose your turn."); } else { // search for letter to see if it is in int numFound = 0; for (Letter l : letter_array) { if ((l.getLetter() == letter) || (l.getLetter() == Character.toUpperCase(letter))) { l.setFound(); numFound += 1; } } if (numFound == 0) { System.out.println("Sorry, but there are no " + letter + "'s."); } else { if (numFound == 1) { System.out.println("Congrats! There is 1 letter " + letter + ":"); } else { System.out.println("Congrats! There are " + numFound + " letter " + letter + "'s:"); } System.out.println(); showPuzzle(); System.out.println(); player.incrementScore(numFound*money); System.out.println("You earned $" + (numFound*money) + ", and you now have: $" + player.getWinnings());
System.out.println("Would you like to try to solve the puzzle? (Y/N)"); letter = sc.next().charAt(0); System.out.println(); if ((letter == 'Y') || (letter == 'y')) { solvePuzzleAttempt(player); } } } } /** * Logic for when user tries to solve the puzzle * @param player */ private void solvePuzzleAttempt(Player player) { if (player.getNumGuesses() >= 3) { System.out.println("Sorry, but you have used up all your guesses."); return; } player.incrementNumGuesses(); System.out.println("What is your solution?"); Scanner sc = new Scanner(System.in); sc.useDelimiter(" "); String guess = sc.next(); if (guess.compareToIgnoreCase(phrase) == 0) { System.out.println("Congratulations! You guessed it!"); puzzleSolved = true; // Round is over. Write message with final stats // TODO } else { System.out.println("Sorry, but that is not correct."); } } /** * Display the puzzle on the console */ private void showPuzzle() { System.out.print("\t\t"); for (Letter l : letter_array) { if (l.isSpace()) { System.out.print(" "); } else { if (l.isFound()) { System.out.print(Character.toUpperCase(l.getLetter()) + " "); } else { System.out.print(" _ "); } } } System.out.println(); } /** * For a new game reset player's number of guesses to 0 */ public void reset() { player1.reset(); } }
Please bold all new code you input thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
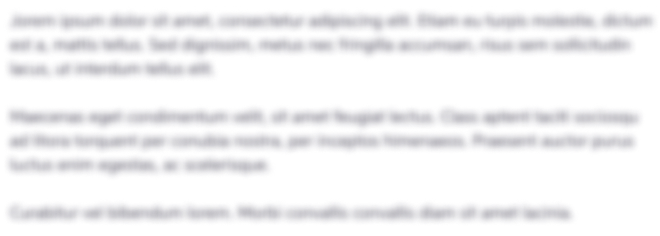
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started