Question
Part 2: Implementing the Game Mechanics (15 points) EDIT: This problem has all the information applied in accordance to the worksheet assigned. However, do know
Part 2: Implementing the Game Mechanics (15 points)
EDIT: This problem has all the information applied in accordance to the worksheet assigned. However, do know that it is done on C++ and is compiled via Visual Studio 2019. Hope this helps.
Overview In this section, you will implement the following functions, to be submitted as source code via Canvas:
In the BattleBoat class:
bool isHit(unsigned int x, unsigned int y) const;
void hit(unsigned int x, unsigned int y);
bool isSunk() const;
In the BattleBoatsGame class:
bool shot(unsigned int x, unsigned int y);
const bool isRevealed(unsigned int x, unsigned int y) const;
unsigned int getBoatsSunk() const;
In addition, you will develop a user interface for playing a game of Battleboats that must be checked off in person by the instructor or an SI.
Backend functions
The actual game progresses as the human player takes shots at the computers board. To implement this game mechanic, you will need to implement two more functions for BattleBoat:
bool isHit(unsigned int x, unsigned int y) const;
void hit(unsigned int x, unsigned int y);
isHit() should take the x- and y- coordinates of a location on the game board and return true if the boat has been hit at that location, or false otherwise. You will need to convert these global coordinates to the index of a segment in the boat (if the boat covers the coordinates specified). If the boat does not cover the coordinates specified, return false.
hit() should take the x- and y- coordinates of a location on the game board and mark that the boat has been hit at that location (if the boat covers the coordinates specified). As with isHit(), you will need to convert the global coordinates to the index of a segment in the boat.
Once you have written those two BattleBoat functions, you can start working on the BattleBoatsGame class. This class already has a constructor, some helper functions, and a couple variables defined, including a reference to an instance of BattleBoatBoard that you should use for the game.
First, you need to write the definition of a function called shot() in the BattleBoatsGame class.
bool shot(unsigned int x, unsigned int y);
This function should attempt to hit a boat at the coordinates (x, y) and return a value as follows:
If there is no boat at the location, or the user has already attacked that location, or the location is out of bounds, shot() should return false.
If there is a boat at the location and the location has not been previously attacked, shot() should return true.
You should also increment the turnCount variable maintained by BattleBoatsGame.
In addition, shot() should somehow mark the square that was targeted as revealed (you may want to use a 2D array to keep track of this). However you keep track of this, implement isRevealed()to return true if the specified coordinates have been revealed, or false otherwise:
const bool isRevealed(unsigned int x, unsigned int y) const;
If x or y is out of bounds, this function should just return false.
Once you have implemented shots and hits, you will need to implement functions to keep track of which boats have been sunk.
In the BattleBoat class, you should implement the isSunk() function that has been declared:
bool isSunk() const;
This function should return true if every segment in the boat has been hit (each square on the board that the ship occupies), or false otherwise (if any square that it occupies has not been hit).
Once you have done that, you should be able to use BattleBoat::isSunk() to implement a function called getBoatsSunk() in the BattleBoatsGame class that counts the number of boats that are sunk:
unsigned int getBoatsSunk() const;
User interface
To finish part 2, you will need to extend your user interface from part 1 to allow the user to play the game. As with part 1, in addition to submitting your code to Canvas, you must get the user interface checked off in person by either the instructor or an SI.
Normally, once the board is created, you would hide it from the player so they cant cheat. However, for the sake of testing (and grading), you need to offer the option of printing the cheat board. This feature is called debug mode. After setting up the board using your interface in part 1, the game should ask the user if they want to see the board. If they respond Y, then you should print out the board as you did in part 1. If the user does not indicate that they want to see the cheat board, do not print it.
Once youve established whether to play in debug mode, you enter the main game loop. Each turn, the user will be prompted for a location x, y to attack on the board. You can assume that the user will enter (x,y) coordinates in the format: x y (that is, without parentheses or a comma).
If there is no boat at the location, or if the user has already attacked that location or the location is out of bounds, print miss. If there is a boat, print hit. If the boat sinks, print sunk. Do not print hit again if the user has already hit this location.
Before each turn, a partially visible representation of the board should be printed to the screen.
For your convenience, a stream insertion operator (<<) has been provided for BattleBoatsGame. This allows you to print the board just like you would any other variable:
// Assume board has been initialized. BattleBoatsGame game { board }; cout << game;
The board will be printed using ASCII art (see example to the right).
Example: Suppose just one boat is placed with the coordinates (0, 0), (0, 1), (0, 2). Turn 1: The user selects (1, 0) and miss is printed Turn 2: The user selects (0, 0) and hit is printed Turn 3: The user selects (0, 0) again; miss is printed Turn 4: The user selects (0, 1) and hit is printed Turn 5: The user selects (-1, 0), which is out of bounds. miss is printed. Turn 6: The user selects (0, 2) and sunk is printed. The game ends because the last boat has sunk. The total number of turns is
6. 0 1 2 3 4 5 6 7 8 9 0 ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ 1 ~~~ ~~~~~~~~~~~~~~~ ~~~~~~ 2 ~~~~~~~~~~~~~~~~~~~~~~~~ ~~~ 3 ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ 4 ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ 5 ~~~~~~~~~ X X X X ~~~~~~~~~ 6 ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ 7 ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ 8 ~~~~~~ ~~~~~~~~~~~~~~~~~~~~~ 9 ~~~~~~~~~~~~~~~~~~~~~~~~~~~ 9
In this visual representation, Xs represent hits i.e. (3,5) through (6, 5) blank areas represent misses i.e. (1, 1), (2, 8), (9, 9), etc. and tildes (~~~) represent fog that is, spaces that havent been guessed yet.
This game board should be printed in addition to the debug board if playing in debug mode.
The game ends when all the boats have been sunk. The game should report how many turns it took to sink all the boats. Lower scores are better!
Part 2 Summary
To receive full credit for Part 2, your implementation of BattleBoatsGame must pass all the tests provided in the starter project. Your implementations of isHit(), hit(), and isSunk() for the BattleBoat class must also pass all of their associated tests. You should see the text Part 2 passed printed out when you run the tests. Your interface must be functional in that it is possible to hit ships, sink ships, and end the game when all ships are sunk, and it must be checked off in person by the instructor or an SI.
As with part 2, your code may also be judged based on style if the instructor cant understand what you did because of a lack of comments or poor organization, expect to lose points
BattleBoat Class.
#include "BattleBoat.h" #include using namespace std;
BattleBoat::BattleBoat(unsigned int length) : length { min(MAX_LENGTH, length) } { }
unsigned int BattleBoat::getLength() const { return length; }
unsigned int BattleBoat::getStartX() const { return startX; }
unsigned int BattleBoat::getStartY() const { return startY; }
bool BattleBoat::isVertical() const { return vertical; }
ostream& BattleBoat::printSegment(ostream& out, unsigned int x, unsigned int y) const { if (length == 1) { // Single cell boat out << "<"; if (hits[0]) { out << "X"; } else { out << "O"; }
out << ">"; } else if (vertical) { if (y == startY) { // Top end cap out << "/";
if (hits[0]) { out << "X"; } else { out << "O"; }
out << "\\"; } else if (y == startY + length - 1) { // Bottom end cap out << "\\";
if (hits[length - 1]) { out << "X"; } else { out << "O"; }
out << "/"; } else { // Middle vertical segment out << "|";
if (hits[y - startY]) { out << "X"; } else { out << "O"; }
out << "|"; } } else { // Left end cap if (x == startX) { out << "<";
if (hits[0]) { out << "X"; } else { out << "O"; }
out << "="; } else if (x == startX + length - 1) { // Right end cap out << "=";
if (hits[length - 1]) { out << "X"; } else { out << "O"; }
out << ">"; } else { // Middle horizontal segment out << "=";
if (hits[x - startX]) { out << "X"; } else { out << "O"; }
out << "="; } }
return out; }
void BattleBoat::setStartX(unsigned int startX) { this->startX = startX; }
void BattleBoat::setStartY(unsigned int startY) { this->startY = startY; }
void BattleBoat::setVertical(bool vertical) { this->vertical = vertical; }
bool BattleBoat::intersects(const BattleBoat& other) const { // TODO: Implement this function return false; }
bool BattleBoat::isHit(unsigned int x, unsigned int y) const { // TODO: Implement this function return false; }
void BattleBoat::hit(unsigned int x, unsigned int y) { // TODO: Implement this function }
bool BattleBoat::isSunk() const { // TODO: Implement this function return false; }
BattleBoatGame Class:
#include "BattleBoatsGame.h" #include "BattleBoat.h" #include using namespace std;
const bool BattleBoatsGame::isRevealed(unsigned int x, unsigned int y) const { // TODO: implement this function return false; }
bool BattleBoatsGame::shot(unsigned int x, unsigned int y) { // TODO: implement this function return false; }
unsigned int BattleBoatsGame::getBoatsSunk() const { // TODO: implement this function return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
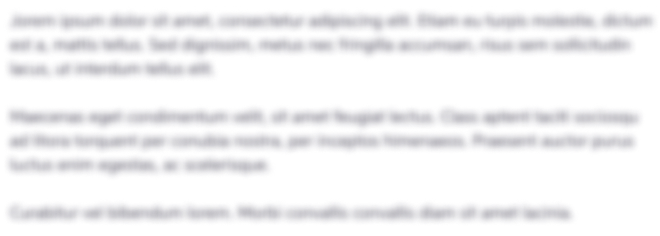
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started