Question
Part 3: Server and client versions For this part of the assignment you may assume that the Geometric Shape Constructor Application is running on the
Part 3: Server and client versions For this part of the assignment you may assume that the Geometric Shape Constructor Application is running on the server. The server calculates area and perimeter of the object sent from the client. The system is sending and receiving data using the User Datagram Protocol (UDP). A client sends an objects related information Object type and its attributes example radius/width/length to the server. The server will validate the message received from the client, that, it calculates area and perimeter of the Geometric Object. The server then sends back the message to the client. The server's port number for this assignment is 20171. The server, may or may not implement the GUI. The server program must continue to listen to the client. There is no need of reading or saving data files. My Classes
GeometricObject.java
public abstract class GeometricObject
{ protected String colour; protected boolean filledIn;
// Construct a geometric object public GeometricObject(String colour, boolean filledIn) { this.colour = colour; this.filledIn = filledIn; }
// Return colour public String getColour() { return colour; }
// Set a new colour public void setColour(String colour) { this.colour = colour; }
// Abstract method findArea public abstract double findArea();
// Abstract method findPerimeter() public abstract double findPerimeter();
public String toString() { return " Colour: " + colour + " and filled " + filledIn; } }
Circle.java
public class Circle extends GeometricObject { private double radius;
// Constructor public Circle(String colour, boolean filledIn, double radius) { super(colour, filledIn); this.radius = radius; }
// Get and set methods for radius public double getRadius() { return radius; }
public void setRadius(double radius) { this.radius = radius; }
// Methods to calculate area and perimeter public double findArea() { return Math.PI * radius * radius; }
public double findPerimeter() { return 2 * Math.PI * radius; }
// toString method public String toString() { String toScreen; toScreen = super.toString() + "Radius of a circle is " + radius; return toScreen; } }
Rectangle.java
public class Rectangle extends GeometricObject
{ private double width; private double length;
// Constructor public Rectangle(String colour, boolean filledIn, double width, double length) { super(colour, filledIn); this.width = width; this.length = length; }
// Getters and setters public double getWidth() { return width; }
public void setWidth(double width) { this.width = width; }
public double getLength() { return length; }
public void setLength(double length) { this.length = length; }
// Calculate area and perimeter public double findArea() { return length * width; }
public double findPerimeter() { return 2 * (length + width); }
// Override parent toString method public String toString() { String toScreen; toScreen = super.toString() + "Width and length of a rectangle is " + width + " " + length + " respectively"; return toScreen; } }
Triangle.java
public class Triangle extends GeometricObject { private double side1; private double side2; private double side3;
//Constructor public Triangle(String colour, boolean filledIn, double side1, double side2, double side3) { super(colour, filledIn); this.side1 = side1; this.side2 = side2; this.side3 = side3; }
//Get and Set methods public double getSide1() { return side1; }
public void setSide1(double side1) { this.side1 = side1; }
public double getSide2() { return side2; }
public void setSide2(double side2) { this.side2 = side2; }
public double getSide3() { return side3; }
public void setSide3(double side3) { this.side3 = side3; }
//Methods to calculate area and perimeter @Override public double findArea() { double p = (side1+side2+side3)/2; double area = Math.sqrt(p*(p-side1)*(p-side2)*(p-side3)); return area; }
@Override public double findPerimeter() { return (side1+side2+side3)/3; } }
Cylinder.java
public class Cylinder extends GeometricObject
{ private double radius; private double height;
//Constructor public Cylinder(String colour, boolean filledIn, double radius,double height) { super(colour, filledIn); this.radius = radius; this.height = height; } //Get and Set methods public double getRadius() { return radius; } public void setRadius(double radius) { this.radius = radius; } public double getHeight() { return height; } public void setHeight(double height) { this.height = height; } // Methods to calculate area and perimeter public double findArea() { return 2* Math.PI * radius *(height+ radius); } public double findPerimeter() { return 2 * (2* Math.PI * radius+height); } //toString method public String toString() { return super.getColour() + "\t" + super.filledIn + "\t" + getRadius()+ "\t" + getHeight(); } }
EquilateralTriangle.java
public class EquilateralTriangle extends GeometricObject
{ private double side; //Constructor public EquilateralTriangle(String colour, boolean filledIn, double side) //Constructor { super(colour, filledIn); this.side = side; } //Get and Set methods public double getSide() { return side; } public void setSide(double side) { this.side = side; } // Methods to calculate area and perimeter public double findArea() { return ( Math.sqrt(3) * side *side)/4; } public double findPerimeter() { return 3 * side; } //toString method public String toString() { return super.getColour() + "\t" + super.filledIn + "\t" + getSide(); } }
Question5Frame.java
import javax.swing.* ;
import java.awt.* ; import java.awt.event.*; public class Question5Frame { public static void main(String [] args) { JFrame myFrame = new JFrame("Lab 3: Question 5") ; // create an instance of Question5Panel and add to frame Question5Panel myPanel = new Question5Panel( ) ; myFrame.add (myPanel ); // set up functionality of frame myFrame.setSize(500, 310 ); myFrame.setVisible(true); myFrame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE) ;;
}//end main }
Question5Panel.java
//import packages
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.*; import javax.swing.JPanel; import javax.swing.border.*;
public class Question5Panel extends JPanel implements ActionListener { // data declarations private JLabel shapeLabel; private JRadioButton circleButton; private JRadioButton rectangleButton; private JRadioButton equTButton; private JRadioButton cylinderButton; private ButtonGroup shape; private JPanel selectShape; private JLabel radius; private JTextField radiusIn; private JLabel rectangle; private JLabel length; private JButton file; private JTextField lengthIn; private JLabel width; private JTextField widthIn; private JLabel side; private JTextField sideIn; private JLabel cylinder; private JLabel height; private JTextField heightIn; private JLabel ra; private JTextField raIn; private JPanel westPanel; private JPanel circlePanel; private JPanel rectanglePanel; private JPanel equTPanel; private JPanel cylinderPanel; private JPanel inputPanel; private JPanel outputPanel; private JTextArea output; private Circle myCircle; private Rectangle myRectangle; private Rectangle myEquTriangle; private Rectangle myCylinder; // constructor to initiate data and set up GUI public Question5Panel() { setLayout(new BorderLayout()); // setting up north area of GUI shapeLabel = new JLabel("Please select a shape "); shapeLabel.setFont(new Font("Arial", Font.BOLD, 16)); // organizing radio buttons and their behaviours circleButton = new JRadioButton("Circle"); rectangleButton = new JRadioButton("Rectangle"); equTButton = new JRadioButton("EquilateralTriangle"); cylinderButton = new JRadioButton("Cylinder"); shape = new ButtonGroup(); shape.add(circleButton); shape.add(rectangleButton); shape.add(equTButton); shape.add(cylinderButton); // adding components to panel to be located north in the GUI selectShape = new JPanel(); selectShape.add(shapeLabel); selectShape.add(circleButton); selectShape.add(rectangleButton); selectShape.add(equTButton); selectShape.add(cylinderButton); add(selectShape, BorderLayout.NORTH); // setting up west area of GUI westPanel = new JPanel(); // setting up components for the circlePanel of the GUI circlePanel = new JPanel(); radius = new JLabel("radius is: "); radiusIn = new JTextField(10); circlePanel.add(radius); circlePanel.add(radiusIn); TitledBorder circleBorder = BorderFactory.createTitledBorder("Circle"); circlePanel.setBorder(circleBorder); add(westPanel, BorderLayout.WEST); // setting up components for the rectanglePanel of GUI length = new JLabel("length is: "); lengthIn = new JTextField(10); width = new JLabel("width is: "); widthIn = new JTextField(10);
// adding components to the rectanglePanel of GUI rectanglePanel = new JPanel(); rectanglePanel.add(length); rectanglePanel.add(lengthIn); rectanglePanel.add(width); rectanglePanel.add(widthIn); TitledBorder rectangleBorder = BorderFactory.createTitledBorder("Rectangle"); rectanglePanel.setBorder(rectangleBorder); // setting up components for the equTPanel of the GUI equTPanel= new JPanel(); side = new JLabel("side is: "); sideIn = new JTextField(10); equTPanel.add(side); equTPanel.add(sideIn); TitledBorder equtBorder = BorderFactory.createTitledBorder("EquilateralTriangle"); equTPanel.setBorder(equtBorder); // setting up components for the cylinderPanel of GUI height = new JLabel("height is: "); heightIn = new JTextField(10); ra = new JLabel("radius is: "); raIn = new JTextField(10); // adding components to the cylinderPanel of GUI cylinderPanel = new JPanel(); cylinderPanel.add(height); cylinderPanel.add(heightIn); cylinderPanel.add(ra); cylinderPanel.add(raIn); TitledBorder cylinderBorder = BorderFactory.createTitledBorder("Cylinder"); cylinderPanel.setBorder(cylinderBorder); add(westPanel, BorderLayout.WEST);
file = new JButton("Read from file"); inputPanel = new JPanel(); inputPanel.add(file); TitledBorder inputBorder = BorderFactory.createTitledBorder("Input File"); inputPanel.setBorder(inputBorder); add(westPanel, BorderLayout.WEST);
// adding the circlePanel, rectanglePanels, equTPanel, and cylinderPanel into the westPanel westPanel.setLayout(new GridLayout(2, 1)); westPanel.setPreferredSize(new Dimension(400, 15)); westPanel.add(circlePanel); westPanel.add(rectanglePanel); westPanel.add(equTPanel); westPanel.add(cylinderPanel); westPanel.add(inputPanel); // setting up center area of GUI outputPanel = new JPanel(); output = new JTextArea(15, 25); outputPanel.add(output); add(outputPanel, BorderLayout.CENTER); // add listeners to radio buttons circleButton.addActionListener(this); rectangleButton.addActionListener(this); equTButton.addActionListener(this); cylinderButton.addActionListener(this); // add listeners to all textfields radiusIn.addActionListener(this); lengthIn.addActionListener(this); widthIn.addActionListener(this); sideIn.addActionListener(this); heightIn.addActionListener(this); raIn.addActionListener(this); file.addActionListener(this); } // end constructor //*************************************************************************** // Students to complete the functionality of the GUI through actionPerformed //***************************************************************************
public void actionPerformed(ActionEvent ae) { System.out.println(ae.getActionCommand()); String data = ""; boolean read = false; if (ae.getActionCommand().equals("Circle")) { data = getData(0); output.setText(data); } else if (ae.getActionCommand().equals("Rectangle")) { data = getData(0, 0); System.out.println(data); output.setText(data); } else if (ae.getActionCommand().equals("EquilateralTriangle")) { data = getData(0); output.setText(data); } else if (ae.getActionCommand().equals("Cylinder")) { data = getData(0, 0); System.out.println(data); output.setText(data); } else if(ae.getActionCommand().equalsIgnoreCase("Read from file")) { String dataFromFile = getDataFromFile(); data = readAndDisplay(dataFromFile); System.out.println(data); output.setText(data); read = true; //set read status to true } if (circleButton.isSelected() && read == false) { System.out.println("--"); try { int i=Integer.parseInt(radiusIn.getText()); data=getData(i); System.out.println(); output.setText(data); } catch (Exception e) { } } if (rectangleButton.isSelected() && read == false) { try { try { int i=Integer.parseInt(lengthIn.getText()); int j=Integer.parseInt(widthIn.getText()); System.out.println(i+","+j); data=getData(i,j); output.setText(data); } catch (Exception e) { } } catch (Exception e) { } } if (equTButton.isSelected() && read == false) { System.out.println("--"); try { int a=Integer.parseInt(sideIn.getText()); data=getData1(a); System.out.println(); output.setText(data); } catch (Exception e) { } } if (cylinderButton.isSelected() && read == false) { try { try { int a=Integer.parseInt(heightIn.getText()); int b=Integer.parseInt(raIn.getText()); System.out.println(a+","+b); data=getData1(a,b); output.setText(data); } catch (Exception e) { } } catch (Exception e) { } } }
private String getData(int i, int j) { Rectangle rectangle=new Rectangle("black",false,i,j); return "rectangle has length of " + i + " with a width of " + j + " " + "and a perimeter of " + rectangle.findPerimeter() + " and a area of " + rectangle.findArea(); } private String getData(int i) { Circle circle = new Circle("black",false,i); return "circle has radius of " + i + " " + "and a perimeter of " + circle.findPerimeter() + " and a area of " + circle.findArea(); } private String getData1(int a, int b) { Cylinder cylinder=new Cylinder("black",false,a,b); return "cylinder has height of " + a+ " with a radius of " + b+ " " + "and a perimeter of " + cylinder.findPerimeter() + " and a area of " + cylinder.findArea(); } private String getData1(int a) { EquilateralTriangle eqt=new EquilateralTriangle("black",false,a); return " Equilaternal triangle has side of " + a + " " + "and a perimeter of " + eqt.findPerimeter() + " and a area of " + eqt.findArea(); }
/** // method to return data read from GeometricObjects.txt * @return */ private String getDataFromFile(){ StringBuilder sb = new StringBuilder(); try { BufferedReader bfr = new BufferedReader(new FileReader("GeometricObjects.txt"));
String eachLine = bfr.readLine(); while (eachLine != null) { sb.append(eachLine); sb.append(System.lineSeparator());//new line eachLine = bfr.readLine();//next line } System.out.println(sb.toString()); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return sb.toString(); }
/** * method to read the string and build the output text * @param dataFromFile * @return */ private String readAndDisplay(String dataFromFile) { String[] data = dataFromFile.split(System.lineSeparator());//split string by new line StringBuilder shapeResult = new StringBuilder();//variable to store the output text try { for (int i = 0; i < data.length; i++) {//iterate through each line String shapeArr[] = data[i].split(",");//seperate using comma switch (shapeArr[0]) {//take first element and check for the shape case "Circle": double radius = Double.parseDouble(shapeArr[1]);//get radius shapeResult.append(" The circle has radius of "); shapeResult.append(radius); Circle cir = new Circle("black", false, radius);//call circle shapeResult.append(" and perimeter of "); shapeResult.append(cir.findPerimeter()); shapeResult.append(" and area of "); shapeResult.append(cir.findArea());//append details to shapeResult break;
case "Rectangle": double len = Double.parseDouble(shapeArr[1]);//get length double wid = Double.parseDouble(shapeArr[2]);//get width shapeResult.append(" The rectangle has length of "); shapeResult.append(len); shapeResult.append(" with a width of "); shapeResult.append(wid); Rectangle rect = new Rectangle("black", false, len, wid);//call rectangle shapeResult.append(" and perimeter of "); shapeResult.append(rect.findPerimeter()); shapeResult.append(" and area of "); shapeResult.append(rect.findArea()); break;
case "Cylinder": double rad = Double.parseDouble(shapeArr[1]);//get radius double ht = Double.parseDouble(shapeArr[2]);//get height shapeResult.append(" The cylinder has radius of "); shapeResult.append(rad); shapeResult.append(" with a height of "); shapeResult.append(ht); Cylinder cylinder = new Cylinder("black", false, rad, ht);//call cylinde shapeResult.append(" and perimeter of "); shapeResult.append(cylinder.findPerimeter()); shapeResult.append(" and area of "); shapeResult.append(cylinder.findArea()); break;
case "EquilateralTriangle": double side = Double.parseDouble(shapeArr[1]);//get side shapeResult.append(" The equilateral triangle has side of "); shapeResult.append(side); EquilateralTriangle eqT = new EquilateralTriangle("black", false, side);//call triangle shapeResult.append(" and perimeter of "); shapeResult.append(eqT.findPerimeter()); shapeResult.append(" and area of "); shapeResult.append(eqT.findArea()); break; case "Triangle": double s1 = Double.parseDouble(shapeArr[1]);//get side double s2 = Double.parseDouble(shapeArr[2]);//get side double s3 = Double.parseDouble(shapeArr[3]);//get side shapeResult.append(" The equilateral triangle has sides of "); shapeResult.append(s1 + "," + s2 + ","+ s3 ); Triangle T = new Triangle("black", false, s1,s2,s3);//call triangle shapeResult.append(" and perimeter of "); shapeResult.append(T.findPerimeter()); shapeResult.append(" and area of "); shapeResult.append(T.findArea()); break; default: shapeResult.append(" Invalid Shape"); break; } } } catch (ArrayIndexOutOfBoundsException e) { shapeResult.append(" Invalid Shape"); } return shapeResult.toString(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
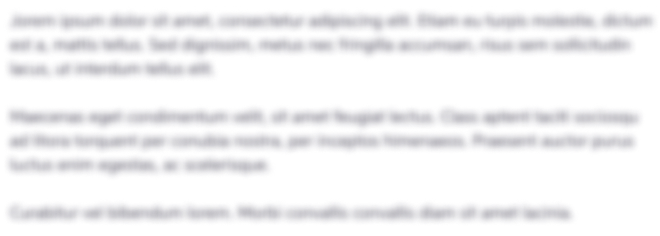
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started