Question
part A: fill in the code in java import java.util.LinkedList; /* * This class models a reference to a published academic journal paper * *
part A: fill in the code in java
import java.util.LinkedList;
/*
* This class models a reference to a published academic journal paper
*
* It stores the paper author, title, journal name, and year of publication
* It also contains a linked list of all papers (each paper stored as a string) that cited this paper
*/
public class Reference implements Comparable
{
String author;
String title;
String journal;
int year;
LinkedList
public Reference(String author, String title, String journal, int year)
{
this.author = author;
this.title = title;
this.journal = journal;
this.year = year;
}
//-----------Start below here. To do: approximate lines of code = 2
// Implement the Comparable interface such that the this and other Reference objects can be
// compared based on the year of publication (ordered oldest to most recent)
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
// Override the equals() method and compare the this and other Reference object
// They are equal if their author and title and journal and year are equal
public boolean equals(Object other)
{
//-----------Start below here. To do: approximate lines of code = 2
//
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
// Override the toString() method and return a string containing
// the title followed by ", " followed by author followed by ", " followed by journal followed by
// ", " followed by year
public String toString()
{
//-----------Start below here. To do: approximate lines of code = 1
//
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
public void addCitation(String paper)
{
citedBy.add(paper);
}
public void printCitations()
{
int counter = 1;
for (String cite: citedBy)
{
System.out.println(counter + ". " + cite);
counter++;
}
}
}
part b:
import java.util.Collections;
import java.util.LinkedList;
import java.util.ListIterator;
/*
* This class stores a linked list of published academic papers (a Reference)
*
* It also has functionality to add a citation to an specific Reference. That is, the citation is in the form of a string
* For example, one Reference might be:
* Deformable Models in Medical Image Analysis: A Survey, McInerney, Medical Image Analysis, 1996
*
* and a paper that was published later cited the paper above might be:
* "Cortical surface-based analysis: Segmentation and surface reconstruction, Dale, Neuroimage, 1999"
*/
public class ReferenceList
{
LinkedList
/*
* Add a paper (a Reference) to the linked list of references
* Keep the References in the linked list sorted based on year of publication (Hint: look at the imports above
* and class Reference)
* Note: before adding, throw a DuplicateReferenceException() if this Reference is
* already in the linked list and pass the toString() of the reference to the constructor
* of the exception object.
*/
public void addPaper(String author, String title, String journal, int year)
{
//-----------Start below here. To do: approximate lines of code = 5
//
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
/*
* Add a citation to the Reference. Uses the author, title, journal, year parameters
* to find the Reference object in the linked list. If the Reference is found, add the citation to it
* If it is not found, throw a ReferenceNotFoundException
*/
public void addCitation(String author, String title, String journal, int year, String citation)
{
//-----------Start below here. To do: approximate lines of code = 8
// Hint: construct a temporary Reference object using the parameters
// and make use of the equals() method in class Reference
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
/*
* Given the parameters, finds the Reference object in the linked list and
* prints all the citations stored in the Reference object
* If the Reference object is not found, throw a ReferenceNotFoundException
*/
public void printCitations(String author, String title, String journal, int year)
{
//-----------Start below here. To do: approximate lines of code = 8
//
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
public void printPapers()
{
for (Reference ref: references)
{
System.out.println(ref.toString());
}
}
}
class DuplicateReferenceException extends RuntimeException
{
public DuplicateReferenceException() {}
public DuplicateReferenceException(String msg)
{
super(msg);
}
}
class ReferenceNotFoundException extends RuntimeException
{
public ReferenceNotFoundException() {}
public ReferenceNotFoundException(String msg)
{
super(msg);
}
}
Step by Step Solution
3.45 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
Referencejava import javautilLinkedList This class models a reference to a published academic journal paper It stores the paper author title journal name and year of publication It also contains a lin...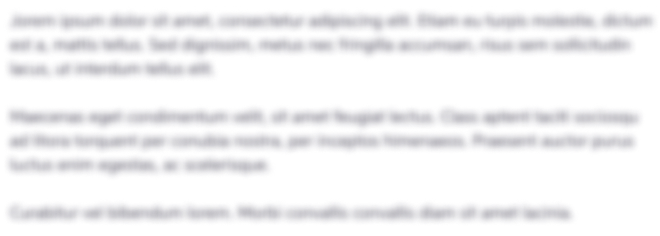
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Document Format ( 2 attachments)
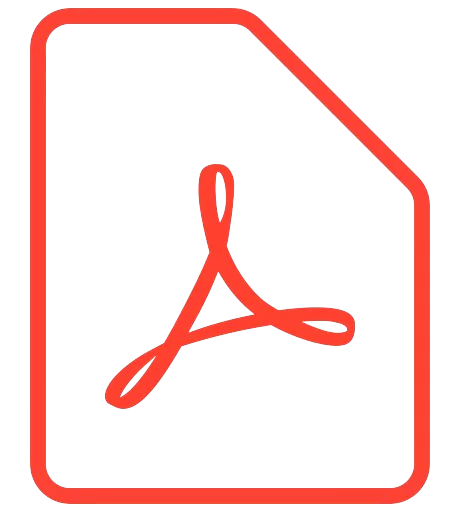
608c00a3823eb_208360.pdf
180 KBs PDF File
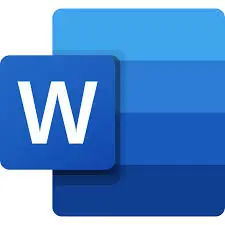
608c00a3823eb_208360.docx
120 KBs Word File
Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started