Question
Part B: The Board Class [25% = 15% test program + 10% documentation] In Part B, you will convert your board module to an encapsulated
Part B: The Board Class [25% = 15% test program + 10% documentation]
In Part B, you will convert your board module to an encapsulated class. The Board class will not have a class invariant.
By the end of Part B, your Board class will have the following public member functions:
Board ();
void print () const;
const Tile& getAt (const CellId& cell_id) const;
void setAt (const CellId& cell_id, const Tile& value, unsigned int owner);
You will also have the following private member functions:
void printColumnNameRow () const;
void printBorderRow () const;
void printEmptyRow () const;
void printDataRow (int row) const;
Perform the following steps:
- Declare a Board class. It should have a 2D array of Tiles as a private member variable. Remove the Board typedef.
- Convert the existing function prototypes to member functions or copy in the ones shown above. Update the implementation file to match.
- Convert the boardInit function into a default constructor. When assigning values to the board array, use the non-default Tile constructor. The syntax is: array_name[i][j] = Tile(put parameters here);
- Change the getAt and setAt functions to take a const reference to a CellId as a parameter instead of a row and a column.
- Add a precondition to the getAt and setAt functions, enforced by an assert. It should require that the cell is on the board.
- Hint: You have a function to check that a cell is on the board.
- Add a parameter to the setAt function for the new tile's owner. The function should set the new tile on the board to have that owner. And a precondition that the tile parameter does not already have an owner.
- Hint: Use a function from the Tile class to change the owner.
- Write interface specifications for the four public functions in the Board class. You do not have to write interface specifications for the private functions.
///////////////////////////////////////////////////////////////// // Tile.h // #pragma once
const unsigned int GENUS_COUNT = 4; const unsigned int GENUS_MONEY = 0; const unsigned int GENUS_DICE = 1; const unsigned int GENUS_POINTS = 2; const unsigned int GENUS_BOMB = 3;
// for money tiles, the species is the money value // for dice tiles, the species is the number of points // for points tiles, the species is the number of points // for bomb tiles, there is no species
class Tile { private: unsigned int owner; unsigned int genus; unsigned int species; void printOwnerChar() const; void printGenusChar() const; void printSpeciesChar() const; bool isInvariantTrue() const; public: Tile(); Tile(unsigned int genus1, unsigned int species1); bool isOwner() const; unsigned int getAffectedPlayer(unsigned int whose_turn) const; unsigned int getGenus() const; unsigned int getSpecies() const; void setOwner(unsigned int owner1); void activate(unsigned int whose_turn) const; void print() const; };
////////////////////////////////////////////////////////////////////////////////////////
// Board.h
//
// A module to represent the game board.
//
#pragma once
#include "BoardSize.h"
#include "Tile.h"
typedef Tile Board[BOARD_SIZE][BOARD_SIZE];
void boardInit (Board board);
const Tile& boardGetAt (const Board board,
int row, int column);
void boardSetAt (Board board,
int row, int column,
const Tile& value,
unsigned int owner);
void boardPrint (const Board board);
void boardPrintColumnNameRow ();
void boardPrintBorderRow ();
void boardPrintEmptyRow ();
void boardPrintDataRow (const Board board,
int row);
//////////////////////////////////////////////////////////
// BoardSize.cpp
//
#include "BoardSize.h"
char getRowName (int row)
{
return 'A' + row;
}
char getColumnName (int column)
{
return '0' + column;
}
/////////////////////////////////////////////////////////////////////////
// Board.cpp
//
#include
#include
#include "BoardSize.h"
#include "Tile.h"
#include "Board.h"
using namespace std;
void boardInit (Board board)
{
for(int r = 0; r < BOARD_SIZE; r++)
{
for(int c = 0; c < BOARD_SIZE; c++)
{
int r2 = abs(r - BOARD_SIZE / 2);
int c2 = abs(c - BOARD_SIZE / 2);
int larger = r2;
if(c2 > r2)
larger = c2;
int money = 4 - larger;
board[r][c] = tileCreate(GENUS_MONEY, money);
}
}
}
const Tile& boardGetAt (const Board board,
int row, int column)
{
return board[row][column];
}
void boardSetAt (Board board,
int row, int column,
const Tile& value,
unsigned int owner)
{
board[row][column] = value;
board[row][column].owner = owner;
}
void boardPrint (const Board board)
{
boardPrintColumnNameRow();
boardPrintBorderRow();
for(int r = 0; r < BOARD_SIZE; r++)
{
boardPrintEmptyRow();
boardPrintDataRow(board, r);
}
boardPrintEmptyRow();
boardPrintBorderRow();
boardPrintColumnNameRow();
}
void boardPrintColumnNameRow ()
{
cout << " ";
for(int c = 0; c < BOARD_SIZE; c++)
{
char label = getColumnName(c);
cout << " " << label << " ";
}
cout << endl;
}
void boardPrintBorderRow ()
{
cout << " +";
for(int c = 0; c < BOARD_SIZE; c++)
{
cout << "-----";
}
cout << "--+" << endl;
}
void boardPrintEmptyRow ()
{
cout << " |";
for(int c = 0; c < BOARD_SIZE; c++)
{
cout << " ";
}
cout << " |" << endl;
}
void boardPrintDataRow (const Board board,
int row)
{
char label = getRowName(row);
cout << " " << label << " |";
for(int c = 0; c < BOARD_SIZE; c++)
{
cout << " ";
tilePrint(board[row][c]);
}
cout << " | " << label << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
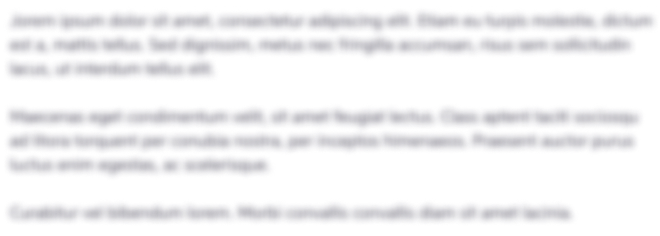
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started