Question
Part II: CD Download the following files: FileIOException.java //has the following public interface: FileIO.java //has the following public interface: o FileIO(String fileName, int operation) //either
Part II: CD Download the following files: FileIOException.java //has the following public interface:
FileIO.java //has the following public interface:
o FileIO(String fileName, int operation) //either FileIO.FOR_READING or FileIO.FOR_WRITING
o boolean EOF() //has the end of the file been reached? o String readLine()
o void writeLine(String line) o void close() //close the file when you are done, especially when writing to a file
Song.java //constructor takes the title and the length of the Song as Strings
CD.java //the year is the search key Complete the CD class by extending KeyedItem. Set the year of the CD as the key field (use the primitive wrapper class for integers, the Integer class). Make sure that there is no setYear method in your CD class to ensure that the key is immutable. A getYear method is not necessary as KeyedItem already has a getKey method. Write the following two methods: public Song getSong(int index)
public void addSong(String title, String length) //create the song and then add it
// FileIO.java
import java.io.*; import java.util.Iterator; import java.util.ArrayList;
/** * This class abstracts the basic Java File I/O classes to make File I/O more straightforward. */
public class FileIO { /** The constant used to indicate that a file is for reading */ public final static int FOR_READING = 1; /** The constant used to indicate that a file is for writing */ public final static int FOR_WRITING = 2;
/** Stores whether the file was intended for writing or for reading */ private int setting;
/** The abstracted BufferedReader if the file was for reading */ private BufferedReader br; /** The abstracted PrintWriter if the file was for writing */ private PrintWriter pw;
/** Indicates whether the end of the file has been reached or not */ private boolean EOF = false; /** Indicates whether the user wanted to extract tokens from each line in the file */ private boolean tokens; /** The delimiter to use if tokens are desired by the user */ private String delimiter;
/** * General purpose constructor that can be used to specify a file and whether it is for reading or for writing. * Preconditions: The file desired (not null) and whether it is for reading or writing must be specified (only options possible). * Postconditions: The specified file is prepared for the desired operation. * Throws: FileIOException if neither reading nor writing is specified or a problem occurs in preparing the file connections. */ public FileIO(String fileName, int operation) throws FileIOException { try { if (operation == FOR_READING || operation == FOR_WRITING) { setting = operation; } else { throw new FileIOException("Must specify reading or writing."); }
File file = new File(fileName);
if (setting == FOR_READING) { FileReader fr = new FileReader(file); br = new BufferedReader(fr); } else if (setting == FOR_WRITING) { FileWriter fw = new FileWriter(file); BufferedWriter bw = new BufferedWriter(fw); pw = new PrintWriter(bw); } } catch (FileNotFoundException fnfe) { throw new FileIOException("File not found."); } catch (IOException ioe) { throw new FileIOException("IO Error"); }
}
/** * Constructor used to read in tokens from a file. * Preconditions: The file desired (not null) and the delimited desired for extracting tokens must be specified. * Postconditions: The specified file is prepared for reading and token extraction. * Throws: FileIOException if a problem occurs in preparing the file connections. */ public FileIO(String fileName, String delimiter) throws FileIOException { try { setting = FOR_READING; tokens = true; this.delimiter = delimiter;
File file = new File(fileName);
FileReader fr = new FileReader(file); br = new BufferedReader(fr); } catch (FileNotFoundException fnfe) { throw new FileIOException("File not found."); } }
/** * Returns the tokens from a single line of text read from a file. * Preconditions: The file must have been opened for reading tokens. * Postconditions: Returns the tokens from a single line of text in the file in an Iterator. * Throws: FileIOException if the file was not opened for reading or if a problem occurs when reading or if the end of the file has been reached. */ public Iterator
if (tokens && !EOF) { String temp = readLine(); if (!EOF) { String[] toks = temp.split(delimiter); list = new ArrayList
for (String str : toks) { list.add(str); } } } else { throw new FileIOException("Tokens not available."); }
return list.iterator(); }
/** * Indicates whether the end of the file has been reached. * Preconditions: None. * Postconditions: Returns true if the end of the file has been reached or false otherwise. */ public boolean EOF() { return EOF; }
/** * Reads in a line of text from a file. * Preconditions: The file must have been opened for reading. * Postconditions: Returns a string with the next line of text from the file or null if the end of the file has been reached. * Throws: FileIOException if the file was not opened for reading or if a problem occurs when reading from the file. */ public String readLine() throws FileIOException {
String temp = null; try { if (setting == FOR_READING) { temp = br.readLine(); if (temp == null) { EOF = true; } } else { throw new FileIOException("File is not open for reading."); } } catch (IOException ioe) { throw new FileIOException("IO Error"); }
return temp; }
/** * Writes a line of text to a file. * Preconditions: The file must have been opened for writing, and the line of text to be written supplied (not null). * Postconditions: The line of text is written to the file. * Throws: FileIOException if the file was not opened for writing or a problem occurs during writing to the file. */ public void writeLine(String line) throws FileIOException {
if (setting == FOR_WRITING) { pw.println(line); } else { throw new FileIOException("File is not open for writing."); }
}
/** * Closes the connection to a file. * Preconditions: None. * Postconditions: The connection to the file is closed. * Throws: FileIOException if a problem occurs when closing the file. */ public void close() throws FileIOException { try { if (setting == FOR_READING) { br.close(); setting = -1; br = null; } else if (setting == FOR_WRITING) { pw.close(); setting = -1; pw = null; } } catch (IOException ioe) { throw new FileIOException("IO Error"); } }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
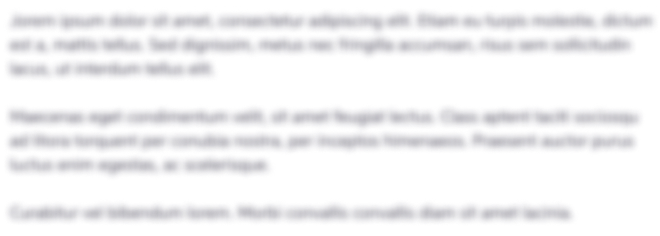
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started