Question
Part II. Java Application: Use the code you submitted in the Project One Milestone to continue developing the game application in this project. Be sure
Part II. Java Application: Use the code you submitted in the Project One Milestone to continue developing the game application in this project. Be sure to correct errors and incorporate feedback before submitting Project One.
Please note: The starter code for this project was provided in the Project One Milestone. If you have not completed the Project One Milestone, you will not be penalized in this assignment, but you will have additional steps to complete to ensure you meet all the components of Project One.
Review the class files provided and complete the following tasks to create a functional game application that meets your clients requirements. You will submit the completed game application code for review.
Begin by reviewing the base Entity class. It contains the attributes id and name, implying that all entities in the application will have an identifier and name.
Software Design Patterns: Review the GameService class. Notice the static variables holding the next identifier to be assigned for game id, team id, and player id.
Referring back to the Project One Milestone, be sure that you use the singleton pattern to adapt an ordinary class, so only one instance of the GameService class can exist in memory at any given time. This can be accomplished by creating unique identifiers for each instance of a game, team, or player.
Your client has requested that the game and team names be unique to allow users to check whether a name is in use when choosing a team name. Referring back to the Project One Milestone, be sure that you use the iterator pattern to complete the addGame() and getGame() methods.
Create a base class called Entity. The Entity class must hold the common attributes and behaviors (as shown in the UML diagram provided in the Supporting Materials section below).
Refactor the Game class to inherit from this new Entity class.
Complete the code for the Player and Team classes. Each class must derive from the Entity class, as demonstrated in the UML diagram.
Every team and player must have a unique name by searching for the supplied name prior to adding the new instance. Use the iterator pattern in the addTeam() and addPlayer() methods.
Functionality and Best Practices
Once you are finished coding, use the main() method provided in the ProgramDriver class to run and test the game application to ensure it is functioning properly.
Be sure your code demonstrates industry standard best practices to enhance the readability of your code, including appropriate naming conventions and in-line comments that describe the functionality.
I have created ENTITY class. But need help with the rest.
GAME.JAVA
package com.gamingroom;
/**
* A simple class to hold information about a game
*
*
* Notice the overloaded constructor that requires
* an id and name to be passed when creating.
* Also note that no mutators (setters) defined so
* these values cannot be changed once a game is
* created.
*
*
* @author coce@snhu.edu
*
*/
public class Game {
long id;
String name;
/**
* Hide the default constructor to prevent creating empty instances.
*/
private Game() {
}
/**
* Constructor with an identifier and name
*/
public Game(long id, String name) {
this();
this.id = id;
this.name = name;
}
/**
* @return the id
*/
public long getId() {
return id;
}
/**
* @return the name
*/
public String getName() {
return name;
}
@Override
public String toString() {
return "Game [id=" + id + ", name=" + name + "]";
}
}
_____________________________________________________________________________
GAMESERVICE.JAVA
package com.gamingroom;
import java.util.ArrayList;
import java.util.List;
/**
* A singleton service for the game engine
*
* @author coce@snhu.edu
*/
public class GameService {
/**
* A list of the active games
*/
private static List
/*
* Holds the next game identifier
*/
private static long nextGameId = 1;
/*Create local instance. The constructor is private and
* it will make this object a singleton.
*/
private static GameService instance = new GameService();
/* A private constructor. A singleton have a private constror
* so taht instantiate would be created outside the class
*/
private GameService() {
}
/* A public accesor fro instance. Allows the outside classess to
* access object in the singleton class
*/
public static GameService getInstance() {
return instance;
}
/**
* Construct a new game instance
*
* @param name the unique name of the game
* @return the game instance (new or existing)
*/
public Game addGame(String name) {
// a local game instance
Game game = null;
// if found, simply return the existing instance
for (Game currentGame : games) {
if (currentGame.getName().equals(name)) {
return currentGame;
}
}
// if not found, make a new game instance and add to list of games
if (game == null) {
game = new Game(nextGameId++, name);
games.add(game);
}
// return the new/existing game instance to the caller
return game;
}
/**
* Returns the game instance at the specified index.
*
* Scope is package/local for testing purposes.
*
* @param index index position in the list to return
* @return requested game instance
*/
Game getGame(int index) {
return games.get(index);
}
/**
* Returns the game instance with the specified id.
*
* @param id unique identifier of game to search for
* @return requested game instance
*/
public Game getGame(long id) {
// a local game instance
Game game = null;
//Scatter current list of games. If it exist return Game instance
// if found, simply assign that instance to the local variable
for (Game currentGame : games) {
if (currentGame.getId()== id) {
game = currentGame;
}
}
return game;
}
/**
* Returns the game instance with the specified name.
*
* @param name unique name of game to search for
* @return requested game instance
*/
public Game getGame(String name) {
// a local game instance
Game game = null;
// if found, simply assign that instance to the local variable
//Scatter the current list of games. If it exist with name
//return Game instance
for (Game currentGame : games) {
if (currentGame.getName().equals(name)) {
game = currentGame;
}
}
return game;
}
/**
* Returns the number of games currently active
*
* @return the number of games currently active
*/
public int getGameCount() {
return games.size();
}
}
____________________________________________________________________________________
PROGRAMDRIVER.JAVA
package com.gamingroom;
/**
* Application start-up program
*
* @author coce@snhu.edu
*/
public class ProgramDriver {
/**
* The one-and-only main() method
*
* @param args command line arguments
*/
public static void main(String[] args) {
GameService service = GameService.getInstance();
System.out.println(" About to test initializing game data...");
// initialize with some game data
Game game1 = service.addGame("Game #1");
System.out.println(game1);
Game game2 = service.addGame("Game #2");
System.out.println(game2);
// use another class to prove there is only one instance
SingletonTester tester = new SingletonTester();
tester.testSingleton();
}
}
_________________________________________________________________________________
PLAYER.JAVA
package com.gamingroom;
/**
* A simple class to hold information about a player
*
* Notice the overloaded constructor that requires
* an id and name to be passed when creating.
* Also note that no mutators (setters) defined so
* these values cannot be changed once a player is
* created.
*
* @author coce@snhu.edu
*
*/
public class Player {
long id;
String name;
/*
* Constructor with an identifier and name
*/
public Player(long id, String name) {
this.id = id;
this.name = name;
}
/**
* @return the id
*/
public long getId() {
return id;
}
/**
* @return the name
*/
public String getName() {
return name;
}
@Override
public String toString() {
return "Player [id=" + id + ", name=" + name + "]";
}
}
_____________________________________________________________________________________
SINGLETONTEST.JAVA
package com.gamingroom;
/**
* A class to test a singleton's behavior
*
* @author coce@snhu.edu
*/
public class SingletonTester {
public void testSingleton() {
System.out.println(" About to test the singleton...");
GameService service = GameService.getInstance();
// a simple for loop to print the games
for (int i = 0; i < service.getGameCount(); i++) {
System.out.println(service.getGame(i));
}
}
}
________________________________________________________________________________
TEAM.JAVA
package com.gamingroom;
/**
* A simple class to hold information about a team
*
* Notice the overloaded constructor that requires
* an id and name to be passed when creating.
* Also note that no mutators (setters) defined so
* these values cannot be changed once a team is
* created.
*
* @author coce@snhu.edu
*
*/
public class Team {
long id;
String name;
/*
* Constructor with an identifier and name
*/
public Team(long id, String name) {
this.id = id;
this.name = name;
}
/**
* @return the id
*/
public long getId() {
return id;
}
/**
* @return the name
*/
public String getName() {
return name;
}
@Override
public String toString() {
return "Team [id=" + id + ", name=" + name + "]";
}
}
________________________________________________________________________
ENTITY.JAVA
package com.gamingroom;
public class Entity {
//private attributes
private long id;
private String name;
//default constructor
private Entity() {
}
//constructor with id & name
public Entity(long id, String name) {
this();
this.id = id;
this.name = name;
}
public long getId() {
return id;
}
public String toString() {
return "Entity [id=" + id + ",name =" + name +"]";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
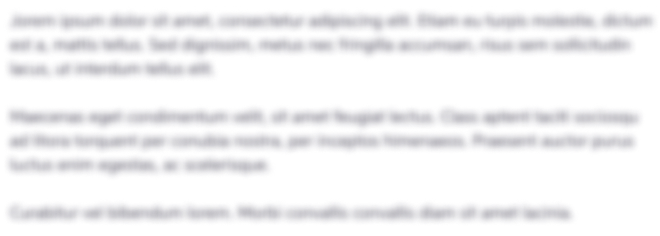
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started