Question
PART ONE: Chapter 11 Lab Exceptions and I/O Streams Lab Objectives Be able to write code that handles an exception Be able to write code
PART ONE:
Chapter 11 Lab Exceptions and I/O Streams Lab Objectives Be able to write code that handles an exception Be able to write code that throws an exception Be able to write a custom exception class Introduction This program will ask the user for a persons name and social security number. The program will then check to see if the social security number is valid. An exception will be thrown if an invalid SSN is entered. Task #1 Understanding a Custom Exception Class SocSecException.java file gives an example of custom exception class. In java, you can use predefined exceptions from J2SE library (check http://docs.oracle.com/javase/7/docs/api/ ), or write a subclass of a pre-defined exceptions. In the subclass, you simply call super class and add specific messages. You do not need to modify the SocSecException.java file. Task #2 Writing Code to Handle an Exception Modify SocSecProcessor.java file to handle an exception: 1. In the main method read a name and social security number from the user as Strings. The main method should contain a try-catch statement. This statement tries to check if the social security number is valid by using the method isValid. If the social security number is valid, it prints the name and social security number. If a SocSecException is thrown, it should catch it and print out the name, social security number entered, and an associated error message indicating why the social security number is invalid. A loop should be used to allow the user to continue until the user indicates that they do not want to continue. 2. The static isValid method: This method throws a SocSecException. True is returned if the social security number is valid, false otherwise. The method checks for the following errors and throws a SocSecException with the appropriate message. a. Number of characters not equal to 11. (Just check the length of the string) b. Dashes in the wrong spots. c. Any non-digits in the SSN. Hint: Use a loop to step through each character of the string, checking for a digit or hyphen in the appropriate spots. 3. Compile, debug, and run your program. Sample output is shown below with user input in bold. OUTPUT (boldface is user input) Name? Sam Sly SSN? 333-00-999 Invalid the social security number, wrong number of characters Continue? y Name? George Washington SSN? 123-45-6789 George Washington 123-45-6789 is valid Continue? y Name? Dudley Doright SSN? 222-00-999o Invalid the social security number, contains a character that is not a digit Continue? y Name? Jane Doe SSN? 333-333-333 Invalid the social security number, dashes at wrong positions Continue? n
SocSecException.java:
public class SocSecException extends Exception { public SocSecException(String error) { super("Invalid the social security number, " + error); } }
SocSecProcessor.java:
import java.util.Scanner;
public class SocSecProcessor { public static void main (String [] args) { Scanner keyboard = new Scanner (System.in); String name; String socSecNumber; String response; char answer = 'Y'; while (Character.toUpperCase(answer) == 'Y') { try { // Task #2 step 1 - To do: // promote user to enter name and ssn number. // save the input to variable name and SocSecNumber. Such as: // System.out.print("Name? "); // name = keyboard.nextLine(); // validate SSN number by calling isValid(socSecNumber). // output the checking result. } catch // To do: catch the SocSecException { System.out.println(e.getMessage()); } System.out.print("Continue? "); response = keyboard.nextLine(); answer = response.charAt(0); } } private static boolean isValid(String number)throws SocSecException { boolean goodSoFar = true; int index = 0; // To do: Task #2 step 2 // 1. check the SSN length. Throw SocSecException if it is not 11 if (number.length() != 11) { throw new SocSecException("wrong number of characters "); } // 2. check the two "-" are in right position. Throw SocSecException if it is not // if (number.charAt(3) != '-') // 3. check other position that should be digits. Throw SocSecException if it is not // if (!Character.isDigit(number.charAt(index))) return goodSoFar; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
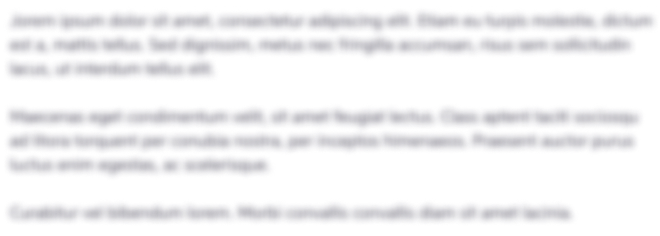
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started