Question
passwords must be at least 6 characters long, and they must use at least 3 of the following 4 types ofcharacters: upper case letters (e.g.,
passwords must be at least 6 characters long, and
they must use at least 3 of the following 4 types ofcharacters:
upper case letters (e.g., A, B, C, ...)
lower case letter (e.g., a, b, c, ...)
digits (e.g., 1, 2, 3, ...)
special characters (e.g., !, @, $, %, ...)
you will implement a program that checks whether a stringentered by the user meets several criteria to qualify as a validpassword.
Create a new Eclipse project by copying ProjectTemplate. Namethe new project PasswordChecker.
Open the src folder of this project and then open (defaultpackage). As a starting point you should useProgramWithIOAndStaticMethod.java. Rename it CheckPassword anddelete the other files from the project.
Edit CheckPassword.java (including updating commentsappropriately) to ask the user for a string (a passwordcandidate), input it, and let the user know whether itsatisfies certain criteria, and if not, which one(s) it fails tosatisfy.
You will do this in stages. In this first step, only check thatthe string satisfies the length requirement. In the next fewsteps, you will add other checks. Test your program after addingeach new check to increase your confidence that the program isworking.
The checking should be done in a static method declared asfollows:
1 2 3 4 5 6 7 8 9 10 11 | /** * Checks whether the given String satisfies the CSE departmentcriteria for * a valid password. Prints an appropriate message to the givenoutput * stream. * * @param s * the String to check * @param out * the output stream */ private static void checkPassword(String s, SimpleWriter out){...} |
Now add a check to checkPassword that the string also containsan upper case letter. For this check you should implement and useanother static method declared as follows:
1 2 3 4 5 6 7 8
| /** * Checks if the given String contains an upper case letter. * * @param s * the String to check * @return true if s contains an upper case letter, falseotherwise */ private static boolean containsUpperCaseLetter(String s){...} |
In a similar fashion, add checks to checkPassword that thestring contains a lower case letter and that it contains a digit.Declare and use appropriate static methods following the example ofcontainsUpperCaseLetter.The boolean static methodsCharacter.isLowerCase(char) andCharacter.isDigit(char) can simplifythis task. Also modify checkPassword so that it rejects a passwordif it does not contain characters from at least two of thethree types checked so far (upper case letters, lowercase letters, and digits).
Complete the password checker by adding the check for specialcharacters (use a new static method like the ones for the otherchecks) and rejecting passwords that do not contain characters fromat least three of the four types. For this stepassume a valid special character is one in the string"!@#$%^&*()_-+={}[]:;,.?".
Modify the main program so that it repeatedly asks the user fora new password, checks it and prints a message. The program shouldexit when the user enters an empty string (i.e., the user justpresses the Enter key without entering any other characters).
LINK:https://www.dropbox.com/s/1wf1u4ir2qcjj2g/Lab_%20Password%20Checker.html?dl=0
SRC LINK:https://www.dropbox.com/s/3f9wibi0ne4t8op/CheckPassword.zip?dl=0
Step by Step Solution
3.50 Rating (157 Votes )
There are 3 Steps involved in it
Step: 1
package checkpassword import static javalangSystemexit import javautilScanner public class CheckPass...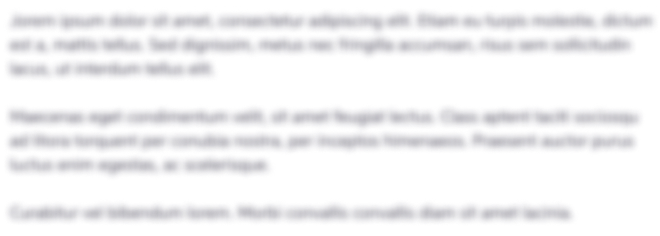
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started