Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Peace be upon you. I want three reports e. Your protocol should support two types of connections: persistent and non- persistent) f. Your server should
Peace be upon you. I want three reports
e. Your protocol should support two types of connections: persistent and non- persistent) f. Your server should support the following functionalities: i. list: this method should be used to list the files in a specific directory ii. make directory: this method is used to create a directory iii. download this method is used to download a specific file iv. upload this method is used to upload a file to a specific directory v. (bonus) delete: this method is used to delete a file vi. exit: this method is used to close the connection if a persistent connection is used vii. (bonus) You server should reply to unknown methods with an error status code g. Your client should use your protocol to allow the user to control remote files and directories. h. Your client should show the user a simple menu and simple readable responses. Do not print status code to the user, in the client code interpret the status code to print human readable messages. (See Appendix I) 3. Implementation and guidelines It is your protocol, so you have control over many things. However, make sure you design an efficient protocol. For example, having many unnecessarily headers is not a good idea, having different message formats for each method will make the protocol complex, try to generalize your format as much as possible. 3.1 Protocol design: When designing your protocol, identify your general request message format and general reply message format. Be specific about what separators between the fields your protocol uses, e.g., space, new line ( ) and so on. Refer to the requirements (section 2, part a). Remember your protocol should support two connection types. (Hint: you need a field to identify the connection type:)) For implementation, you can use string libraries to help you parse the messages at the client and at the server. When the client sends a "list" request, your server should parse the request to figure out what method the client has requested and to properly reply to it. For example, you can use the split() method and any other string method you may need. 2 Below is an example to extract a word from a string where the separator is a space (or multiple space): Python: line = "Hello we are learning about split" words = req.split("") first_word = words[0] fourth_word = words[3) Also, you can use python slicing [1:] to remove some characters from the beginning of a string. You are allowed to use any string's python methods you need Java: String line = "Hello we are learning about split"; String delims = "[]+"; //[ ]+ is a regular expression String [] words = line.split(delims); String first_word = words[0]; String fourth_word = words[3]; System.out.println(first_word); Also, you can use the substring() method to remove some characters from the beginning of a string. You are allowed to use any string's Java methods you need 3.2 LIST List can be used to list files and directories) in either the current directory, or it can be used to list the files of a specific directory. It does not list the files of a directory inside another directory. 3.3 DOWNLOAD The user can use the download method to download a specific file (located at a specific directory) into a specific path at the user machine. For simplicity, you can assume that the downloaded files are always text files. 3 3.4 UPLOAD The user can use the upload method to upload a file into a specific path at the server (can be current directory or another directory, e.g., mydir/mydir2/file.txt). The uploaded file content should match the original file content. For example, if the original file has some new lines, the uploaded file should have new lines well. For simplicity, you can assume that the uploaded files are always text files. 3.5 MAKE DIRECTORY The user can use the make directory method to create new directory into a specific path (can be current directory or another directory, e.g., mydir/mydir2ewdir), If the directory exists, your server should reply with a proper status code indicating that the directory already exists. 3.6 DELETE The user can use the delete method to delete a file of a specific path (can be current directory or another directory, e.g., mydir/mydir2/file.txt). 3.7 EXIT The user can use the exit method to close a persistent connection. The client should wait for a reply message from the server before closing the connection. 3.8 Design and coding style Use descriptive variable names, add comments, and modularize your code by implementing different functions perform different tasks. For example, implement a function to decide which method is requested by the client, add another method for list requests, and so on. 3.9 Guidelines a) Start with the design of your protocol (message format and syntax), then start coding and modify your design as you code b) Strat small by building functionalities one by one. For example, first only write a code to list files in the current directory, then list files of a specific directory, move to reading files which you will need for download requests and so on. (You can start by only listing the files as an output at the server before moving to sending the listed files to the client) c) After having a functional server, you can add the option of persistent and non-persistent feature to your protocol d) Debug your code function by function, do not move to the next function unless you are sure all previous functions are error-free e) Remember to always use try statements to have an error-prone server f) Divide the functionalities between the two team members, start by implementing one function together (e.g., LIST), then move to work separately where each member works on one function alone. 4. Testing a) Test all the functionality one by one using a persistent connection a. LIST current directory files b. Make directory: mydir1 c. Upload file1 to mydir1 d. LIST files of mydiri e. Create mydiri again (should receive an error since the directory exist) What would you like to do? 1. list 2. download 3- upload 4-delete 5- make new directory 6-exit Enter your choice: 2 Enter the name of the file: myfile2 File/directory not found What would you like to do? 1- list 2- download 3- upload 4-delete 5- make new directory 6- exit Enter your choice: 2 Enter the name of the file: myfile Operation succeeded What would you like to do? 1. list 2. download 3- upload 4. delete 5-make new directory 6- exit Enter your choice: 3 Enter the name of the file: myfile1 Enter the destination path: mydir/myfile2 Operation succeeded Note in the above three examples the replies are readable texts and not status codes. 8 Appendix 11 e. Your protocol should support two types of connections: persistent and non- persistent) f. Your server should support the following functionalities: i. list: this method should be used to list the files in a specific directory ii. make directory: this method is used to create a directory iii. download this method is used to download a specific file iv. upload this method is used to upload a file to a specific directory v. (bonus) delete: this method is used to delete a file vi. exit: this method is used to close the connection if a persistent connection is used vii. (bonus) You server should reply to unknown methods with an error status code g. Your client should use your protocol to allow the user to control remote files and directories. h. Your client should show the user a simple menu and simple readable responses. Do not print status code to the user, in the client code interpret the status code to print human readable messages. (See Appendix I) 3. Implementation and guidelines It is your protocol, so you have control over many things. However, make sure you design an efficient protocol. For example, having many unnecessarily headers is not a good idea, having different message formats for each method will make the protocol complex, try to generalize your format as much as possible. 3.1 Protocol design: When designing your protocol, identify your general request message format and general reply message format. Be specific about what separators between the fields your protocol uses, e.g., space, new line ( ) and so on. Refer to the requirements (section 2, part a). Remember your protocol should support two connection types. (Hint: you need a field to identify the connection type:)) For implementation, you can use string libraries to help you parse the messages at the client and at the server. When the client sends a "list" request, your server should parse the request to figure out what method the client has requested and to properly reply to it. For example, you can use the split() method and any other string method you may need. 2 Below is an example to extract a word from a string where the separator is a space (or multiple space): Python: line = "Hello we are learning about split" words = req.split("") first_word = words[0] fourth_word = words[3) Also, you can use python slicing [1:] to remove some characters from the beginning of a string. You are allowed to use any string's python methods you need Java: String line = "Hello we are learning about split"; String delims = "[]+"; //[ ]+ is a regular expression String [] words = line.split(delims); String first_word = words[0]; String fourth_word = words[3]; System.out.println(first_word); Also, you can use the substring() method to remove some characters from the beginning of a string. You are allowed to use any string's Java methods you need 3.2 LIST List can be used to list files and directories) in either the current directory, or it can be used to list the files of a specific directory. It does not list the files of a directory inside another directory. 3.3 DOWNLOAD The user can use the download method to download a specific file (located at a specific directory) into a specific path at the user machine. For simplicity, you can assume that the downloaded files are always text files. 3 3.4 UPLOAD The user can use the upload method to upload a file into a specific path at the server (can be current directory or another directory, e.g., mydir/mydir2/file.txt). The uploaded file content should match the original file content. For example, if the original file has some new lines, the uploaded file should have new lines well. For simplicity, you can assume that the uploaded files are always text files. 3.5 MAKE DIRECTORY The user can use the make directory method to create new directory into a specific path (can be current directory or another directory, e.g., mydir/mydir2ewdir), If the directory exists, your server should reply with a proper status code indicating that the directory already exists. 3.6 DELETE The user can use the delete method to delete a file of a specific path (can be current directory or another directory, e.g., mydir/mydir2/file.txt). 3.7 EXIT The user can use the exit method to close a persistent connection. The client should wait for a reply message from the server before closing the connection. 3.8 Design and coding style Use descriptive variable names, add comments, and modularize your code by implementing different functions perform different tasks. For example, implement a function to decide which method is requested by the client, add another method for list requests, and so on. 3.9 Guidelines a) Start with the design of your protocol (message format and syntax), then start coding and modify your design as you code b) Strat small by building functionalities one by one. For example, first only write a code to list files in the current directory, then list files of a specific directory, move to reading files which you will need for download requests and so on. (You can start by only listing the files as an output at the server before moving to sending the listed files to the client) c) After having a functional server, you can add the option of persistent and non-persistent feature to your protocol d) Debug your code function by function, do not move to the next function unless you are sure all previous functions are error-free e) Remember to always use try statements to have an error-prone server f) Divide the functionalities between the two team members, start by implementing one function together (e.g., LIST), then move to work separately where each member works on one function alone. 4. Testing a) Test all the functionality one by one using a persistent connection a. LIST current directory files b. Make directory: mydir1 c. Upload file1 to mydir1 d. LIST files of mydiri e. Create mydiri again (should receive an error since the directory exist) What would you like to do? 1. list 2. download 3- upload 4-delete 5- make new directory 6-exit Enter your choice: 2 Enter the name of the file: myfile2 File/directory not found What would you like to do? 1- list 2- download 3- upload 4-delete 5- make new directory 6- exit Enter your choice: 2 Enter the name of the file: myfile Operation succeeded What would you like to do? 1. list 2. download 3- upload 4. delete 5-make new directory 6- exit Enter your choice: 3 Enter the name of the file: myfile1 Enter the destination path: mydir/myfile2 Operation succeeded Note in the above three examples the replies are readable texts and not status codes. 8 Appendix 11Step by Step Solution
There are 3 Steps involved in it
Step: 1
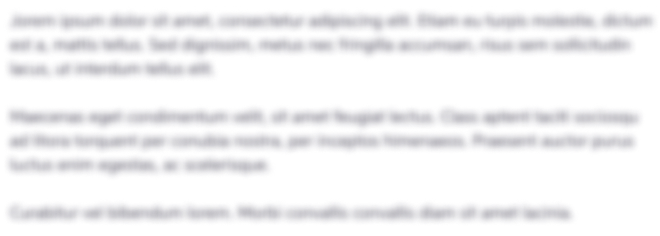
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started