Question
Perform lexical operations that are implemented with a Finite Automata (or Finite State Machine). Write a program in C++ to convert a character representation of
Perform lexical operations that are implemented with a Finite Automata (or Finite State Machine).
Write a program in C++ to convert a character representation of a non-negative real number to a double value without using the string-to-double conversions, must do a character-by-character conversion. Use a separate method to do the actual conversion.
Note the ASCII characters for 0 through 9 are in order.
Do not use hardcoded numbers for ASCII values of digits or any other characters.
real ::= {0 | 1 | | 9}+ . {0 | 1 | | 9}* | {0 | 1 | | 9}* . {0 | 1 | | 9}+
Possible strings include 1., 3.14, .5. Note . must be present.
Something like this:
Token scan_real(char *line, int lookahead) /* scan a real number from input */
{
int pos = lookahead; /* remember start of number */
if (isdigit(line[lookahead])) { /* move from state 1 to state 2 */
while (isdigit(line[++lookahead])) /* stay in state 2 */
;
if (line[lookahead] = = .) { /* move to state 3 */
while (isdigit(line[++lookahead])) /* stay in state 3 */
;
strcpy(token.symbol, line + pos, lookahead pos); /* copy number to token */
}
else
printf(Syntax error: real number doesnt end with decimal point );
}
else if (line[lookahead] = = .) { /* move from state 1 to state 4 */
if (isdigit(line[++lookahead])) /* move from state 4 to state 5 */
same as state 3 above /* stay in state 5 */
else
printf(Syntax error: decimal point must be preceded or followed by digits);
}
else
printf(Syntax error: expected number, found %c , line[lookahead]);
}
0-9 start. 1) 0-9-23 . start 33 40-9.50-9 0-9 start. 1) 0-9-23 . start 33 40-9.50-9Step by Step Solution
There are 3 Steps involved in it
Step: 1
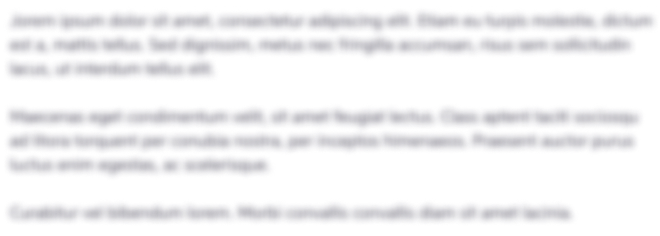
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started