Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Person Class / * * * Person Class * Class contains: First name, Last name, and age * / public class Person { / /
Person Class Person Class Class contains: First name, Last name, and age public class Person Attributesfields private String firstName; private String lastName; private int personAge; Constructors Constructors Default public Person firstName "None"; lastName "None"; personAge ; Constructors Populates data with arguments public PersonString pFirst, String pLast, int pAge setFirstNamepFirst; setLastNamepLast; setPersonAgepAge; GettersSetters public String getFirstName return firstName; public void setFirstNameString firstName this.firstName firstName; public String getLastName return lastName; public void setLastNameString lastName this.lastName lastName; public int getPersonAge return personAge; public void setPersonAgeint personAge this.personAge personAge; Methods public String PrintData String myReturn ; myReturn "Name: getFirstName getLastName; myReturn Age: getPersonAge; return myReturn; END Person Class The provided Java class, Person, models a person and their attributes such as first name, last name, and age. Attributesfields: The class has three private instance variables or attributes firstName, lastName, and personAge. They are private to ensure data encapsulation, which means they can be accessed or modified only through methods within the Person class itself. Constructors: The class provides two constructors: Default constructor public Person: This constructor initializes the firstName and lastName fields to "None" and the personAge to Parameterized constructor public PersonString pFirst, String pLast, int pAge: This constructor uses parameters to initialize the firstName, lastName, and personAge fields. It uses the provided setter methods to set the values. GettersSetters: These are methods that allow outside code to safely access get or modify set the private instance variables. This is a common practice in objectoriented programming to enforce encapsulation. In this class, there are getter and setter methods for each of the three private attributes. Methods: PrintData: This method returns a String which includes the person's first name, last name, and age. It uses the getter methods to access the private instance variables. In summary, this class represents a simple model of a person. It demonstrates several key principles of objectoriented programming, such as encapsulation using private fields and public gettersetter methods constructors to initialize objects, and methods to represent behaviors or actions associated with the object in this case, printing the person's data Driver Program Code writes a file and reads the data into an ArrayList of the Person object import java.util.ArrayList; import java.util.Scanner; import java.ioFile; Import for File Object import java.ioIOException; Import for IO Exceptions import java.ioFileWriter; Import the FileWriter class import java.ioFileNotFoundException; Import this class to handle errors public class LabDriver public static void mainString args Variables we will use in the program ArrayList Students new ArrayList; String firstName; String lastName; int age; int index ; index for the ArrayList Lets create our Data File here we will use a trycatch in the event something goes wrong try Create an instance of the File Writer and give it the filename FileWriter myWriter new FileWriterPersontxt; Write our data to the file myWriter.writeKevin Roark "Sam Read "Sally Smith "Bart Simpson ; close the file we opened MUST BE DONE myWriter.close; Message that all was done System.out.printlnSuccessfully wrote to the file."; catch IOException e An issue happened Message user System.out.printlnAn error occurred."; eprintStackTrace; Now lets read the file into our ArrayList of object Person
Person Class
Person Class
Class contains: First name, Last name, and age
public class Person
Attributesfields
private String firstName;
private String lastName;
private int personAge;
Constructors
Constructors Default
public Person
firstName "None";
lastName "None";
personAge ;
Constructors Populates data with arguments
public PersonString pFirst, String pLast, int pAge
setFirstNamepFirst;
setLastNamepLast;
setPersonAgepAge;
GettersSetters
public String getFirstName
return firstName;
public void setFirstNameString firstName
this.firstName firstName;
public String getLastName
return lastName;
public void setLastNameString lastName
this.lastName lastName;
public int getPersonAge
return personAge;
public void setPersonAgeint personAge
this.personAge personAge;
Methods
public String PrintData
String myReturn ;
myReturn "Name: getFirstName getLastName;
myReturn Age: getPersonAge;
return myReturn;
END Person Class
The provided Java class, Person, models a person and their attributes such as first name, last name, and age.
Attributesfields: The class has three private instance variables or attributes firstName, lastName, and personAge. They are private to ensure data encapsulation, which means they can be accessed or modified only through methods within the Person class itself.
Constructors: The class provides two constructors:
Default constructor public Person: This constructor initializes the firstName and lastName fields to "None" and the personAge to
Parameterized constructor public PersonString pFirst, String pLast, int pAge: This constructor uses parameters to initialize the firstName, lastName, and personAge fields. It uses the provided setter methods to set the values.
GettersSetters: These are methods that allow outside code to safely access get or modify set the private instance variables. This is a common practice in objectoriented programming to enforce encapsulation. In this class, there are getter and setter methods for each of the three private attributes.
Methods:
PrintData: This method returns a String which includes the person's first name, last name, and age. It uses the getter methods to access the private instance variables.
In summary, this class represents a simple model of a person. It demonstrates several key principles of objectoriented programming, such as encapsulation using private fields and public gettersetter methods constructors to initialize objects, and methods to represent behaviors or actions associated with the object in this case, printing the person's data
Driver Program
Code writes a file and reads the data into an ArrayList of the Person object
import java.util.ArrayList;
import java.util.Scanner;
import java.ioFile; Import for File Object
import java.ioIOException; Import for IO Exceptions
import java.ioFileWriter; Import the FileWriter class
import java.ioFileNotFoundException; Import this class to handle errors
public class LabDriver
public static void mainString args
Variables we will use in the program
ArrayList Students new ArrayList;
String firstName;
String lastName;
int age;
int index ; index for the ArrayList
Lets create our Data File
here we will use a trycatch in the event something goes wrong
try
Create an instance of the File Writer and give it the filename
FileWriter myWriter new FileWriterPersontxt;
Write our data to the file
myWriter.writeKevin Roark
"Sam Read
"Sally Smith
"Bart Simpson ;
close the file we opened MUST BE DONE
myWriter.close;
Message that all was done
System.out.printlnSuccessfully wrote to the file.";
catch IOException e
An issue happened Message user
System.out.printlnAn error occurred.";
eprintStackTrace;
Now lets read the file into our ArrayList of object Person
Step by Step Solution
There are 3 Steps involved in it
Step: 1
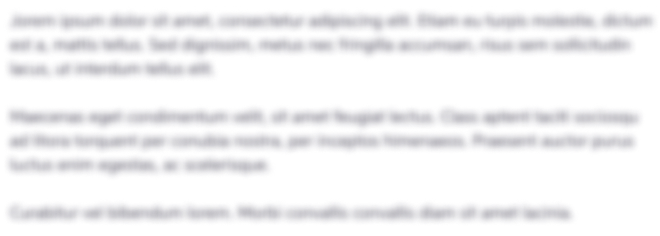
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started