Question
Phase 1: Chemicals First, implement the related classes Chemical and ChemicalList . The Chemical class should have: Two instance variables: the chemicals name (a String
Phase 1: Chemicals
First, implement the related classes Chemical and ChemicalList.
The Chemical class should have:
Two instance variables: the chemicals name (a String), and a list of the other chemicals that it interacts with (a ChemicalList).
A constructor with one parameter giving the chemicals name. The list of interactions should initially be empty.
An instance method void addInteraction(Chemical) which will add an interaction between this chemical and another chemical. This interaction should be added to the list of interactions for both chemicals. So the call one.addInteraction(two) should add two to the list of interactions with one, and also add one to the list of interactions with two.
A standard toString method that returns only the name of the chemical.
A special String toStringExtended() method that will also include the list of interactions, on a separate line, preceded by a tab character (use and \t to create such a String). For example, see the sample output below, which uses this method.
The ChemicalList class should have:
Instance variables that will allow a list of up to 100 Chemical objects to be stored. Use a simple partially-full array to do this. You may assume that the list will never become full no error checking is needed.
A constructor with no parameters that creates an empty list.
An instance method void addChemical(Chemical) which will add a chemical to the list.
A standard toString method which will return the names of the chemicals, separated by commas. There should be no comma after the last chemical. See the sample output below for an example.
You can test your classes using the supplied test program TestPhase1.java. You should get the output shown below.
Chemicals
---------
1: aaratine
interacts with boricium
2: boricium
interacts with aaratine,eekamouse
3: catboxite
interacts with drystophan,eekamouse
4: drystophan
interacts with catboxite
5: eekamouse
interacts with boricium,faroutman,catboxite
6: faroutman
interacts with eekamouse
7: gooeygood
interacts with
Phase 2: Medicines
Next, implement the related classes Medicine and MedicineList.
The Medicine class should have:
Two instance variables: the medicines name (a String), and a list of the chemicals that it contains (a ChemicalList).
A constructor Medicine(String, Chemical[]) which specifies the name of the medicine, and all of the chemicals that it contains. This should be the only constructor. The array of chemicals will be a full array, not a partially full array. You will have to use this Chemical[] to create the necessary ChemicalList.
A standard toString method that returns only the name of the medicine.
A special String toStringWithIngredients() method which will add a list of the chemicals in this medicine, after the name, surrounded by parentheses. See the sample output below.
The MedicineList class should have:
Instance variables that will allow a list of up to 100 Medicine objects to be stored. Use a simple partially-full array to do this. You may assume that the list will never become full no error checking is needed.
A constructor with no parameters that creates an empty list.
An instance method void addMedicine(Medicine) which will add a medicine to the list.
A standard toString method which will return the names of the medicines, separated by commas. There should be no comma after the last medicine. See the sample output below for an example.
Note that this class, so far, is almost identical to the ChemicalList class. You might think that there should be some easy way to create them without so much identical code. There is. We will cover it later in the course.
Modify the Chemical class as follows:
Add an instance variable so that each chemical will keep a list of the medicines that contain it (a MedicineList).
Make appropriate modifications to the constructor.
Add an instance method void addMedicine(Medicine) which will add a medicine to the list of medicines that contain this chemical. This method should be called from the constructor in the Medicine class.
Modify the toStringExtended method to add one more line that lists the medicines that have this chemical as an ingredient. See the sample output below for an example.
You can test your classes using the supplied test program TestPhase2.java. You should get the output shown below.
Chemicals
---------
aaratine
interacts with boricium
ingredient in MiracleCure,Hydramax,TastyPill
boricium
interacts with aaratine,eekamouse
ingredient in ExxoPlexx
catboxite
interacts with drystophan,eekamouse
ingredient in ExxoPlexx
drystophan
interacts with catboxite
ingredient in Hydramax,TastyPill
eekamouse
interacts with boricium,faroutman,catboxite
ingredient in MiracleCure
faroutman
interacts with eekamouse
ingredient in Hydramax,Buyalot
gooeygood
interacts with
ingredient in TastyPill
Medicines
---------
MiracleCure(aaratine,eekamouse)
ExxoPlexx(catboxite,boricium)
Hydramax(drystophan,aaratine,faroutman)
Buyalot(faroutman)
TastyPill(aaratine,drystophan,gooeygood)
Phase 3: Patients
Next, implement the Patient class.
The Patient class should have:
Two instance variables: the patients name (a String), and a list of all of this patients prescriptions (a MedicineList).
This time, there will be no separate PatientList class. Instead, provide class (static) variables that will keep track of all of the Patient objects that have ever been created. Allow for up to 100 Patient objects. Use a simple partially-full array to do this. You may assume that the list will never become full no error checking is needed.
A constructor with a single String parameter giving the patients name.
A method void prescribe(Medicine) which will add a medicine to this patients list of prescriptions.
A standard toString method which will return a String containing the patients name followed by a list of prescriptions, in parentheses. See the sample output below.
A class (static) method void listAllPatients() which will print a list of all of the patients that have been created, using the toString format. (This one can contain a println statement to produce output.)
You can test your class using the supplied test program TestPhase3.java. You should get the same output as you did for Phase 2, plus the following additional output:
Patients
--------
Arthur (ExxoPlexx,MiracleCure,Hydramax)
Betty (TastyPill,MiracleCure)
Chris (Hydramax)
Don (ExxoPlexx,Hydramax,TastyPill)
Eddie (Buyalot,Hydramax,TastyPill)
Fran (ExxoPlexx,Hydramax)
George (ExxoPlexx,Buyalot,TastyPill)
Helen ()
Phase 4: Interactions
Next, implement the Interaction and InteractionList classes, and add additional methods to all of the other classes, to allow a complete search for dangerous medicine interactions.
The Interaction class is a very short class that will allow one single object to store information of the form chemical1 in medicine1 interacts with chemical2 in medicine2. This will permit such information to be sent as a single parameter to methods, or returned as the result of methods. This is a common use for classes and objects. The Interaction class should have:
Instance variables to hold 2 Medicines, and 2 Chemicals contained in them, which have a bad interaction. Make sure you can tell which medicine contains which chemical.
An instance method void setMeds(Medicine, Medicine) which will set the two Medicine fields, and an instance method void setChems(Chemical, Chemical) which will set the two Chemical fields. Make sure that the order is the same so that the first parameter to setChems corresponds to the first parameter to setMeds. It is being done this way because the medicines and chemicals will be found in very different places in your code.
A standard toString method which will produce a String in the format below, which can also be seen in the sample output.
MiracleCure contains eekamouse interacting with faroutman in Hydramax
The InteractionList class should be almost the same as the other two list classes, and should have:
Instance variables that will allow a list of up to 10 Interaction objects to be stored. Use a simple partially-full array to do this. You may assume that the list will never become full no error checking is needed.
A constructor with no parameters that creates an empty list.
An instance method void addInteraction (Interaction) which will add an interaction to the list.
A standard toString method which will return a multi-line String, with each line starting with a tab (\t) character. If the list is empty, the line should be "\tNo Interactions. ". Otherwise it should give one line per interaction in the list, in the format produced by toString. See the sample output below for an example.
Now complete the job by adding various search functions to the other five classes written in Phases 1 to 3.
Add an instance method boolean contains(Chemical) to the ChemicalList class which will determine whether or not that Chemical appears in the list. Look for that specific object. Do not look at the name of the Chemical.
Add an instance method boolean interactsWith(Chemical) to the Chemical class which will determine whether or not this chemical interacts with the one passed as the parameter.
Add an instance method Interaction anyInteraction(ChemicalList) to the ChemicalList class. This will look for any interaction between any chemical in this list with any chemical in the list passed as the parameter. If none are found, it should return null. If any are found, it should return an Interaction object with its two Chemical instance variables set appropriately. There may be multiple interactions between chemicals in the two lists, but you only need to find one of them.
Add an instance method Interaction interactsWith(Medicine) to the Medicine class. It should look for any interaction between this medicine and the medicine passed as a parameter. If it finds one, it should return an Interaction object with all four instance variables set properly. If none are found, it should return null.
Add an instance method InteractionList findInteractions() to the MedicineList class. It should look for any interactions between any two medicines in this list of medicines. Every pair of medicines that interact should appear in the list only once (dont report that A interacts with B and also B interacts with A thats redundant). It should always return an InteractionList, never null. If there are no interactions, the list will simply be an empty list.
Finally, add a class method void drugInteractionReport() to the Patient class. This should look at every patient stored in the system, and check the list of medications prescribed to that patient for possible interactions. It should print a report in the format shown below, using println statements.
You can test your class using the supplied test program TestPhase4.java. You should get the same output as you did for Phase 3, plus the following additional output:
Interaction Report
==================
Patient Arthur:
ExxoPlexx contains catboxite interacting with eekamouse in MiracleCure
ExxoPlexx contains catboxite interacting with drystophan in Hydramax
MiracleCure contains eekamouse interacting with faroutman in Hydramax
Patient Betty:
No interactions.
Patient Chris:
No interactions.
Patient Don:
ExxoPlexx contains catboxite interacting with drystophan in Hydramax
ExxoPlexx contains catboxite interacting with drystophan in TastyPill
Patient Eddie:
No interactions.
Patient Fran:
ExxoPlexx contains catboxite interacting with drystophan in Hydramax
Patient George:
ExxoPlexx contains catboxite interacting with drystophan in TastyPill
Patient Helen:
No interactions.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
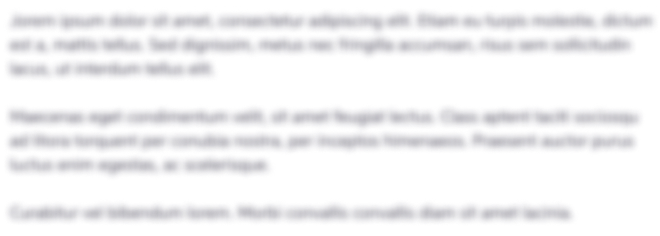
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started