Question
Phase 1: Shifting One Line The 2048 board is a square 2-dimensional grid of tiles. Each place in the grid might be empty, or contain
Phase 1: Shifting One Line The 2048 board is a square 2-dimensional grid of tiles. Each place in the grid might be empty, or contain a tile showing a number that is a power of 2 (2, 4, 8, 16, etc.). In this assignment, a simple int[][] array will be used, with the value 0 representing an empty place. The main operation involved in playing the game is to shift a line of tiles in some direction. In Phase 1, only a 1dimensional line of tiles will be used, and the tiles will only be shifted to the left (from higher index values toward 0). Define a Board2048 class. For now, it should contain only a public static boolean alterOneLine(int[]) method. This method should accept an int[] array representing one line of tiles, and alter the array according to the rules and examples given below. It should return true if the array was changed in any way, and false if it remained exactly the same as it was before. It should handle any size of array. The rules can be implemented and tested one at a time. Rule 1: All the tiles should be shifted to the left as far as they will go. (Empty spaces (0s) do not count.) For example: > int[] test = {0,2,0,0,8,0,4};
> Board2048.alterOneLine(test) true
> test { 2, 8, 4, 0, 0, 0, 0 }
> int[] test = {0,0,0,0,8,2,4};
> Board2048.alterOneLine(test) true
> test { 8, 2, 4, 0, 0, 0, 0 }
> int[] test = {2,4,2,0,0,0,0};
> Board2048.alterOneLine(test) false (the line did not change)
> test { 2, 4, 2, 0, 0, 0, 0 } Rule 2: If two tiles containing the same number are shifted into adjacent positions, they are merged into a single tile with double that value. > int[] test = {2,0,2,4,0,0,4};
> Board2048.alterOneLine(test) true
> test { 4, 8, 0, 0, 0, 0, 0 } (the two 2s merged, and the two 4s merged)
> int[] test = {2,0,4,2,0,0,4};
> Board2048.alterOneLine(test) true
> test { 2, 4, 2, 4, 0, 0, 0 } (nothing merged the 2s are not adjacent, nor are the 4s) Rule 3: If a new tile is created by merging two other tiles (Rule 2) then it is locked and may not be changed again in this shift operation. (But it may be changed in future calls to alterOneLine.) Pairs of tiles must always be merged from left to right. > int[] test = {2,0,2,2,0};
> Board2048.alterOneLine(test) true
> test { 4, 2, 0, 0, 0 } (The first pair of 2s on the left merge, not the last pair.)
> int[] test = {2,0,2,2,0,2};
> Board2048.alterOneLine(test) true
> test { 4, 4, 0, 0, 0, 0 } (Two pairs of 2s merge, but the 4s are locked and do not merge.)
> int[] test = {2,0,2,4,0,8};
> Board2048.alterOneLine(test) true
> test { 4, 4, 8, 0, 0, 0 } (The 2s merge but the new 4 that results is locked.)
> Board2048.alterOneLine(test) true
> test { 8, 8, 0, 0, 0, 0 } (Another shift of the same array will merge the 4s.)
> Board2048.alterOneLine(test) true
> test { 16, 0, 0, 0, 0, 0 } (A third shift of the same array will merge the 8s.)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
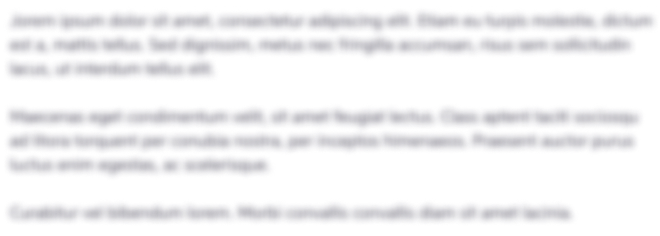
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started