Question
Phone,type,area code,prefix,suffix (3) the class must be able to read a file (ask the user for the name of the file) and somehow return an
Phone,type,area code,prefix,suffix
(3) the class must be able to read a file (ask the user for the name of the file) and somehow return an ArrayList. This ArrayList can then be passed to your Database class and used with the methods there.The I/O class must also be able to take your ArrayList of Person objects and write all of the data to a NEW output file.
4.ystem to your database program which allows the user to test all functionality from the first program, i.e. menu options for all the things you were supposed to test in Homework 05. In addition to being able to save / load information to / from a chosen input file and chosen output file. Add an option to also be able to create a new person for the database. The person could be any of the person types from the previous assignment. This new person should be added to the ArrayList of people.
The requirement is that I have to (1) have a menu system method in database class for the user
2have a class that provides a way to read and write data from / to a file and populate an ArrayList of Person objects or save the data of an ArrayList of objects. Build a new class which uses Text I/O to read text from a .csv (ObjectType,first-name,last-name, . all the rest of the information
Can someone check the code that I have so far, homework 5 is basically creating the database system that has several classes inheriting from the main Person class, and it will count how many people are in the system and how many students/how many staff/how many employees etc. and this assignment is an add up to hmk 5, so far I have the code as below. I am stuck on creating the menu to let user create the new person and store it to the arraylist so that it can access the methods in database class, and also the reading of the file, I always got an IndexOutofBound exception and I dont know how to solve it..
//Person class
import java.util.ArrayList;
public class Person {
private String firstname;
private String lastname;
private String emailaddress;
private Address address;
private ArrayList number;
public Person() {}
public String getFirstname() {
return firstname;
}
public void setFirstname(String firstname) {
this.firstname = firstname;
}
public String getLastname() {
return lastname;
}
public void setLastname(String lastname) {
this.lastname = lastname;
}
public String getEmailaddress() {
return emailaddress;
}
public void setEmailaddress(String emailaddress) {
this.emailaddress = emailaddress;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public ArrayList getNumber() {
return number;
}
public void setNumber(ArrayList number) {
this.number = number;
}
public String toString() {
String s;
s= "Name: " + firstname + " " +lastname + " " + address + " Email: " + emailaddress + " "+"Phone: ";
for(int i = 0; i
PhoneNumber num = number.get(i);
s += num +" ";
}
return s;
}}
//Address class to display address:
public class Address {
private int streetNumber;
private String optionalApartmentNumber;
private String streetName;
private String city;
private String state;
private int zipCode;
public int getStreetNumber() {
return streetNumber;
}
public void setStreetNumber(int streetNumber) {
this.streetNumber = streetNumber;
}
public String getOptionalApartmentNumber() {
return optionalApartmentNumber;
}
public void setOptionalApartmentNumber(String n) {
this.optionalApartmentNumber = n;}
public String getStreetName() {
return streetName;
}
public void setStreetName(String streetName) {
this.streetName = streetName;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public int getZipCode() {
return zipCode;
}
public void setZipCode(int zipCode) {
this.zipCode = zipCode;
}
public String toString() {
String s5="";
if(optionalApartmentNumber!=null && optionalApartmentNumber !="-1") {
s5 = "Address: " + streetNumber + " " + streetName + " "+
" " + optionalApartmentNumber + " " + city + ", " + state + ", " + zipCode;
return s5;
}
else if(optionalApartmentNumber == "-1") {
s5 = "Address: " + streetNumber + " " + streetName + " "+
" " + city + ", " + state + ", " + zipCode;
return s5;
}
return s5;
}}
//PhoneNumber class to display home numbers:
public class PhoneNumber {
private String type;
private int areaCode;
private int prefix;
private int suffix;
public PhoneNumber() {}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public int getAreaCode() {
return areaCode;
}
public void setAreaCode(int areaCode) {
this.areaCode = areaCode;
}
public int getPrefix() {
return prefix;
}
public void setPrefix(int prefix) {
this.prefix = prefix;
}
public int getSuffix() {
return suffix;
}
public void setSuffix(int suffix) {
this.suffix = suffix;
}
public String toString() {
String s1;
s1 = "" + type + ": " + " (" + areaCode + ") " + prefix + " - " + suffix;
return s1;
}
}
//Employee class that extends from Person class
public class Employee extends Person {
private String location;
private double salary;
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public String toString() {
String s2 = super.toString();
s2 = s2 + "Office Location: " + location + " " +
"Salary: " + salary + " ";
return s2;
}
}
//Faculty class that extends from Employee class
public class Faculty extends Employee {
String officeHours;
String rank;
public String getOfficeHours() {
return officeHours;
}
public void setOfficeHours(String officeHours) {
this.officeHours = officeHours;
}
public String getRank() {
return rank;
}
public void setRank(String rank) {
this.rank = rank;
}
@Override
public String toString() {
String s3;
s3 = super.toString();
s3 += "Office Hours: " + officeHours + " " +
"Rank: " + rank + " ";
return s3;
}}
//Staff class that extends Employee class:
public class Staff extends Employee{
String jobTitle;
public String getJobTitle() {
return jobTitle;
}
public void setJobTitle(String jobTitle) {
this.jobTitle = jobTitle;
}
@Override
public String toString() {
String s4;
s4 = super.toString();
s4+= "Job Title: " + jobTitle + " ";
return s4;
}}
//Student class that extends Person class:
public class Student extends Person {
String classStanding;
public String getClassStanding() {
return classStanding;
}
public void setClassStanding(String classStanding) {
this.classStanding = classStanding;
}
@Override
public String toString() {
String string = super.toString();
string += "Class Standing: " + classStanding + " ";
return string;
}}
//database class that displays the # of people # of students etc...:
import java.util.ArrayList;
import java.util.Scanner;
import hw1_and_hw2.NPC;
public class Database {
private static ArrayList per = new ArrayList();
public Database(ArrayList per) {
this.per = per;
}
public static void printDatabase(){
for(int i =0; i
Person person = per.get(i);
System.out.println("(" +(i+1)+")." +" " +person);
}
}
public static void printDatabase(String occupation) {
for(int i =0; i
Person person = per.get(i);
//person.getClass() method returns class object of person(which is Person).
//getSimpleName() method returns the name of class such as Student,Faculty, Employee, and Staff.
if(person.getClass().getSimpleName().equalsIgnoreCase(occupation)) {
System.out.println(person);
}
}
}
public static int getNumberOfPeople() {
return per.size();
}
public static int getNumberOfStudents() {
int count = 0;
for(int i =0; i
Person person = per.get(i);
if(person.getClass().getSimpleName().equalsIgnoreCase("Student")) {
count++;
}
}
return count;
}
public static int getNumberOfEmployees() {
int count = 0;
for(int i =0; i
Person person = per.get(i);
if(person.getClass().getSimpleName().equalsIgnoreCase("employee")) {
count++;
}
}
return count;
}
public static int getNumberOfStaff() {
int count = 0;
for(int i =0; i
Person person = per.get(i);
if(person.getClass().getSimpleName().equalsIgnoreCase("staff")) {
count++;
}
}
return count;
}
public static int getNumberOfFaculty() {
int count = 0;
for(int i =0; i
Person person = per.get(i);
if(person.getClass().getSimpleName().equalsIgnoreCase("faculty")) {
count++;
}
}
return count;
}
public static int getNumberOfStudentsByClassStanding(String standing) {
int count = 0;
for(int i =0; i
Person person = per.get(i);
if(person.getClass().getSimpleName().equalsIgnoreCase("student")) {
//casting person to refer to the Student class and thus access the methods in that class.
if(((Student) person).getClassStanding().equalsIgnoreCase(standing)) {
count++;
}
}}
return count;
}
public static void displayEmployeesGreaterThanSalary(double salary ) {
for(int i =0; i
Person person = per.get(i);
//check whether the object person is an instance of the Employee class.
if(person instanceof Employee) {
//Casting the person object to enable it to access getSalary method in Employee class.
if(((Employee) person).getSalary()>salary) {
System.out.println(person);
}
}
}
}
public static void displayEmployeesEqualToSalary(double salary) {
for(int i =0; i
Person person = per.get(i);
if(person instanceof Employee) {
if(((Employee) person).getSalary() == salary) {
System.out.println(person);
}
}
}
}
public static void displayEmployeesLessThanSalary(double salary) {
for(int i =0; i
Person person = per.get(i);
if(person instanceof Employee) {
if(((Employee) person).getSalary()
System.out.println(person);
}
}
}
}
public ArrayList getPer() {
return per;
}
public void setPer(ArrayList per) {
this.per = per;
}
public static void Text() {
Scanner hi = new Scanner(System.in);
String hello = hi.nextLine();
UseTextIO.readFile(hello);
ArrayList persons=UseTextIO.createObjects();
// for (Person person : persons) {
// if(person!=null)
// System.out.println(person.toString()+" ");}
//
//
for(int i = 0;i
System.out.println(persons.get(i) + " ");
}
}
public static ArrayList createPerson(ArrayList list) {
Scanner sc = new Scanner(System.in);
Scanner sc1 = new Scanner(System.in);
String firstname;
String lastname;
String emailaddress;
int prefix;
int suffix;
int areaCode;
// ArrayList number;
System.out.println("Please enter the position of the person: ");
if(sc.nextLine().equalsIgnoreCase("student")) {
Student students = new Student();
System.out.println("Please enter the First Name: ");
students.setFirstname(sc.nextLine());
System.out.println("Please enter the last name: ");
students.setLastname(sc.nextLine());
System.out.println("Please enter the Email Address: ");
emailaddress = sc.nextLine();
students.setEmailaddress(emailaddress);
System.out.println("Please enter the class standing: ");
students.setClassStanding(sc.nextLine());
PhoneNumber phone = new PhoneNumber();
System.out.println("Please enter the Phone Type: ");
phone.setType(sc.nextLine());
System.out.println("Please enter the Area Code: ");
phone.setAreaCode(sc.nextInt());
System.out.println("Please enter the Prefix: ");
phone.setPrefix(sc.nextInt());;
System.out.println("Please enter the Suffix: ");
phone.setSuffix(sc.nextInt());
ArrayList ph = new ArrayList();
ph.add(phone);
students.setNumber(ph);
Address address = new Address();
System.out.println("Please enter the street number: ");
address.setStreetNumber(sc.nextInt());
System.out.println("Please enter the street name: ");
address.setStreetName(sc1.nextLine());
System.out.println("Please enter the city: ");
address.setCity(sc1.nextLine());
System.out.println("Please enter the state: ");
address.setState(sc.nextLine());
System.out.println("Please enter the zip code: ");
address.setZipCode(sc.nextInt());
students.setAddress(address);
list.add(students);
}
if(sc.nextLine().equalsIgnoreCase("staff")) {
Staff staff = new Staff();
System.out.println("Please enter the First Name: ");
firstname = sc.nextLine();
staff.setFirstname(firstname);
System.out.println("Please enter the Last Name: ");
lastname = sc.nextLine();
staff.setLastname(lastname);
System.out.println("Please enter the Email Address: ");
emailaddress = sc.nextLine();
staff.setEmailaddress(emailaddress);
System.out.println("Please enter the salary: ");
staff.setSalary(sc.nextDouble());
System.out.println("Please enter the job title: ");
staff.setJobTitle(sc.nextLine());
PhoneNumber phone1 = new PhoneNumber();
System.out.println("Please enter the Phone Type: ");
phone1.setType(sc.nextLine());
System.out.println("Please enter the Area Code: ");
phone1.setAreaCode(sc.nextInt());
System.out.println("Please enter the Prefix: ");
phone1.setPrefix(sc.nextInt());;
System.out.println("Please enter the Suffix: ");
phone1.setSuffix(sc.nextInt());
ArrayList ph1 = new ArrayList();
ph1.add(phone1);
staff.setNumber(ph1);
Address address1 = new Address();
System.out.println("Please enter the street number: ");
address1.setStreetNumber(sc.nextInt());
System.out.println("Please enter the street name: ");
address1.setStreetName(sc.nextLine());
System.out.println("Please enter the city: ");
address1.setCity(sc.nextLine());
System.out.println("Please enter the state: ");
address1.setState(sc.nextLine());
System.out.println("Please enter the zip code: ");
address1.setZipCode(sc.nextInt());
staff.setAddress(address1);
list.add(staff);
}
// System.out.println("Please enter the First Name: ");
// firstname = sc.nextLine();
// System.out.println("Please enter the Last Name: ");
// lastname = sc.nextLine();
// System.out.println("Please enter the Email Address: ");
// emailaddress = sc.nextLine();
// System.out.println("");
// PhoneNumber phone = new PhoneNumber();
// System.out.println("Please enter the Phone Type: ");
// phone.setType(sc.nextLine());
// System.out.println("Please enter the Area Code: ");
// phone.setAreaCode(sc.nextInt());
// System.out.println("Please enter the Prefix: ");
// phone.setPrefix(sc.nextInt());;
// System.out.println("Please enter the Suffix: ");
// phone.setSuffix(sc.nextInt());
// number.add(phone);
// System.out.println("Please enter the street number: ");
// address1.setStreetNumber(sc.nextInt());
// System.out.println("Please enter the street name: ");
// address1.setStreetName(sc.nextLine());
// System.out.println("Please enter the city: ");
// address1.setCity(sc.nextLine());
// System.out.println("Please enter the state: ");
// address1.setState(sc.nextLine());
// System.out.println("Please enter the zip code: ");
// address1.setZipCode(sc.nextInt());
return list;
}
public static void MenuSystem() {
Scanner in = new Scanner(System.in);
while(true) {
System.out.println("Menu:");
System.out.println("1.Displaying all the people.");
System.out.println("2.Displaying all the staff members.");
System.out.println("3.Displaying all the students.");
System.out.println("4.Displaying all the facultys.");
System.out.println("5.Displaying all the employees.");
System.out.println("6.Displaying all members with salary equal to 4000");
System.out.println("7.Displaying all members with salary less than 4000");
System.out.println("8.Displaying all members with salary greater than 4000:");
System.out.println("9.Displaying total number of people.");
System.out.println("10.Displaying number of employees.");
System.out.println("11.Displaying number of faculty.");
System.out.println("12.Displaying number of staff.");
System.out.println("13.Displaying nmber of students.");
System.out.println("14.Displaying number of freshman.");
System.out.println("15.Displaying number of sophomore");
System.out.println("16.Displaying number of Junior");
System.out.println("17.Displaying number of Senior");
System.out.println("18.Read the File. What file do you want to read?");
System.out.println("19.Exit.");
System.out.println("20.Create a new person");
int input = in.nextInt();
if(input == 1) {
printDatabase();
}
else if(input == 2) {
printDatabase("staff");
}
else if(input == 3) {
printDatabase("student");
}
else if(input == 4) {
printDatabase("faculty");
}
else if(input ==5) {
printDatabase("employee");
}
else if(input == 6) {
displayEmployeesEqualToSalary(4000);
}
else if(input == 7) {
displayEmployeesLessThanSalary(4000);
}
else if(input == 8) {
displayEmployeesGreaterThanSalary(4000);
}
else if(input == 9) {
System.out.println("The Number Of People: " + per.size());
}
else if(input==10) {
System.out.println("The Number Of Employees:: " + getNumberOfEmployees());
}
else if(input == 11) {
System.out.println("The Number Of faculty: " + getNumberOfFaculty());
}
else if(input ==12) {
System.out.println("The Number Of staff: "+getNumberOfStaff());
}
else if(input == 13) {
System.out.println("The Number Of students:" + getNumberOfStudents());
}
else if(input == 14) {
System.out.println("The Number Of freshman:" + getNumberOfStudentsByClassStanding("freshman"));
}
else if(input == 15) {
System.out.println("The Number Of sophmore:" + getNumberOfStudentsByClassStanding("sophmore"));
}
else if(input == 16) {
System.out.println("The Number Of junior:" + getNumberOfStudentsByClassStanding("Junior"));
}
else if(input == 17) {
System.out.println("The Number Of senior:" + getNumberOfStudentsByClassStanding("senior"));
}
else if(input == 18) {
System.out.println("Please Enter the File Name: ");
Text();
}
else if(input == 19) {
System.out.println("Goodbye~");
System.exit(0);
}
else if(input == 20) {
ArrayList newperson = new ArrayList();
createPerson(newperson);
}
else {
System.out.println("Error");
}
}}}
and also the textIO class that reads the csv file :
import java.io.File;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
//import java.nio.file.Files;
import java.util.ArrayList;
import java.util.Scanner;
public class UseTextIO {
static ArrayList data = new ArrayList();
// Read the file
public static void readFile(String filename) {
File in = new File("resources/data.csv");
try {
Scanner s = new Scanner(in);
while (s.hasNext()){
data.add(s.next());}
s.close();
System.out.println(data.toString().replace("[", "").replace("]",""));
}
catch (IOException e) {
e.printStackTrace();}
}
public static ArrayList createObjects() {
ArrayList persons = new ArrayList();
// first get the whole data
// parse data to objects and add to list of people
// first we need to identify object type and then create object accordingly
int index = 0;
do {
String string = data.get(index);
if (string.contains("Student")) {
// create a student object
// read first line and split using ,
String arr[] = string.split(",");
Student student = new Student();
student.setFirstname(arr[1]);
student.setLastname(arr[2]);
// create address object
Address add = new Address();
try {
add.setStreetNumber(Integer.parseInt(arr[3]));
add.setOptionalApartmentNumber(arr[4]);
add.setStreetName(arr[5]);
add.setState(arr[6]);
add.setCity(arr[7]);
add.setZipCode(Integer.parseInt(arr[8]));
student.setEmailaddress(arr[9]);
student.setClassStanding(arr[10]);
student.setAddress(add);}catch(IndexOutOfBoundsException ex) {
System.out.println("Too many");
}
// create phone objects
String s = null;
index++;
ArrayList numbers = new ArrayList();
do {
s = data.get(index);
if ((s.contains("Staff") || s.contains("Faculty") || s.contains("Student"))) {
break;
}
String array[] = s.split(",");
PhoneNumber phone = new PhoneNumber();
phone.setAreaCode(Integer.parseInt(array[2]));
phone.setType(array[1]);
phone.setPrefix(Integer.parseInt(array[3]));
phone.setSuffix(Integer.parseInt(array[4]));
numbers.add(phone);
index++;
} while (data.size() >index);
student.setNumber(numbers);
persons.add(student);
} else if (string.contains("Faculty")) {
// create a student object
// read first line and split using ,
String arr[] = string.split(",");
Faculty faculty = new Faculty();
faculty.setFirstname(arr[1]);
faculty.setLastname(arr[2]);
// create address object
Address add = new Address();
add.setStreetNumber(Integer.parseInt(arr[3]));
add.setOptionalApartmentNumber(arr[4]);
add.setStreetName(arr[5]);
add.setState(arr[6]);
add.setCity(arr[7]);
add.setZipCode(Integer.parseInt(arr[8]));
faculty.setEmailaddress(arr[9]);
faculty.setLocation(arr[10]);
faculty.setSalary(Double.parseDouble(arr[11]));
faculty.setOfficeHours(arr[12]);
faculty.setRank(arr[13]);
faculty.setAddress(add);
// create phone objects
String s = null;
index++;
ArrayList numbers = new ArrayList();
do {
s = data.get(index);
if ((s.contains("Staff") || s.contains("Faculty") || s.contains("Student"))) {
break;
}
String array[] = s.split(",");
PhoneNumber phone = new PhoneNumber();
phone.setAreaCode(Integer.parseInt(array[2]));
phone.setType(array[1]);
phone.setPrefix(Integer.parseInt(array[3]));
phone.setSuffix(Integer.parseInt(array[4]));
numbers.add(phone);
index++;
} while (data.size() >index);
faculty.setNumber(numbers);
persons.add(faculty);
} else if (string.contains("Staff")) {
// create a student object
// read first line and split using ,
String arr[] = string.split(",");
Staff staff = new Staff();
staff.setFirstname(arr[1]);
staff.setLastname(arr[2]);
// create address object
Address add = new Address();
add.setStreetNumber(Integer.parseInt(arr[3]));
add.setOptionalApartmentNumber(arr[4]);
add.setStreetName(arr[5]);
add.setState(arr[6]);
add.setCity(arr[7]);
add.setZipCode(Integer.parseInt(arr[8]));
staff.setEmailaddress(arr[9]);
staff.setLocation(arr[10]);
staff.setSalary(Double.parseDouble(arr[11]));
staff.setJobTitle(arr[12]);
staff.setAddress(add);
// create phone objects
String s = null;
index++;
ArrayList numbers = new ArrayList();
do {
s = data.get(index);
if ((s.contains("Staff") || s.contains("Faculty") || s.contains("Student"))) {
break;
}
String array[] = s.split(",");
PhoneNumber phone = new PhoneNumber();
phone.setAreaCode(Integer.parseInt(array[2]));
phone.setType(array[1]);
phone.setPrefix(Integer.parseInt(array[3]));
phone.setSuffix(Integer.parseInt(array[4]));
numbers.add(phone);
index++;
} while (data.size() >index);
staff.setNumber(numbers);
persons.add(staff);
}
} while (data.size() >index);
return persons;}}
//Finally I have my test class to test out:
import java.util.ArrayList;
import java.util.Scanner;
public class Testrun {
public static void main(String[] args) {
ArrayList listOfPeople=new ArrayList();
Database database=new Database(listOfPeople);
Scanner in = new Scanner(System.in);
Student student1=new Student();
student1.setFirstname("Stephnie");
student1.setLastname("Khan");
student1.setEmailaddress("SteKhan@mail.com");
student1.setClassStanding("freshman");
PhoneNumber ph_one=new PhoneNumber();
ph_one.setAreaCode(626);
ph_one.setType("Home");
ph_one.setPrefix(322);
ph_one.setSuffix(6532);
PhoneNumber ph_two=new PhoneNumber();
ph_two.setAreaCode(815);
ph_two.setType("Mobile");
ph_two.setPrefix(774);
ph_two.setSuffix(1212);
ArrayList phones=new ArrayList();
phones.add(ph_one);
phones.add(ph_two);
student1.setNumber(phones);
Student student2=new Student();
student2.setFirstname("Ken");
student2.setLastname("Chen");
student2.setEmailaddress("kman@mail.com");
student2.setClassStanding("junior");
PhoneNumber ph_three=new PhoneNumber();
ph_three.setAreaCode(626);
ph_three.setType("Mobile");
ph_three.setPrefix(789);
ph_three.setSuffix(2345);
ArrayList phones2=new ArrayList();
phones2.add(ph_three);
student2.setNumber(phones2);
Staff staff1=new Staff();
staff1.setFirstname("Jennifer");
staff1.setLastname("Mach");
staff1.setEmailaddress("jennychinchin@mail.com");
staff1.setSalary(5000);
staff1.setJobTitle("Vice President");
PhoneNumber sph_one=new PhoneNumber();
sph_one.setAreaCode(626);
sph_one.setType("Mobile");
sph_one.setPrefix(757);
sph_one.setSuffix(2223);
PhoneNumber sph_two=new PhoneNumber();
sph_two.setAreaCode(626);
sph_two.setType("Mobile");
sph_two.setPrefix(663);
sph_two.setSuffix(3222);
ArrayList sphones=new ArrayList();
sphones.add(sph_one);
sphones.add(sph_two);
staff1.setNumber(sphones);
Staff staff2=new Staff();
staff2.setFirstname("Loren");
staff2.setLastname("Chen");
staff2.setEmailaddress("Lchen@mail.com");
staff2.setSalary(6000);
staff2.setJobTitle("Administrator");
PhoneNumber s2ph_one=new PhoneNumber();
s2ph_one.setAreaCode(583);
s2ph_one.setType("Home");
s2ph_one.setPrefix(202);
s2ph_one.setSuffix(314);
PhoneNumber s2ph_two=new PhoneNumber();
s2ph_two.setAreaCode(111);
s2ph_two.setType("Mobile");
s2ph_two.setPrefix(334);
s2ph_two.setSuffix(334);
ArrayList s2phones=new ArrayList();
s2phones.add(s2ph_one);
s2phones.add(s2ph_two);
staff2.setNumber(s2phones);
Faculty faculty = new Faculty();
faculty.setFirstname("Zac");
faculty.setLastname("You");
faculty.setEmailaddress("zac1@csula.com");
faculty.setSalary(4000);
faculty.setRank("#11");
faculty.setOfficeHours("M,W,F, 1:50PM-3:05PM");
faculty.setLocation("CSULA");
PhoneNumber ph_4=new PhoneNumber();
ph_4.setAreaCode(345);
ph_4.setType("Mobile");
ph_4.setPrefix(111);
ph_4.setSuffix(111);
ArrayList phones41=new ArrayList();
phones41.add(ph_4);
faculty.setNumber(phones41);
Employee emp = new Employee();
emp.setFirstname("Keenan");
emp.setLastname("Wang");
emp.setEmailaddress("Knnena@gamil.com");
emp.setSalary(3000.0);
emp.setLocation("UCLA");
PhoneNumber ph_5=new PhoneNumber();
ph_5.setAreaCode(626);
ph_5.setType("Mobile");
ph_5.setPrefix(123);
ph_5.setSuffix(111);
ArrayList phones4=new ArrayList();
PhoneNumber ph_6 = new PhoneNumber();
ph_6.setAreaCode(728);
ph_6.setPrefix(111);
ph_6.setSuffix(112);
ph_6.setType("Home");
phones4.add(ph_5);
phones4.add(ph_6);
emp.setNumber(phones4);
Address ad1=new Address();
ad1.setStreetNumber(112);
ad1.setCity("Las Vegas");
ad1.setState("NV");
ad1.setOptionalApartmentNumber("A1333");
ad1.setStreetName("Washington Ave");
ad1.setZipCode(644443);
Address ad2=new Address();
ad2.setStreetNumber(999);
ad2.setCity("Los Angeles");
ad2.setState("CA");
ad2.setOptionalApartmentNumber("777");
ad2.setStreetName("Peck Road");
ad2.setZipCode(91755);
Address ad3=new Address();
ad3.setStreetNumber(555);
ad3.setCity("Monterey Park");
ad3.setState("CA");
ad3.setOptionalApartmentNumber("D");
ad3.setStreetName("Alhambra Ave");
ad3.setZipCode(91755);
Address ad4=new Address();
ad4.setStreetNumber(673);
ad4.setCity("Hollywood");
ad4.setState("CA");
ad4.setOptionalApartmentNumber("Z12");
ad4.setStreetName("Hollywood Dr.");
ad4.setZipCode(90076);
Address ad5 = new Address();
ad5.setStreetNumber(14209);
ad5.setCity("Baldwin Park");
ad5.setStreetName("Riverside St.");
ad5.setZipCode(91706);
ad5.setState("CA");
student1.setAddress(ad1);
student2.setAddress(ad2);
staff1.setAddress(ad3);
staff2.setAddress(ad4);
faculty.setAddress(ad5);
emp.setAddress(ad5);
listOfPeople.add(student1);
listOfPeople.add(student2);
listOfPeople.add(staff1);
listOfPeople.add(staff2);
listOfPeople.add(faculty);
listOfPeople.add(emp);
Database.MenuSystem();
Database.Text();
}
}
I know this is a lot but I am totally stuck on this assignment, please help.
Overview: For this program you will be adding a new class to Homework 05. This class will provide a way to read and write data from / to a file and populate an ArrayList of Person objects or save the data of an ArrayList of objects. Build a new class which uses Text I/O to read text from a.csv (comma separated value) file. The text file will have values in the following format: ObjectType,first-name, 1ast-name, all the rest of the information Phone, type, area code,prefix, suffix A person could have any number of phone numbers listed after their information. There are no spaces between the entries, and no spaces before or after a comma. I am leaving this assignment fairly vague because I want you to design the class in your own way. The only requirement is that the class must be able to read a file (ask the user for the name of the file) and somehow return an ArrayList. This ArrayList can then be passed to your Database class and used with the methods there. The I/O class must also be able to take your ArrayList of Person objects and write all of the data to a NEW output file. Example File: Overview: For this program you will be adding a new class to Homework 05. This class will provide a way to read and write data from / to a file and populate an ArrayList of Person objects or save the data of an ArrayList of objects. Build a new class which uses Text I/O to read text from a.csv (comma separated value) file. The text file will have values in the following format: ObjectType,first-name, 1ast-name, all the rest of the information Phone, type, area code,prefix, suffix A person could have any number of phone numbers listed after their information. There are no spaces between the entries, and no spaces before or after a comma. I am leaving this assignment fairly vague because I want you to design the class in your own way. The only requirement is that the class must be able to read a file (ask the user for the name of the file) and somehow return an ArrayList. This ArrayList can then be passed to your Database class and used with the methods there. The I/O class must also be able to take your ArrayList of Person objects and write all of the data to a NEW output file. Example FileStep by Step Solution
There are 3 Steps involved in it
Step: 1
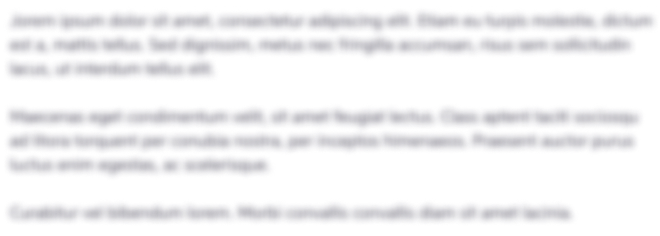
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started