Question
Please add Commenting (commented each line of the code and used proper variable naming conventions which are self explanatory.) on this Square, will implement a
Please add Commenting (commented each line of the code and used proper variable naming conventions which are self explanatory.) on this
Square, will implement a data type Square that represents squares with the x and y coordinates of their upper-left corners and the length.
The API should be as follows.
Square(double x, double y, double length) //constructor double area() //returns the area of the square double perimeter() //returns the perimeter of the square boolean intersects(Square b) //does this square intersect b? Two squares would intersect if they share one or more common points boolean contains(Square b) //does this square contain b? void draw() //draw this square on standard drawing
Code:
import java.util.Scanner;
public class Square {
private double x;
private double y;
private double length;
// Getters and Setters for the private members
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
//Constructor
public Square(double x, double y, double length) {
this.x = x;
this.y = y;
this.length = length;
}
//To calculate are of square
public double area() {
return this.length * this.length;
}
//To calculate perimeter of Square
public double perimeter() {
return 4 * length;
}
// To check if two squares intersect
public boolean intersect( Square s)
{
double p1X = this.x;
double p1Y = this.y;
double p2X = this.x + this.length;
double p2Y = this.y - this.length;
// If one rectangle is on left side of other
if (p1X > (s.getX() + s.getLength()) || s.getX() > p2X)
return false;
// If one rectangle is above other
if (p1Y < (s.getY() - s.getLength()) || s.getY() < p2Y)
return false;
return true;
}
//To check if second square is inside another
public boolean contains(Square s) {
double p1X = this.x;
double p1Y = this.y;
double p2X = this.x + this.length;
double p2Y = this.y - this.length;
double s1X = s.getX();
double s1Y = s.getY();
double s2X = s.getX() + s.getLength();
double s2Y = s.getY() - s.getLength();
if(s1X < p1X && s1Y > p1Y && s2X > p2X && s2Y < p2Y)
return true;
return false;
}
public static void main(String args[]) {
Square s = new Square (0.2,0.7,0.3);
System.out.println("The area is : " + s.area());
System.out.println("The perimeter is : " + s.perimeter());
System.out.println("Enter the upper-left coordinates and the length of a square:");
Scanner scanner = new Scanner(System.in);
String[] inputs = scanner.nextLine().split(" ");
double a = Double.parseDouble(inputs[0]);
double b = Double.parseDouble(inputs[1]);
double c = Double.parseDouble(inputs[2]);
Square sq = new Square (a,b,c);
if(s.intersect(sq) == true)
System.out.println("It intersects the first square");
else
System.out.println("It doesnot intersects the first square");
if(s.contains(sq) == true)
System.out.println("It contains the first square");
else
System.out.println("It doesnot contain the first square");
scanner.close();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
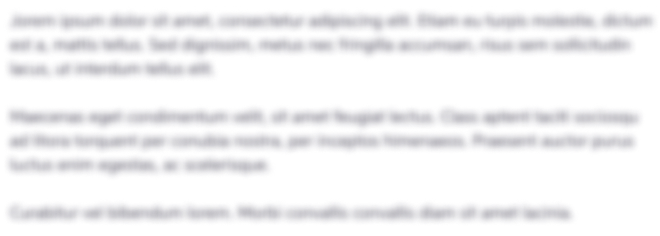
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started