Question
Please answer both requirements on each file correctly. Do not post the same code already answered on here by others. I need a different code!!!
Please answer both requirements on each file correctly. Do not post the same code already answered on here by others. I need a different code!!!
C# programming:
Complete the Tic-Tac-Toe game:
Download the game template TicTacToe.zip.
Add Exception throwing to the .Move method as described in the TBD comment
.
Add Exception handling to the Main() method as described in the TBD comment.
Complete the Ended method. The game is over when the grid is full or one player has 3 in a row. Humans are very good at recognizing patterns and can see 3 in a row quite easily. Think about how you would write down a step by step process of detecting this and then implement it. You may discuss your ideas in the forums but do not share code.
Code so far:
Game.cs:
using System; using System.Security.Cryptography.X509Certificates;
namespace TicTacToe { internal class Game { char[,] grid = new char[3,3]; public Game() { for (int x = 0; x < 3; x++) { for (int y = 0; y < 3; y++) { grid[x, y] = '.'; } } }
public bool Ended() { //TBD: Determine the 'end' condition return false; }
internal void Move(int position, char player) { //TBD: This function needs validation //1. Position needs to be in the range 0..9 - if not throw an ArgumentException //2. The grid cell needs to be unoccupied - if not throw a BadMoveException // .Net does not have a BadMoveException so you must define a custom exception class
grid[position % 3, (position /3)] = player; }
public void Print() { Console.Clear();
Console.WriteLine("Key"); int cell = 0; for (int y = 0; y < 3; y++) { if (y >= 1) Console.WriteLine("---+---+---"); for (int x = 0; x < 3; x++) { if (x >= 1) Console.Write("|"); Console.Write(" {0} ", cell++); } Console.WriteLine(); }
Console.WriteLine(); for (int y = 0; y < 3; y++) { if (y >= 1) Console.WriteLine("---+---+---"); for (int x = 0; x < 3; x++) { if (x >= 1) Console.Write("|"); Console.Write(" {0} ", grid[x,y]); } Console.WriteLine(); }
}
} }
Program.cs:
using System;
namespace TicTacToe { class Program { static void Main() { var game = new Game(); var player = 'X'; while (!game.Ended()) { game.Print();
//Get an integer position Console.Write("Enter your move:"); string input; int position; do { input = Console.ReadLine(); } while (!int.TryParse(input, out position));
//TBD: Handle the Exceptions: //1. Once you have implemented exceptions in game.Move() you must catch the ones you expect to see here //and handle them with suitable error messages //2. Also handle any unexpected exceptions with an "Unknown Error" message. Work out a way to test that code! game.Move(position, player);
//Switch players player = (player == 'X') ? 'O' : 'X'; } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
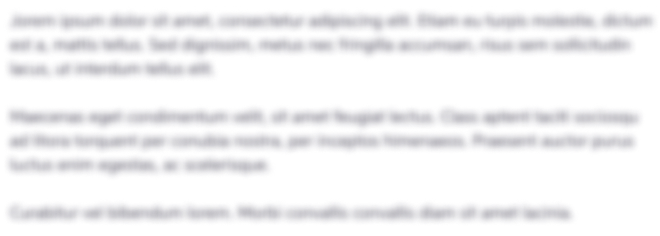
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started