Question
Please answer the question correctly and show the correct code and show that it runs. Thank you. IN C PROGRAM Make files (about 45-60 minutes)
Please answer the question correctly and show the correct code and show that it runs. Thank you. IN C PROGRAM
Make files (about 45-60 minutes) Purpose: Creating a make file Files: Download the tutorial tar file t9_make.tar and extract the files into the tutorial directory. As programs get larger and include multiple files, the management of the files and the executable is getting harder. Often it is hard to keep track of all the files that were changed. As a result if changed files are not compiled and relinked then the changes are not included in the executable. One option is to recompile and link all the files. However, this may time consuming. For example, if a program includes 50 *.c files and each file compilation takes 3 seconds then one has to wait 150 seconds or about 3 minutes before one can test the program again. Unix has a utility that assists in the management of the files the make utility. The make utility is designed to help determine which files should be recompiled and relinked into the program. The make utility reads an input file, which by default is named Makefile, to manage the code. The purpose of the Makefile is to maintain the decency between files. For example, if FileA depends on FileB then whenever a change occurs in FileB then FileA must be recompiled. For example, file main_test.c contains test program for a linked list and file linked_list.c contains the linked list functions. In this case if there are changes to the linked list code then the file main_test.c may need to be recompiled and linked with the updated linked_list.c file. 2 The dependencies are listed in the make file as follows: File name: list of dependencies In the above example the dependency will appear in the file as FileA : FileB FileA, which appear before the : is termed the target file and FileB is termed the dependency file list. Next, one needs to determine the action that must be taken when a change has occurred in one of the files (e.g., FileB above) The action is written below the list of dependencies. For example if FileB has changed then a new executable is created using gcc o FileA FileB This will appear in the file as follows: FileA : FileB
PERSON.H
#ifndef HEADER_PERSON #define HEADER_PERSON
#define NAME_LENGTH 16 #define TWO_NAME_LENGTH 32 #define NUM_YEARS 5
struct person { unsigned int id; unsigned short age; float salary[NUM_YEARS]; char first_name[NAME_LENGTH]; char last_name[NAME_LENGTH]; };
void populate(struct person *p); void print(struct person *p);
#endif
PERSON.C
#include
#include "person.h" #include "mystat.h"
#define BASE_SALARY 30000
// Print the information of a person. void print(struct person *p) { float avg_best_five_years = 0; char s[TWO_NAME_LENGTH];
average(p->salary, NUM_YEARS, &avg_best_five_years); sprintf(s,"%s %s",p->first_name,p->last_name); printf("Person Name: %-32s, Age:%3hu, salary average: %7.2f ", s, p->age, avg_best_five_years); }
// Populate the information of a person. void populate(struct person *p) {
int i; static long long id = 0; char *first_name[5] = { "David", "John", "Diana", "Rob", "Sarah" }; char *last_name[5] = { "Smith", "Bell", "West", "Johnson", "Ford" };
strncpy(p->last_name, last_name[rand() % 5], NAME_LENGTH-1); strncpy(p->first_name, first_name[rand() % 5], NAME_LENGTH-1);
p->id = id++; p->age = (rand() % 45) + 20;
// Populate the salary information. for (i = 0; i < NUM_YEARS; i++) { p->salary[i] = BASE_SALARY + (rand() & 5000); } }
MYSTAT.H
#ifndef HEADER_MYSTAT #define HEADER_MYSTAT
void average(float *arr, int size, float *average);
#endif
MYSTAT.C
#include
#include "mystat.h"
// Compute the average of an array of floats. void average(float *arr, int size, float *average) { int i; *average = 0;
for (i = 0; i < size; i++, arr++) { *average += *arr; } *average /= size; }
MAIN.C
#include
#include "person.h"
#define NUM_PEOPLE 5
int main(int argc, char *argv[]) { struct person people[NUM_PEOPLE]; int i;
for (i = 0; i < NUM_PEOPLE; i++) { populate(&people[i]); }
for (i = 0; i < NUM_PEOPLE; i++) { print(&people[i]); }
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
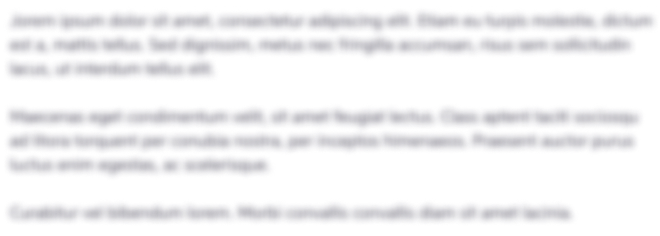
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started