Question
please answer this question. This is for data structure course. steps: 1) write a sequence2.cpp file 2) write sequence2.h file 3) test it 4) show
please answer this question. This is for data structure course. steps: 1) write a sequence2.cpp file 2) write sequence2.h file 3) test it 4) show your results that your codes passed by that test.
The Assignment:
You will implement and test a revised sequence class that uses a dynamic array to store the items.
Purposes:
Ensure that you can write a small class that uses a dynamic array as a private member variable.
Programming files that you must write and turn in:
1 sequence2.h: The header file for the new sequence class. Actually, you don't have to write much of this file. Just start with our version and add your name and other information at the top. If some of your member functions are implemented as inline functions, then you may put those implementations in this file too. By the way, you might want to compare this header file with your first sequence header file (sequence1.h) . The new version no longer has a CAPACITY constant because the items are stored in a dynamic array that grows as needed. But there is a DEFAULT_CAPACITY constant, which provides the initial size of the array for a sequence created by the default constructor.
// Provided by: ____________(your name here)__________
// Email Address: ____________(your email address here)________
// FILE: sequence2.h
// CLASS PROVIDED: sequence (part of the namespace main_savitch_4)
// There is no implementation file provided for this class since it is
// a programming assignment from Chpater 4 of "Data Structures and Other Objects Using C++"
//
// TYPEDEFS and MEMBER CONSTANTS for the sequence class:
// typedef ____ value_type
// sequence::value_type is the data type of the items in the sequence. It
// may be any of the C++ built-in types (int, char, etc.), or a class with a
// default constructor, an assignment operator, and a copy constructor.
//
// typedef ____ size_type
// sequence::size_type is the data type of any variable that keeps track of
// how many items are in a sequence.
//
// static const size_type DEFAULT_CAPACITY = _____
// sequence::DEFAULT_CAPACITY is the initial capacity of a sequence that is
// created by the default constructor.
//
//
// CONSTRUCTOR for the sequence class:
// sequence(size_type initial_capacity = DEAULT_CAPACITY)
// Postcondition: The sequence has been initialized as an empty sequence.
// The insert/attach functions will work efficiently (without allocating
// new memory) until this capacity is reached.
//
// sequence(const sequence& source)
- write the preconditions and postconditions yourself!
//
// MODIFICATION MEMBER FUNCTIONS for the sequence class:
// void resize(size_type new_capacity)
// Postcondition: The sequence's current capacity is changed to the
// new_capacity (but not less that the number of items already on the
// sequence). The insert/attach functions will work efficiently (without
// allocating new memory) until this new capacity is reached.
//
// void start( )
// Postcondition: The first item on the sequence becomes the current item
// (but if the sequence is empty, then there is no current item).
//
// void advance( )
// Precondition: is_item returns true.
// Postcondition: If the current item was already the last item on the
// sequence, then there is no longer any current item. Otherwise, the new
// current item is the item immediately after the original current item.
//
// void insert(const value_type& entry)
// Postcondition: A new copy of entry has been inserted in the sequence before
// the current item. If there was no current item, then the new entry has
// been inserted at the front of the sequence. In either case, the newly
// inserted item is now the current item of the sequence.
//
// void attach(const value_type& entry)
// Postcondition: A new copy of entry has been inserted in the sequence after
// the current item. If there was no current item, then the new entry has
// been attached to the end of the sequence. In either case, the newly
// inserted item is now the current item of the sequence.
//
// void remove_current( )
// Precondition: is_item returns true.
// Postcondition: The current item has been removed from the sequence, and the
// item after this (if there is one) is now the new current item.
//
// CONSTANT MEMBER FUNCTIONS for the sequence class:
// size_type size( ) const
// Postcondition: The return value is the number of items on the sequence.
//
// bool is_item( ) const
// Postcondition: A true return value indicates that there is a valid
// "current" item that may be retrieved by activating the current
// member function (listed below). A false return value indicates that
// there is no valid current item.
//
// value_type current( ) const
// Precondition: is_item( ) returns true.
// Postcondition: The item returned is the current item on the sequence.
//
// VALUE SEMANTICS for the sequence class:
// Assignments and the copy constructor may be used with sequence objects.
//
// DYNAMIC MEMORY USAGE by the sequence
// If there is insufficient dynamic memory, then the following functions
// call new_handler: The constructors, insert, attach.
#ifndef MAIN_SAVITCH_SEQUENCE2_H
#define MAIN_SAVITCH_SEQUENCE2_H
#include
namespace main_savitch_4
{
class sequence
{
public:
// TYPEDEFS and MEMBER CONSTANTS
typedef double value_type;
typedef size_t size_type; //typedef std::size_t size_type;
static const size_type DEFAULT_CAPACITY = 30; //enum {DEFAULT_CAPACITY = 30}; //
// CONSTRUCTOR
sequence(size_type initial_capacity = DEFAULT_CAPACITY);
sequence(const sequence& source);
~sequence( );
// MODIFICATION MEMBER FUNCTIONS
void start( );
void advance( );
void insert(const value_type& entry);
void attach(const value_type& entry);
void remove_current( );
void resize(size_type new_capacity);
void operator=(const sequence& source);
// CONSTANT MEMBER FUNCTIONS
size_type size( ) const;
bool is_item( ) const;
value_type current( ) const;
private:
-- Fill in your private member variables here.
-- You'll need a pointer to a dynamic array, and a size_type
-- variable to keep track of the current length of the
-- sequence, an index to the current item, and
-- another size_type variable to keep track of the
-- complete capacity of the dynamic array.
};
}
#endif
2 sequence2.cxx: The implementation file for the new sequence class. You will write all of this file, which will have the implementations of all the sequence's member functions.
3) Test file:
// FILE: sequence_test.cxx
// An interactive test program for the new sequence class
#include
#include
#include
#include "sequence1.h" // With value_type defined as double
using namespace std;
using namespace main_savitch_3;
// PROTOTYPES for functions used by this test program:
void print_menu( );
// Postcondition: A menu of choices for this program has been written to cout.
char get_user_command( );
// Postcondition: The user has been prompted to enter a one character command.
// The next character has been read (skipping blanks and newline characters),
// and this character has been returned.
void show_sequence(sequence display);
// Postcondition: The items on display have been printed to cout (one per line).
double get_number( );
// Postcondition: The user has been prompted to enter a real number. The
// number has been read, echoed to the screen, and returned by the function.
int main( )
{
sequence test; // A sequence that well perform tests on
char choice; // A command character entered by the user
cout << "I have initialized an empty sequence of real numbers." << endl;
do
{
print_menu( );
choice = toupper(get_user_command( ));
switch (choice)
{
case '!': test.start( );
break;
case '+': test.advance( );
break;
case '?': if (test.is_item( ))
cout << "There is an item." << endl;
else
cout << "There is no current item." << endl;
break;
case 'C': if (test.is_item( ))
cout << "Current item is: " << test.current( ) << endl;
else
cout << "There is no current item." << endl;
break;
case 'P': show_sequence(test);
break;
case 'S': cout << "Size is " << test.size( ) << '.' << endl;
break;
case 'I': test.insert(get_number( ));
break;
case 'A': test.attach(get_number( ));
break;
case 'R': test.remove_current( );
cout << "The current item has been removed." << endl;
break;
case 'Q': cout << "Ridicule is the best test of truth." << endl;
break;
default: cout << choice << " is invalid." << endl;
}
}
while ((choice != 'Q'));
return EXIT_SUCCESS;
}
void print_menu( )
// Library facilities used: iostream.h
{
cout << endl; // Print blank line before the menu
cout << "The following choices are available: " << endl;
cout << " ! Activate the start( ) function" << endl;
cout << " + Activate the advance( ) function" << endl;
cout << " ? Print the result from the is_item( ) function" << endl;
cout << " C Print the result from the current( ) function" << endl;
cout << " P Print a copy of the entire sequence" << endl;
cout << " S Print the result from the size( ) function" << endl;
cout << " I Insert a new number with the insert(...) function" << endl;
cout << " A Attach a new number with the attach(...) function" << endl;
cout << " R Activate the remove_current( ) function" << endl;
cout << " Q Quit this test program" << endl;
}
char get_user_command( )
// Library facilities used: iostream
{
char command;
cout << "Enter choice: ";
cin >> command; // Input of characters skips blanks and newline character
return command;
}
void show_sequence(sequence display)
// Library facilities used: iostream
{
for (display.start( ); display.is_item( ); display.advance( ))
cout << display.current( ) << endl;
}
double get_number( )
// Library facilities used: iostream
{
double result;
cout << "Please enter a real number for the sequence: ";
cin >> result;
cout << result << " has been read." << endl;
return result;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
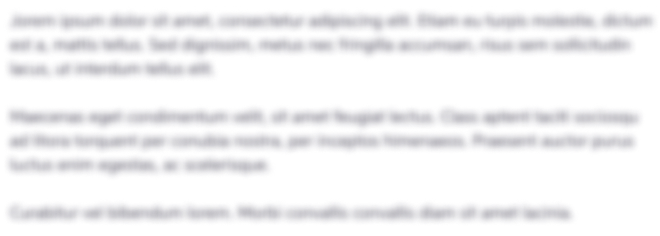
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started