Question
Please assist: IF NEEDED I CAN SEND FULL FILES Start by downloading the attached Week5_Pizza_FileIO_Shell.zip program, unzip the program and open it up into Netbeans.
Please assist: IF NEEDED I CAN SEND FULL FILES
Start by downloading the attached "Week5_Pizza_FileIO_Shell.zip" program, unzip the program and open it up into Netbeans. The shell project will compile and execute, and even read a stream file and populate the list with data from a string file.
Steps
You are then asked to complete the following TODO action items:
Review the FileStream Class and:
- in the readlist method add the statements to add a string to the array list
- in the writeData method add the statement to write the string to the file
Review the PizzaFileIO class and:
- In the writeData method add code to add the missing order fields to the record
- In the readData method add code to add the missing fields to the order object
Review the OrderList class
- In the remove method add statements to remove the order from the list
- In the save method add the statement to write the array list to the file.
Graphical User Interface
Update the given graphical user interface to:
- Save the list in the Order list to a file using both types of file I/O (streams and objects).
- Retrieve the list from the file and display the list
- Add a menu items Save Orders and Retrieve Orders
- Update retrieveOrders method to retrieve the list from the orderList object.
in OrderList.java;
public void remove(PizzaOrder aOrder) {
if (aOrder != null) {
//TODO: remove the order object from the arraylist
}
}
public int save() throws IOException {
int count = 0;
//TODO: write the array list to file
return count;
}
public int retrieve(DefaultListModel listModel) throws IllegalArgumentException, IOException {
int count = 0;
orderList = streamFile.readData();
if (!orderList.isEmpty()) {
listModel.clear();
count = orderList.size();
for(PizzaOrder order : orderList) {
listModel.addElement(order);
}
}
public void remove(PizzaOrder aOrder) {
if (aOrder != null) {
//TODO: remove the order object from the arraylist
}
}
public int save() throws IOException {
int count = 0;
//TODO: write the array list to file
return count;
}
//Pizza_Main.java
private void retrieveOrders() throws IllegalArgumentException, IOException {
int count = 0;
//TODO: add statement to retrieved the order list (remember to pass in the list model)
String message = count + " pizza orders retreived from file: " + orderList.getFileName();
JOptionPane.showMessageDialog(null, message, "Orders Retrieved", JOptionPane.PLAIN_MESSAGE);
}
//FileStream.java
public static Boolean writeData(String data, String fileName) throws IOException
{
Boolean success;
try
{
FileWriter dataFile = new FileWriter(fileName, false);
try (PrintWriter output = new PrintWriter(dataFile)) {
//TODO: provide the statement to write the entire data string to the file
}
success = true;
}
catch (IOException ioe)
{
JOptionPane.showMessageDialog(null, ioe.getMessage(), "File Write Error", JOptionPane.ERROR);
success = false;
}
return success;
}
public static ArrayList readList(String fileName) throws FileNotFoundException, IllegalArgumentException, IOException
{
ArrayList dataList = new ArrayList<>();
String str;
try {
String file = checkFile(fileName);
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
while ((str = br.readLine()) != null)
{
//TODO: write the statement to add the string to the arrayList
}
}
}
catch (IOException ioe)
{
JOptionPane.showMessageDialog(null, ioe.getMessage(), "File Read Error", JOptionPane.ERROR);
dataList = null;
}
return dataList;
}
//FileStream.java
public static Boolean writeData(String data, String fileName) throws IOException
{
Boolean success;
try
{
FileWriter dataFile = new FileWriter(fileName, false);
try (PrintWriter output = new PrintWriter(dataFile)) {
//TODO: provide the statement to write the entire data string to the file
}
success = true;
}
catch (IOException ioe)
{
JOptionPane.showMessageDialog(null, ioe.getMessage(), "File Write Error", JOptionPane.ERROR);
success = false;
}
return success;
}
try {
String file = checkFile(fileName);
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
while ((str = br.readLine()) != null)
{
//TODO: write the statement to add the string to the arrayList
}
//PizzaFileO.java
if(!orderList.isEmpty()) {
for(PizzaOrder aOrder:orderList) {
count++;
recordList.append(aOrder.getFirstName());
recordList.append(DELIMTER);
recordList.append(aOrder.getLastName());
recordList.append(DELIMTER);
recordList.append(aOrder.getPizzaSize());
recordList.append(DELIMTER);
recordList.append(aOrder.getCheese());
recordList.append(DELIMTER);
//TODO, write the code to: (remember to separate the fields by the delimiter)
//1. add the statement to add the sausage value to the record
//2. add the statement to add the ham value to the record
//3. add the statements to add the total value to the record
aOrder.setCheese(Boolean.valueOf(row.nextToken()));
//TODO: add statements to:
//1. add the sausage value to the order object
//2. add the ham order to the object
//3. add the total value to the order (keeping in mind you have to "parse" the value into a double
//4. add the order to the array list
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
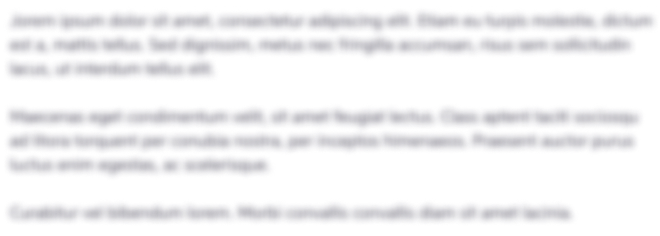
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started