Question
Please attempt, due in 50 minutes I need help Your objective is to implement the main operations of a binary search tree from the lecture.
Please attempt, due in 50 minutes I need help
Your objective is to implement the main operations of a binary search tree from the lecture. To be clear, this entails creating a class for your tree nodes, then creating another class for your binary search tree. Create a class called Node (this is different from your Linked List Node class) that has three fields: a field called left for the left child link, a field called right for the right child link and a field called data for the int data being held. These fields should be private with public accessors and mutators (i.e. getLeft, getRight, getData, setLeft, setRight and setData). This Node class can either be a public class in its own file (i.e. Node.java) or a class without the public keyword in the same file as your BST class (described below). Create a public class called BST (and put it in a file called BST.java) that has the following: Fields - public field called root that points to the root of the tree. This must be public for the visualization to work. - Any other fields you need - Public methods - A default constructor (i.e. a constructor that doesn't take any parameters). This will initialize root to null. - insert(value) a. Takes an int as input and inserts it into the logical place in the tree b. You don't have to worry about duplicate values (see 'Edge Cases and Notes' for more information) c. You may use either recursion or iteration here. - search(value) a. This method takes an int as input and returns true if the given value is in the tree, false otherwise. You don't have to return the location in the tree, just a boolean indicating whether the value is found. b. If root is null, you should return false (since the value could not be found) c. You may use either recursion or iteration here. - delete(value) a. This method takes an int as input, searches for it in the tree and deletes it according to the method described in the nodes. b. If the value given is not found or if the root is null, this operation does nothing c. Do NOT even attempt this method until everything else works! d. You may use either recursion or iteration here.
- min() a. Returns the smallest value in the tree. b. If the root is null, return -1 c. You may use either recursion or iteration here. - max() a. Returns the largest value in the tree. b. If the root is null, return -1 c. You may use either recursion or iteration here. - inorder() a. Traverses the tree according to the inorder traversal described in the notes. b. Do not print values. Instead, construct a String, concatenating the node values as they are processed with either a comma or a space in between values. Return this String. c. If the root is null, return an empty string (i.e. "" with nothing in between the quotes) d. You must use recursion here. - preorder() a. Traverses the tree according to the preorder traversal described in the notes. b. Do not print values. Instead, construct a String, concatenating the node values as they are processed with either a comma or a space in between values. Return this String. c. If the root is null, return an empty string (i.e. "" with nothing in between the quotes) d. You must use recursion here. - postorder() a. Traverses the tree according to the postorder traversal described in the notes. b. Do not print values. Instead, construct a String, concatenating the node values as they are processed with either a comma or a space in between values. Return this String. c. If the root is null, return an empty string (i.e. "" with nothing in between the quotes) d. You must use recursion here. Edge Cases and Notes: You do not have to use generics for this. For this assignment, just use int as the data type for the data stored in your binary search tree nodes. Do not worry about handling duplicate values. You can safely assume that I will not attempt to store the same value twice in your tree, so your tree does not have to handle this situation. Every function should handle the case where the root is null. See the function description above for instructions on how to handle it for that function. You may use helper functions to help you with the recursion. For example, you could have a public inorder method (i.e. public String inorder()) that simply calls a private inorderRecursive method (i.e. private String inorderRecursive(Node cur)) and sends it the root node to start the inorder traversal (i.e. inorderRecursive(root)). This is helpful since this means a developer using your tree class would only need to call tree.inorder(), assuming they have a BST object named "tree", without needing access to the root.
Main part: Make a separate public class called Main and put it into its own file. Put your entry point inside this class. Make sure to put Vis.class and Color.class in the same folder as your .java files if you plan on running your code on the command line (more on this below). Create an object of your BST class (call it whatever you like). You can now call whichever operations you want on your object. This will initialize your tree by giving it a set of nodes it will contain. When you are ready to see the visualize of your tree and test it in real-time, call Vis.test(nameOfYourTreeObject); Here is an example (this code would appear inside your main function in your Main class): // instantiate our BST object BST tree = new BST(); // load it up with some initial values tree.insert(5); tree.insert(15); tree.insert(2); // are you on Windows? Vis.runOnWindows = false; // set to true if running on Windows // test it out Vis.test(tree);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
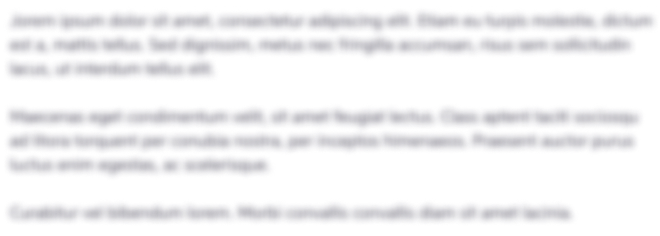
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started