Please Code in C++, PLEASE code stack.cpp, Stack.hpp, NumStack.cpp, and NumStack.hpp using the main.cpp as the TEST CASE ----------------------------------------- For the assignment, we are given
Please Code in C++, PLEASE code stack.cpp, Stack.hpp, NumStack.cpp, and NumStack.hpp using the main.cpp as the TEST CASE
-----------------------------------------
For the assignment, we are given a Stack class that has only has two member functions: add and sub. You will see also the specification file and implementation file for the NumStack class. The stack is implemented as an array of the type integer.
For this assignment, you will need to provide these public member functions that perform the following tasks:
a) mult Pops the top two values off the stack, multiplies them, and pushes their product onto the stack.
b) div Pops the top two values off the stack, divides the second value by the first, and pushes the quotient onto the stack.
c) addAll Pops all values off the stack, adds them, and pushes their sum onto the stack.
d) multAll Pops all values off the stack, multiplies them, and pushes their product onto the stack.
Next, you will need modify the NumStack class to that of a template that will create this stack for any data type.
There are plenty of specification and implementation files in this assignments folder. There is also the original driver program as well, called main. Modify the driver to include 2 cases of each of the 4 new member functions, and then make sure your program can execute the test cases successfully.
(TEST CASE) main:
#include
int main() { int catchVar; // To hold values popped off the stack
// Create a MathStack object. Stack stack(5);
// Push 3 and 6 onto the stack. std::cout << "Pushing 3 "; stack.push(3); std::cout << "Pushing 6 "; stack.push(6);
// Add the two values. stack.add();
// Pop the sum off the stack and display it. std::cout << "The sum is "; stack.pop(catchVar); std::cout << catchVar << std::endl << std::endl;
// Push 7 and 10 onto the stack std::cout << "Pushing 7 "; stack.push(7); std::cout << "Pushing 10 "; stack.push(10);
// Subtract 7 from 10. stack.sub();
// Pop the difference off the stack and display it. std::cout << "The difference is "; stack.pop(catchVar); std::cout << catchVar << std::endl; return 0; }
-------------------------------------------------------
Stack.hpp:
#ifndef STACK_HPP #define STACK_HPP #include "NumStack.hpp"
class Stack : public NumStack { public: // Constructor Stack(int s) : NumStack(s) {}
// MathStack operations void add(); void sub(); };
#endif // STACK_HPP_INCLUDED
--------------------------------------------------
NumStack.hpp:
#ifndef NUMSTACK_HPP_INCLUDED #define NUMSTACK_HPP_INCLUDED
class NumStack { private: int *stackArray; // Pointer to the stack array int stackSize; // The stack size int top; // Indicates the top of the stack
public: // Constructor NumStack(int);
// Copy constructor NumStack(const NumStack &);
// Destructor ~NumStack();
// Stack operations void push(int); void pop(int &); bool isFull() const; bool isEmpty() const; };
#endif // NUMSTACK_HPP_INCLUDED
------------------------------------------------------------------
stack.cpp:
#include "stack.hpp"
//*********************************************** // Member function add. add pops * // the first two values off the stack and * // adds them. The sum is pushed onto the stack. * // pre: no parameters * // post: return the new stack entry of sum * //***********************************************
void Stack::add() { int num, sum;
// Pop the first two values off the stack. pop(sum); pop(num);
// Add the two values, store in sum. sum += num;
// Push sum back onto the stack. push(sum); }
//*********************************************** // Member function sub. sub pops the * // first two values off the stack. The * // second value is subtracted from the * // first value. The difference is pushed * // onto the stack. * // pre: no parameters * // post: return the new stack entry of diff * //***********************************************
void Stack::sub() { int num, diff;
// Pop the first two values off the stack. pop(diff); pop(num);
// Subtract num from diff. diff -= num;
// Push diff back onto the stack. push(diff); }
---------------------------------------------------------
NumStack.cpp:
#include #include "NumStack.hpp"
//*********************************************** // Constructor * // This constructor creates an empty stack. The * // size parameter is the size of the stack. * //***********************************************
NumStack::NumStack(int size) { stackArray = new int[size]; stackSize = size; top = -1; }
//*********************************************** // Copy constructor * //***********************************************
NumStack::NumStack(const NumStack &obj) { // Create the stack array. if (obj.stackSize > 0) stackArray = new int[obj.stackSize]; else stackArray = nullptr;
// Copy the stackSize attribute. stackSize = obj.stackSize;
// Copy the stack contents. for (int count = 0; count < stackSize; count++) stackArray[count] = obj.stackArray[count];
// Set the top of the stack. top = obj.top; }
//*********************************************** // Destructor * //***********************************************
NumStack::~NumStack() { delete [] stackArray; }
//************************************************* // Member function push pushes the argument onto * // the stack. * //*************************************************
void NumStack::push(int num) { if (isFull()) { std::cout << "The stack is full. "; } else { top++; stackArray[top] = num; } }
//**************************************************** // Member function pop pops the value at the top * // of the stack off, and copies it into the variable * // passed as an argument. * //****************************************************
void NumStack::pop(int &num) { if (isEmpty()) { std::cout << "The stack is empty. "; } else { num = stackArray[top]; top--; } }
//*************************************************** // Member function isFull returns true if the stack * // is full, or false otherwise. * //***************************************************
bool NumStack::isFull() const { bool status;
if (top == stackSize - 1) status = true; else status = false;
return status; }
//**************************************************** // Member function isEmpty returns true if the stack * // is empty, or false otherwise. * //****************************************************
bool NumStack::isEmpty() const { bool status;
if (top == -1) status = true; else status = false;
return status; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
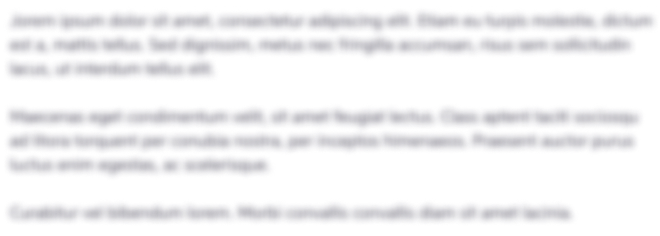
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started