Question
Please code in Java, and code HAS to be an extension of the code posted below. Please post screenshot of output. Extend the DeckofCards class
Please code in Java, and code HAS to be an extension of the code posted below. Please post screenshot of output.
Extend the DeckofCards class to implement a card game application such as BlackJack, Texas poker or others.
Your game should support multiple players (up to 5 for BlackJack).
You must build your game based on the Cards and DeckofCards class from the book.
You need to implement the logic of the game.
Hint:
Use a test class to test above classes. Include a UML based problem solving process in your submission.
// Card class represents a playing card. public class Card { private String face; // face of card ("Ace", "Deuce", ...) private String suit; // suit of card ("Hearts", "Diamonds", ...) // two-argument constructor initializes card's face and suit public Card( String cardFace, String cardSuit ) { face = cardFace; // initialize face of card suit = cardSuit; // initialize suit of card } // end two-argument Card constructor // return String representation of Card public String toString() { return face + " of " + suit; } // end method toString } // end class Card
public class DeckOfCards { private Card deck[]; // array of Card objects private int currentCard; // index of next Card to be dealt private final int NUMBER_OF_CARDS = 52; // constant number of Cards private Random randomNumbers; // random number generator
// constructor fills deck of Cards public DeckOfCards() { String faces[] = { "Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King" }; String suits[] = { "Hearts", "Diamonds", "Clubs", "Spades" };
deck = new Card[ NUMBER_OF_CARDS ]; // create array of Card objects currentCard = 0; // set currentCard so first Card dealt is deck[ 0 ] randomNumbers = new Random(); // create random number generator
// populate deck with Card objects for ( int count = 0; count < deck.length; count++ ) deck[ count ] = new Card( faces[ count % 13 ], suits[ count / 13 ] ); } // end DeckOfCards constructor
// shuffle deck of Cards with one-pass algorithm public void shuffle() { // after shuffling, dealing should start at deck[ 0 ] again currentCard = 0; // reinitialize currentCard
// for each Card, pick another random Card and swap them for ( int first = 0; first < deck.length; first++ ) { // select a random number between 0 and 51 int second = randomNumbers.nextInt( NUMBER_OF_CARDS ); // swap current Card with randomly selected Card Card temp = deck[ first ]; deck[ first ] = deck[ second ]; deck[ second ] = temp; } // end for } // end method shuffle
// deal one Card public Card dealCard() { // determine whether Cards remain to be dealt if ( currentCard < deck.length ) return deck[ currentCard++ ]; // return current Card in array else return null; // return null to indicate that all Cards were dealt } // end method dealCard } // end class DeckOfCards
Step by Step Solution
There are 3 Steps involved in it
Step: 1
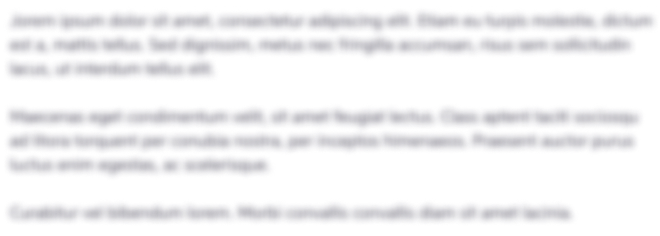
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started