Question
// PLEASE CODE THE FOLLOWING IN C: call calculateScore, insertionSort, writeFile, and displayWinners under main. Also code void displayWinners(const LIST *perfData) in C. Thank you.
// PLEASE CODE THE FOLLOWING IN C: call calculateScore, insertionSort, writeFile, and displayWinners under main.
Also code void displayWinners(const LIST *perfData) in C. Thank you.
#include
#include
#include
#define NUM_JUDGES 5
#define NUM_PERFRM 100
#define NAME_SIZE 32
typedef struct
{
char name[NAME_SIZE];
int scores[NUM_JUDGES];
int final;
}PERFORMER;
typedef struct
{
int size; // the actual number of performers
PERFORMER list[NUM_PERFRM]; // the array of performers
} LIST;
void printInfo(void);
void printEnd(void);
int readPerfData(LIST *perfData, char fileName[]);
int getOutFileName(const char fileName[], char outFileName[]);
void writeFile(const LIST *perfData, char outFileName[]);
void calculateScore(LIST *perfData);
void getMinMax(const int scoreList[], int *min, int *max);
void insertionSort(LIST *perfData);
void displayWinners(const LIST *perfData);
void showErrorMessage(int errorCode, char s[]);
int main (void)
{
char inFileName[][NAME_SIZE] =
{"performers.txt", "contestants.txt", "abc.txt", "test", "test.txt", ""};
LIST perfData;
int i, success;
char outFileName[32];
// call printInfo
printInfo();
for ( i = 0; *(inFileName[i]) != '\0'; i++)
{
if (!getOutFileName(inFileName[i], outFileName))
showErrorMessage(0, inFileName[i]);
else
{
success = readPerfData(&perfData, inFileName[i]);
if (success != 1)
showErrorMessage(success, inFileName[i]);
else // everything is OK
{
printf("Reading from \"%s\" . . . Writing to \"%s\" ", inFileName[i], outFileName);
// call calculateScore
// call insertionSort
// call writeFile
// call displayWinners
}
}
}
// call printEnd
printEnd();
return 0;
}
/* *******************************************************
Displays the winner(s)
PRE : perfData
POST : one or more winners displayed
*/
void displayWinners(const LIST *perfData)
{
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
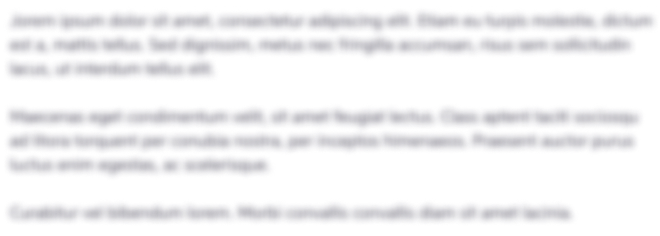
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started