Question
Please Complete in C++ Language Introduce a linked list to your program Modify the BookArray class (if you are starting from your Tutorial 5 code)
Please Complete in C++ Language
Introduce a linked list to your program
Modify the BookArray class (if you are starting from your Tutorial 5 code) or the Library class (if you are starting from the original LMS code base) so that the underlying collection for storing books is a linked list, rather than a C-style array. You will implement this list the way we did in class, as a singly linked list, using no dummy nodes. Your list will have a head but no tail. Do not make changes to the BookArray or the Library class interface! All existing member function prototypes must stay the same. Make all required changes to the BookArray or the Library member function implementations so that no other classes in the program need to change. Adding a book should always add it to the end of the list.
---------------------Book.hh--------------------------------
#ifndef BOOK_H #define BOOK_H
#include
#include "types.h"
class Book { public: Book(); Book(string, string, int); void setStatus(BookStatusType); BookStatusType getStatus(); int getId(); string getTitle(); string getAuthor(); private: static int nextId; int id; string title; string author; int year; BookStatusType status; };
#endif
---------------------Book.cc--------------------------------
#include "Book.h"
int Book::nextId = 1001;
Book::Book() { title = ""; author = ""; year = 0; id = nextId++; status = CHECKED_IN; }
Book::Book(string t, string a, int y) { title = t; author = a; year = y; id = nextId++; status = CHECKED_IN; }
void Book::setStatus(BookStatusType s) { status = s; }
int Book::getId() { return id; } string Book::getTitle() { return title; } string Book::getAuthor() { return author; } BookStatusType Book::getStatus() { return status; }
---------------------BookArray.h--------------------------------
#ifndef BOOKARRAY_H
#define BOOKARRAY_H
#include "Book.h"
class BookArray
{
public:
BookArray();
~BookArray(); //tutorial 6
int addBook(Book*);
Book* getBook(int);
int getNumBooks();
private:
Book* books[MAX_COLL_SIZE];
int numBooks;
};
#endif
---------------------BookArray.cc--------------------------------
#include "BookArray.h"
#include "Book.h"
#include "types.h"
//added
/*The constructor simply initializes the size data member properly*/
BookArray::BookArray(){
numBooks =0;
}
/*The destructor must iterate over the stored objects (which are to be dynamically
allocated), freeing the allocated memory for each one.*/
BookArray::~BookArray(){ //tutorial6
for(int i=0; i delete books[i]; books[i] = NULL; } } /*getSize Getter*/ int BookArray::getNumBooks() { return numBooks; } /*The add function takes a pointer to a dynamically allocated object. It checks if there is room in the array of pointers for this object. If not, it returns C_NOK (defined in defs.h). If there is, it sets the appropriate pointer in the array to point at the same object as the parameter, increments the size data member and returns C_OK (also defined in defs.h).*/ int BookArray::addBook(Book* book) { if (numBooks >= MAX_COLL_SIZE - 1) { return C_NOK; } books[numBooks++] = book; return C_OK; } /*The get function takes an index value. If this index is not valid (ie. it is outside of the range of valid objects being stored), the function returns 0. Otherwise it returns the pointer at index i.*/ Book* BookArray::getBook(int index) { if (index < 0 || index >= numBooks) return 0; return books[index]; } ---------------------LibControl.h------------------------------- #ifndef LIB_CONTROL_H #define LIB_CONTROL_H #include "types.h" #include "Library.h" #include "View.h" class View; class LibControl { public: LibControl(); void launch(); void addPatron(); private: Library lib; View view; void initLib(); }; #endif ---------------------LibControl.cc------------------------------ #include #include using namespace std; #include "LibControl.h" LibControl::LibControl() { initLib(); } void LibControl::launch() { int mainChoice = -1; int adminChoice = -1; int patronChoice = -1; Patron* p; string fn, ln; int rc; while (mainChoice != 0) { view.mainMenu(mainChoice); switch(mainChoice) { case 1: // 1. Admin menu while (adminChoice != 0) { view.adminMenu(adminChoice); switch(adminChoice) { case 1: // 1. Add patron addPatron(); break; default: // 0. Exit admin menu break; } } adminChoice = -1; break; case 2: // 2. Patron menu view.getPatronName(fn, ln); rc = lib.findPatron(fn, ln, &p); if (rc != C_OK) { view.printError("Could not find patron..."); break; } while (patronChoice != 0) { view.patronMenu(patronChoice); switch(patronChoice) { default: // 0. Exit patron menu break; } } patronChoice = -1; break; case 3: // 3. View collection view.viewCollection(lib); break; default: // 0. Exit main menu break; } } view.printAll(lib); } void LibControl::addPatron() { Patron *patron; string fn, ln; int rc; view.getPatronName(fn, ln); patron = new Patron(fn, ln); rc = lib.addPatron(patron); if (rc != C_OK) { view.printError(" Could not add patron to library, press } } void LibControl::initLib() { Book* newBook; Patron* newPatron; newBook = new Book("Ender's Game", "Orson Scott Card", 1985); lib.addBook(newBook); newBook = new Book("Dune", "Frank Herbert", 1965); newBook->setStatus(LOST); lib.addBook(newBook); newBook = new Book("Foundation", "Isaac Asimov", 1951); lib.addBook(newBook); newBook = new Book("Hitch Hiker's Guide to the Galaxy", "Douglas Adams", 1979); lib.addBook(newBook); newBook = new Book("1984", "George Orwell", 1949); lib.addBook(newBook); newBook = new Book("Stranger in a Strange Land", "Robert A. Heinlein", 1961); newBook->setStatus(UNDER_REPAIR); lib.addBook(newBook); newBook = new Book("Farenheit 451", "Ray Bradbury", 1954); newBook->setStatus(LOST); lib.addBook(newBook); newBook = new Book("2001: A Space Odyssey", "Arthur C. Clarke", 1968); lib.addBook(newBook); newBook = new Book("I, Robot", "Isaac Asimov", 1950); lib.addBook(newBook); newBook = new Book("Starship Troopers", "Robert A. Heinlein", 1959); lib.addBook(newBook); newBook = new Book("Do Androids Dream of Electric Sheep?", "Philip K. Dick", 1968); lib.addBook(newBook); newBook = new Book("Neuromancer", "William Gibson", 1984); newBook->setStatus(LOST); lib.addBook(newBook); newBook = new Book("Ringworld", "Larry Niven", 1970); lib.addBook(newBook); newBook = new Book("Rendezvous with Rama", "Arthur C. Clarke", 1973); newBook->setStatus(UNDER_REPAIR); lib.addBook(newBook); newBook = new Book("Hyperion", "Dan Simmons", 1989); lib.addBook(newBook); newPatron = new Patron("Jack", "Shephard"); lib.addPatron(newPatron); newPatron = new Patron("Kate", "Austen"); lib.addPatron(newPatron); newPatron = new Patron("Hugo", "Reyes"); lib.addPatron(newPatron); newPatron = new Patron("James", "Ford"); lib.addPatron(newPatron); newPatron = new Patron("Sayid", "Jarrah"); lib.addPatron(newPatron); newPatron = new Patron("Sun-Hwa", "Kwon"); lib.addPatron(newPatron); newPatron = new Patron("Jin-Soo", "Kwon"); lib.addPatron(newPatron); newPatron = new Patron("John", "Locke"); lib.addPatron(newPatron); newPatron = new Patron("Juliet", "Burke"); lib.addPatron(newPatron); newPatron = new Patron("Benjamin", "Linus"); lib.addPatron(newPatron); } ---------------------Library.h------------------------------- #ifndef LIBRARY_H #define LIBRARY_H #include "Patron.h" #include "PatronArray.h" #include "Book.h" #include "BookArray.h" class Library { public: int addPatron(Patron*); Patron* getPatron(int); PatronArray& getPatrons(); int findPatron(string, string, Patron**); int getNumPatrons(); int addBook(Book*); Book* getBook(int); BookArray& getBooks(); int getNumBooks(); private: /* Book* books[MAX_COLL_SIZE]; Patron* patrons[MAX_COLL_SIZE]; */ BookArray books; PatronArray patrons; }; #endif ---------------------Library.cc------------------------------ #include "Library.h" //#include "types.h" int Library::addPatron(Patron* p) { return patrons.addPatron(p); } Patron* Library::getPatron(int i) { return patrons.getPatron(i); } PatronArray& Library::getPatrons() { return patrons; } int Library::findPatron(string fn, string ln, Patron** patron) { return C_OK; } int Library::getNumPatrons() { return patrons.getNumPatrons(); } int Library::addBook(Book* p) { return books.addBook(p); } Book* Library::getBook(int i) { return books.getBook(i); } BookArray& Library::getBooks() { return books; } int Library::getNumBooks() { return books.getNumBooks(); } ---------------------Patron.h------------------------------- #ifndef PATRON_H #define PATRON_H #include class Patron { public: Patron(string="", string=""); string getFname(); string getLname(); private: string fname; string lname; }; #endif ---------------------Patron.cc------------------------------ #include "Patron.h" Patron::Patron(string fn, string ln) { fname = fn; lname = ln; } string Patron::getFname() { return fname; } string Patron::getLname() { return lname; } ---------------------PatronArray.h------------------------------- #ifndef PATRONARRAY_H #define PATRONARRAY_H #include "Patron.h" class PatronArray { public: PatronArray(); ~PatronArray(); //tutorial6 int addPatron(Patron*); int getNumPatrons(); Patron* getPatron(int); int findPatron(string, string, Patron**); private: Patron* patrons[MAX_COLL_SIZE]; int numPatrons; }; #endif ---------------------PatronArray.cc------------------------------ #include "PatronArray.h" #include "Patron.h" #include "types.h" /*The constructor simply initializes the size data member properly*/ PatronArray::PatronArray(){ numPatrons = 0; } /*The destructor must iterate over the stored objects (which are to be dynamically allocated), freeing the allocated memory for each one.*/ PatronArray::~PatronArray(){ //tutorial 6 for(int i=0; i delete patrons[i]; patrons[i] = NULL; } } /*getSize Getter*/ int PatronArray::getNumPatrons() { return numPatrons; } /*The add function takes a pointer to a dynamically allocated object. It checks if there is room in the array of pointers for this object. If not, it returns C_NOK (defined in defs.h). If there is, it sets the appropriate pointer in the array to point at the same object as the parameter, increments the size data member and returns C_OK (also defined in defs.h).*/ int PatronArray::addPatron(Patron* patron) { if (numPatrons >= MAX_COLL_SIZE - 1) { return C_NOK; } patrons[numPatrons++] = patron; return C_OK; } /*The get function takes an index value. If this index is not valid (ie. it is outside of the range of valid objects being stored), the function returns 0. Otherwise it returns the pointer at index i.*/ Patron* PatronArray::getPatron(int index) { if (index < 0 || index >= numPatrons) return 0; return patrons[index]; } int PatronArray::findPatron(string fn, string ln, Patron** patron) { for (int i=0; i if (patrons[i]->getFname() == fn && patrons[i]->getLname() == ln) { *patron = patrons[i]; return C_OK; } } *patron = 0; return C_NOK; } ---------------------View.h------------------------------- #ifndef VIEW_H #define VIEW_H #include "Library.h" #include "Book.h" #include "PatronArray.h" #include "BookArray.h" #include "Patron.h" class View { public: View(); void mainMenu(int&); void adminMenu(int&); void patronMenu(int&); void viewCollection(Library&); void printAll(Library&); void printError(const string&); void getPatronName(string&, string&); private: void printCollection(Library&); void printPatrons(Library&); void printBookInfo(Book*); }; #endif ---------------------View.cc------------------------------ #include //added #include "View.h" /*#include "BookArray.h" #include "Book.h" #include "PatronArray.h" #include "Patron.h"*/ View::View() { } void View::mainMenu(int& choice) { string str; choice = -1; cout<< " WELCOME TO THE LIBRARY SYSTEM "; cout<< " Here are your options: "; cout<< " 1. Administrator Menu "; cout<< " 2. Patron Menu "; cout<< " 3. View Entire Collection "; cout<< " 0. Exit "; cout<< " Enter one of the choices above: "; getline(cin, str); while (str != "1" && str != "2" && str != "3" && str != "0") { cout<< " Enter one of the choices above: "; getline(cin, str); } stringstream ss(str); ss >> choice; } void View::adminMenu(int& choice) { string str; choice = -1; cout<< " ADMINISTRATOR MENU "; cout<< " Here are your options: "; cout<< " 1. Add a Patron "; cout<< " 0. Exit "; cout<< " Enter one of the choices above: "; getline(cin, str); while (str != "1" && str != "0") { cout<< " Enter one of the choices above: "; getline(cin, str); } stringstream ss(str); ss >> choice; } void View::patronMenu(int& choice) { string str; choice = -1; cout<< " PATRON MENU "; cout<< " Here are your options: "; cout<< " 0. Exit "; cout<< " Enter one of the choices above: "; getline(cin, str); while (str != "0") { cout<< " Enter one of the choices above: "; getline(cin, str); } stringstream ss(str); ss >> choice; } void View::viewCollection(Library& lib) { printCollection(lib); cout<< " Press void View::printAll(Library& lib) { printCollection(lib); printPatrons(lib); } void View::printCollection(Library& lib) { cout << endl << setw(40)<< "COLLECTION" < for (int i=0; i void View::printBookInfo(Book* book) { cout<< " "< switch(book->getStatus()) { case CHECKED_IN: cout<< " "< void View::printPatrons(Library& lib) { string str; cout<< endl< for (int i=0; i void View::printError(const string& err) { cout << err << endl; cin.get(); } void View::getPatronName(string& fn, string& ln) { string str; cout<< endl<<" Enter patron name ([first] [last]: "; getline(cin, str); stringstream ss(str); ss >> fn >> ln; } ---------------------types.h------------------------------- #ifndef TYPES_H #define TYPES_H #define MAX_COLL_SIZE 1024 #define C_OK 0 #define C_NOK -1 typedef enum {CHECKED_IN, CHECKED_OUT, UNDER_REPAIR, LOST} BookStatusType; #endif ---------------------main.cc------------------------------ #include #include "LibControl.h" int main() { LibControl control; control.launch(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
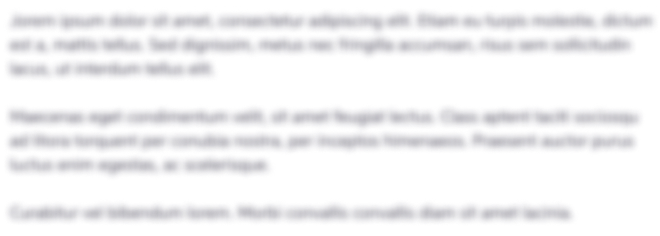
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started