Question
Please complete in C language. --------------------------------------struct.h------------------------------------------------ #ifndef STRUCT_HEADER #define STRUCT_HEADER /***************************************************************/ //DEFINES #define NAME_SIZE 15 // size of array for patient/employee name (maximum name length
Please complete in C language.
--------------------------------------struct.h------------------------------------------------
#ifndef STRUCT_HEADER
#define STRUCT_HEADER
/***************************************************************/
//DEFINES
#define NAME_SIZE 15 // size of array for patient/employee name (maximum name length is NAME_SIZE - 1)
//Patient related defines
#define PATIENT_TYPE 1 // determine that the record is a patient record
#define NUM_SEVERITIES 4 // number of different states of a patient
#define LIGHT 0
#define STABLE 1
#define ACCUTE 2
#define IMMEDIATE_SURGERY 3
//Employee related defines
#define EMPLOYEE_TYPE 0 // determines that the record is an employee record
#define MAX_POSITIONS 4 // number of different positions
#define DOCTOR 0
#define NURSE 1
#define SURGEON 2
#define ADMIN 3
// Department defines
#define MAX_DEPARTMENTS 6
// structure contains patient information
typedef struct patient {
int department; // department in hospital
long dailyCost; // cost of hospitalization per day
short numDaysInHospital; // number of days in hospital
char severity; // severity of illness
} PatientRec;
// structure contains employee information
typedef struct employee {
int position; // position of employee in hospital;
long yearsService; // years of service
unsigned long department; // department in hospital
float salary; // annual salary
} EmployeeRec;
// structure contains person information
typedef struct person {
char firstName[NAME_SIZE];
char familyName[NAME_SIZE];
char emplyeeOrPatient;
union {
EmployeeRec emp;
PatientRec patient;
};
} PersonRec;
#endif
-----------------------------populateRecords.c ---------------------------------------------------
/*
Purpose: populate the records of the patient and the employee
The file consists of several functions for ppopulating a person array and thus populating patient records and employee records
in the array.
The records are populated using random number generator. No special seed point is given (using the default seed of 1).
*/
/***********************************************************/
//INCLUDES
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "populateRecords.h"
/*********************************************************/
//FUNCTION PROTOTYPES
void populateEmployee(EmployeeRec *employee);
void populatePatient(PatientRec *patient);
/**************************************************************/
/* populates an employees
input
emp - an employee record
output
emp - populated employee
*/
/*****************************************************************/
// DEFINES
#define MAX_YEARS_SERVICE 63
#define MAX_SALARY 300000
/*****************************************************************/
void populateEmployee(EmployeeRec *emp)
{
emp->salary = (rand() % MAX_SALARY) * 1.34f ;
emp->yearsService = rand() % MAX_YEARS_SERVICE+1;
emp->department = rand() % MAX_DEPARTMENTS + 1;
emp->position = rand() % MAX_POSITIONS;
}
/**************************************************************/
/* populate a patient record
input
patient - a student record
output
patient - a student record
*/
#define MAX_DAILY_COST 50
#define MAX_DAYS_IN_HOSPITAL 30
void populatePatient(PatientRec *p)
{
p->department = rand() % MAX_DEPARTMENTS + 1;
p->dailyCost = rand() % MAX_DAILY_COST+5;
p->severity = rand() % NUM_SEVERITIES;
p->numDaysInHospital = rand() % MAX_DAYS_IN_HOSPITAL+1;
}
/**************************************************************/
/* populate an employee record
input
person - an array of persons
numPersons - number of person in the array
output
emp - array of employees
assumption:
numPersons is
*/
#define NUM_NAMES 7
void populateRecords(PersonRec *person, int numPersons)
{
int i = 0;
int j;
static int initRandom = 0;
char *fn[NUM_NAMES] = { "John", "Jane", "David", "Dina", "Justin","Jennifer", "Don" };
char *sn[NUM_NAMES] = { "Smith", "Johnson", "Mart", "Carp", "Farmer","Ouster","Door" };
if (!initRandom) {
initRandom++;
}
while (i
j = rand() % NUM_NAMES;
person->firstName[NAME_SIZE - 1] = '\0';
strncpy(person->firstName, fn[j], NAME_SIZE - 1);
j = rand() % NUM_NAMES;
person->familyName[NAME_SIZE - 1] = '\0';
strncpy(person->familyName, sn[j], sizeof(person->familyName) - 1);
if (rand() % 2) {
person->emplyeeOrPatient = PATIENT_TYPE;
populatePatient(&person->patient);
} else {
populateEmployee(&person->emp);
person->emplyeeOrPatient = EMPLOYEE_TYPE;
}
person++;
i++;
}
}
-----------------------------------populateRecords.h-------------------------------- /* Purpose: populate the records of the patient and the employee
The user can populate the reocrds of an array of tyupe person.
*/
#ifndef POPULATE_ARRAY_HEADER #define POPULATE_ARRAY_HEADER
#include "struct.h"
void populateRecords(PersonRec *person, int numRecords); // parameter: person and array of person records, nuMRecords the array size
#endif
----------------------------------hospital.c-------------------------------------------
#include "stdio.h"
#include "stdlib.h"
#include "struct.h"
#include "string.h"
#include "populateRecords.h"
#include "patient.h"
#include "employee.h"
#define NUM_RECORDS 20
int main()
{
struct person person[NUM_RECORDS];
char rc = 0;
// populating the array person with data of patients and employees
populateRecords(person, NUM_RECORDS);
return 0;
}
----------------------patient.h-----------------------------
// include files #include "struct.h"
/******************************************************/ // function prototypes
void printPatients(PersonRec *person, int numRecords); void printPatientSummary(PersonRec *person, int numRecords); void searchPatient(PersonRec *person, int numRecords); void printPatientSummary(PersonRec *person, int numRecords);
----------------------patient.c------------------------------
#include "string.h" #include "stdio.h" #include "patient.h"
/********************************************************************/ void printPatient(PersonRec person)
{
// add code
}
/********************************************************************/ void printPatients(PersonRec *person, int numRecords) {
// add code
}
/********************************************************************/ void searchPatient(PersonRec *person, int numRecords)
{
// add code
}
4.3. Print all Patients (10pts) Print all the patients. Hintsa. create a function to print a patient then use the function is a "for loop" chcking cach record if it is an employee record; b. use sprintf to print the first name and the last name to a temporary string. Then using the temporary string print the name using the formating Print format First Name Family Name (33) characters deptxx days in hospital:xxx severity:xx daily costxox total cost:xxxx John Johnson dept: 3 days in hospital: 21 severity: 0 daily cost: 32 total cost: 672 Output example Patient List John Johnson David Carp dept: 3 days in hospital: 21 severity: 0 daily cost: 32 total cost: 672 dept: 5 days in hospital: 26 severity: 1 daily cost: 37 total cost: 962Step by Step Solution
There are 3 Steps involved in it
Step: 1
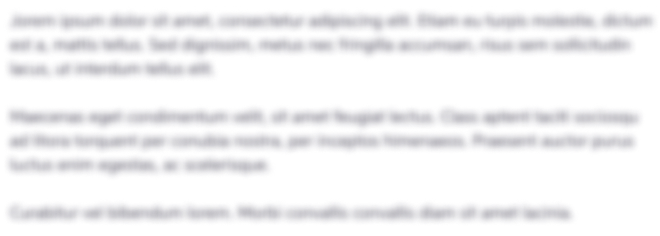
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started