Question
PLEASE COMPLETE MY CODE We want to create a C++ system that manages the employees of a pharmaceutical database. The database has two types of
PLEASE COMPLETE MY CODE
We want to create a C++ system that manages the employees of a pharmaceutical database. The database has two types of employees: Researchers and Administrators. Both types of employees have the following properties: employee id (int), name (string), position (string), seniority (int). In addition, researchers have the following attributes: title (string) and area of expertise (string). Administrative employees have the following additional attributes: number of employees that are managed by the employee (int).
Question1: Create the classes needed to model the employees of the pharmaceutical database. It is up to you to decide how many classes are needed. You must also decide about which functions should be virtual, constant, etc. You need to provide both a class definition and implementation. Each class must have at least the following functions:
A default constructor, a regular constructor, a copy constructor, a destructor
Accessing functions
A print function
Question 2 :We want to create a class, called EmployeeDB, to keep track of the employees defined in Q1. The class EmployeeDB stores the employee ID as its index and has the following attributes: name (string) address (string), list of employees (an array of objects of the Employee class), and number of employees the database has (int). Provide the definition and implementation of the class EmployeeDB. The class must implement the following functions:
A default constructor, a regular constructor, a copy constructor, a destructor
Accessing functions
A function that insert an employee to the database (message should show if the employee already exists)
A function that removes an employee from the company (message should show if the employee already exists)
A function that returns the number of employees of the company
A function that returns the list (names) of the employees
A function that returns the information of an employee given the employee id.
MY CODE IS MISSING TWO FUNCTIONS: ADD AND INSERT IN THE SECOND QUESTION , THE MAIN FUNCTION IS MISSING AN OBJECT OF EMPLOYEEDB AND THE TESTING OF THE FUNCTIONS
THIS IS MY CODE SO FAR :
**************************Employee.h**************************
#ifndef EMPLOYEES_H_
#define EMPLOYEES_H_
#include
#include
using namespace std;
class Employee {
protected:
int employeeId;
string name;
string position;
int seniority;
public:
Employee();
Employee(int, string, string, int); //constructor
Employee(const Employee &); //copy cobnstructor
virtual ~Employee(); //Destructor
void setemployeeId(int);
void setname(string);
void setposition(string);
void setseniority(int);
int getemployeeId();
string getname();
string getposition();
int getseniority();
virtual void printInfo()=0;
};
#endif
****************************************************************************************
*****************************Employee.cpp*******************************************
#include "Employee.h"
Employee::Employee() {
employeeId = 0;
seniority = 0;
name = "";
position = "";
}
Employee::Employee(int employeeIDd, string positionn, string namee, int seniorityy)
{
employeeId = employeeIDd;
position = positionn;
name = namee;
seniority = seniorityy;
}
//copy contructor
Employee::Employee(const Employee & emp) {
employeeId = emp.employeeId;
seniority = emp.seniority;
name = emp.name;
position = emp.position;
}
//destructor
Employee::~ Employee() {
cout << "Destructor called" << endl;
}
void Employee::setemployeeId(int employeeIDd) {
employeeId = employeeIDd;
}
void Employee::setname(string namee) {
name = namee;
}
void Employee::setposition(string positionn) {
position = positionn;
}
void Employee::setseniority(int seniorityy) {
seniority = seniorityy;
}
int Employee::getemployeeId() {
return employeeId;
}
string Employee::getname(){
return name;
}
string Employee::getposition() {
return position;
}
int Employee::getseniority() {
return seniority;
}
void Employee::printInfo()
{
cout << "Employee Id : " << getemployeeId() << endl;
cout << "Name :" << getname() << endl;
cout << "Position :" << getposition() << endl;
cout << "Seniority :" << getseniority() << endl;
}
*************************************************************************************
#ifndef RESEARCHER_H_
#define RESEARCHER_H_
#include
#include
#include "Employee.h"
using namespace std;
class researchers :public Employee {
private:
string title;
string areaOfExpertise;
public:
researchers();
researchers(int, string, string, int, string, string); //constructor
researchers(const researchers &research); //copy constructor
virtual ~researchers();//destructor
void settitle(string);
void setareaOfExpertise(string);
string gettitle();
string getareaOfExpertise();
//print function
virtual void printInfo();
};
#endif /* RESEARCHER_H_ */
******************************************************************************88
#include "Researcher.h"
researchers::researchers():Employee() {
title = "";
areaOfExpertise = "";
}
researchers::researchers(int employeeID, string namee, string positionn, int seniorityy, string titlee, string areaOfExpertisee) : Employee(employeeID, namee, positionn, seniorityy) {
title = titlee;
areaOfExpertise = areaOfExpertisee;
}
researchers::researchers(const researchers &research) :Employee(research) {
title = research.title;
areaOfExpertise = research.areaOfExpertise;
}
researchers::~researchers(){
cout << "Destructor called";
}
void researchers::settitle(string titlee) {
title = titlee;
}
void researchers::setareaOfExpertise(string areaOfExpertisee) {
areaOfExpertise = areaOfExpertisee;
}
string researchers::gettitle() {
return title;
}
string researchers::getareaOfExpertise() {
return areaOfExpertise;
}
void researchers::printInfo() {
cout << "Employee Id : " << getemployeeId() << endl;
cout << "Name :" << getname() << endl;
cout << "Position :" << getposition() << endl;
cout << "Seniority :" << getseniority() << endl;
cout << "Title :" << gettitle() << endl;
cout << "Area Of Expertise :" << getareaOfExpertise() << endl;
}
******************************************************************************************88
#ifndef ADMINISTRATOR_H_
#define ADMINISTRATOR_H_
#include
#include
#include "Employee.h"
using namespace std;
class Administrator :public Employee {
private:
int numberOfEmployees;
public:
Administrator();
Administrator(int, string, string, int, int); //constructor
Administrator(const Administrator & admin); //copy constructor
virtual ~Administrator();//destructor
void setnumberOfEmployees(int);
int getnumberOfEmployees();
virtual void printInfo();
};
#endif
*************************************************************************************************8
#include "Administrator.h"
#include
Administrator::Administrator():Employee() {
numberOfEmployees = 0;
}
Administrator::Administrator(int employeeID, string namee, string positionn, int seniorityy, int numOfEmployees): Employee(employeeID,namee,positionn,seniorityy) {
numberOfEmployees = numOfEmployees;
}
Administrator::Administrator(const Administrator &admin) : Employee(admin) {
numberOfEmployees = admin.numberOfEmployees;
}
Administrator::~Administrator() {
cout << "Destructor called" << endl;
}
void Administrator::setnumberOfEmployees(int numOfEmployees) {
numberOfEmployees = numOfEmployees;
}
int Administrator::getnumberOfEmployees() {
return numberOfEmployees;
}
void Administrator::printInfo() {
cout << "Employee Id : " << getemployeeId() << endl;
cout << "Name :" << getname() << endl;
cout << "Position :" << getposition() << endl;
cout << "Seniority :" << getseniority() << endl;
cout << "Number Of Employee : " << getnumberOfEmployees() << endl;
}
**************************************************************************************************8
#ifndef EMPLOYEEDB_H_
#define EMPLOYEEDB_H_
#include
#include
#include "Employee.h"
using namespace std;
class EmployeeDB{
private:
string Name;
string Address;
Employee *list; // items will point to the dynamically allocate
size_t numlist;
static const size_t LIST_SIZE = 150; // the maximum items in the list
public:
EmployeeDB()
{
list = new Employee[LIST_SIZE];
numlist = 0;
Name = "";
Address="";
}
//constructor
EmployeeDB(string aName,string anAddress)
{
list = new Employee[LIST_SIZE];
numlist = 0;
Name = aName;
Address= anAddress;
}
//copy contructor
EmployeeDB(const EmployeeDB & comp) {
list = new Employee[comp.LIST_SIZE];
numlist = 0;
Name = comp.Name;
Address= comp.Address;
for (int i = 0; i < numlist; i++) {
list[i] = comp.list[i];
}
}
//destructor
~EmployeeDB() {
delete[]list;
}
void EmployeeDB::setName(string aName) {
Name = aName;
}
void setAddress(string anAddress) {
Address = anAddress;
}
string getName(){
return Name;
}
string EmployeeDB::getAddress() {
return Address;
}
//adds a employee to the list
int numberOfEmployees() {
return numlist;
}
void listOfNames(){
for (int i = 0; i < numlist; i++){
cout<< "Employee location: " << i << " Name:" << list[i].getname() << endl;
}
}
//display all Employees of the list.
void printEmployeeDBInfo() const {
cout << Name << endl << Address << endl;
}
};
#endif
***************************************************************************************
#include
#include
#include "Employee.h"
#include "Employee.cpp"
#include "Administrator.h"
#include "Administrator.cpp"
#include "Researcher.h"
#include "Researcher.cpp"
#include "EmployeeDB.h"
using namespace std;
int main() {
//Creating Employee Objects of Researchers and Administrators.
Administrator a1(4825, "Riad", "Director", 4, 15);
Administrator a2;
researchers r1(4826, "Hussein", "scientist", 2, "professor", "Electrical");
researchers r2;
researchers r3(r1);
researchers r4(4829, "Nathalie", "scientist", 2, "professor", "Electrical");
Administrator a3(4825, "Jessica", "Assistant", 5, 16);
//Print function that display the informations of the employees.
cout << "||Print Employees Information||" << endl;
a1.printInfo();
a2.printInfo();
a3.printInfo();
r1.printInfo();
r2.printInfo();
r3.printInfo();
r4.printInfo();
cout<< endl<< endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
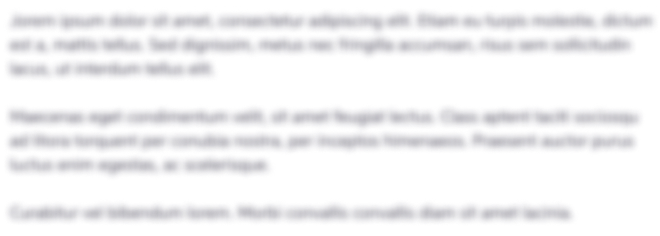
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started