Question
Please complete the following code. Thanks! Give an algorithm to find all nodes less than some value, X, in a binary heap. Your algorithm should
Please complete the following code. Thanks!
Give an algorithm to find all nodes less than some value, X, in a binary heap. Your algorithm should run in O(K), where K is the number of nodes output.
import java.util.Arrays;
public class HeapLessThan {
public static void heapify(int[] a) { int n = a.length; int last = getParent(n - 1); for (int p = last; p >= 0; p--) siftDown(a, p, n); }
public static void siftDown(int[] a, int p, int n) { // TODO }
public static void swap(int[] a, int i, int j) { int temp = a[i]; a[i] = a[j]; a[j] = temp; }
public static int getLeft(int p) { // TODO return 0; }
public static int getRight(int p) { return getLeft(p) + 1; }
public static int getParent(int p) { // TODO return 0; } public static void printLessThan(int[] heap, int x) { System.out.print("keys < " + x + ": "); // TODO System.out.println(); }
public static void main(String[] args) { int[] a = new int[] { 5, 13, 2, 25, 7, 17, 20, 8, 4, }; System.out.println(Arrays.toString(a)); heapify(a); for (int i = 0; i < a.length; i++) printLessThan(a, a[i]); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
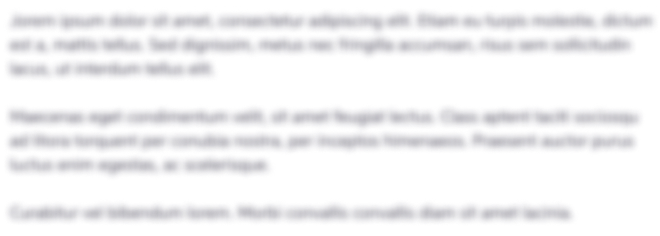
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started