Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please complete the following: Develop a simple Personal Diary Management System in Java that allows users to add new diary entries, view all entries, and
Please complete the following:
Develop a simple Personal Diary Management System in Java that allows users to add new diary entries, view all entries, and search for entries by date. This lab focuses on practicing file IO operations using ScannerFileReader and FileWriter classes no other IO classes as well as basic exception handling and resource management without the use of trywithresources syntax.
Part : Diary Entry Model
No need for a separate class model in this exercise. You will directly work with strings and file operations to manage diary entries.
Part : Implementing the Diary Management System
Add New Entry:
Implement a method to add a new diary entry. The user should input the date in the format YYYYMMDD and the content of the diary entry.
Use FileWriter to append this information to a file named diarytxt with the date and content separated by a symbol. Ensure each entry is on a new line.
View All Entries:
Implement a method to display all diary entries.
Use FileReader and Scanner to read from diarytxt parsing each line to separate the date and content. Print each entry with the date and content on separate lines.
Search Entries by Date:
Implement a method that prompts the user for a date and searches diarytxt for entries with that date, displaying the content of any entries found.
Part : Main Method and User Interface
Implement a textbased menu in the main method allowing users to choose an action add an entry, view all entries, search by date, or exit
Use a loop to keep the program running until the user chooses to exit.
#### Implementation Notes:
File IO Operations: Strictly use FileReader for reading files, FileWriter for writing to files, and Scanner for both reading user input and file content.
Resource Management: Manually manage resources opening and closing files without using the trywithresources statement. Ensure resources are closed properly in a finally block to avoid resource leaks.
Error Handling: Implement basic error handling to manage exceptions that may occur during file operations, providing userfriendly error messages.
#### Deliverables:
Source code for the DiaryManager class, implementing the specified functionalities.
A text file named diarytxt used by the application to store diary entries.
A brief report documenting your approach to implementing the lab, any challenges you faced, and how you resolved them.
#### Provisos:
Page of Sept
Do not use any classes for file IO operations other than ScannerFileReader and FileWriter
Avoid using the trywithresources syntax for resource management; instead, manually open and close your resources.
Ensure your application can handle exceptions gracefully, such as file not found errors or IO errors, without crashing.
This lab exercise is designed to strengthen your understanding of basic file operations in Java, manual resource management, and application of exception handling mechanisms.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
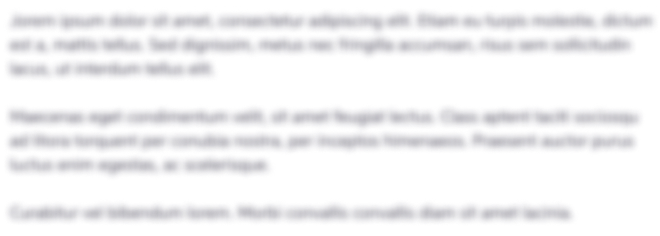
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started