Question
Please create a new Coin Calculator Android Java Project in Android Studio using the following directions: make a new Basic Activity project In activity_main.xml :
Please create a new Coin Calculator Android Java Project in Android Studio using the following directions:
- make a new Basic Activity project
- In activity_main.xml: please change the FAB's image to the media player
- In content_main.xml: create a CardView containing a TextView with a String like "Please enter in the coin count", for "Banner" purposes.
- Create four CardViews, one for each of Penny, Nickel, Dime and Quarter.
- Each CardView should contain:
- an ImageView of the coin (from drawables provided)
- below that, an EditText for the user to type in (numbers only) the amount of that coin
- Each CardView should contain:
- Center:
- Center horizontally the four coin CardViews in a ConstraintLayout
- Center the "Banner" CardView above the four coin cards, as shown. Please put in a margin above it, below the Toolbar.
- Create a "Status Bar" TextView constrained to the bottom of the window
- On app run, it should show SC initially
- Once the user clicks on the FAB, it should show the calculation results, as shown above.
- Create a menu with two items
- Clear All
- Always shown on Toolbar with an icon of your choice
- About
- Never shown on Toolbar; java code to run when clicked:
- Clear All
- Please make sure all Strings, dimensions, etc. are stored in their respective XML files in res/values, not hard-coded in layout XML files, etc.
The app should look like the image below:
MVC Model: CoinCounter.java
package com.android.coin_counter;
import com.google.gson.Gson;
public class CoinCounter { private int mCountOfPennies, mCountOfNickels, mCountOfDimes, mCountOfQuarters;
public int getCentsValueTotal () { return getCentsValueOfPennies () + getCentsValueOfNickels () + getCentsValueOfDimes () + getCentsValueOfQuarters (); }
public int getCentsValueOfPennies () { return mCountOfPennies; }
public int getCentsValueOfNickels () { return mCountOfNickels * 5; }
public int getCentsValueOfDimes () { return mCountOfDimes * 10; }
public int getCentsValueOfQuarters () { return mCountOfQuarters * 25; }
public int getCountOfPennies () { return mCountOfPennies; }
public void setCountOfPennies (int countOfPennies) { this.mCountOfPennies = Math.max(0, countOfPennies); }
public void setCountOfPennies (String countOfPennies) { this.mCountOfPennies = (countOfPennies == null || countOfPennies.equals ("")) ? 0 : Integer.parseInt (countOfPennies); }
public int getCountOfNickels () { return mCountOfNickels; }
public void setCountOfNickels (int countOfNickels) { this.mCountOfNickels = Math.max(0, countOfNickels); }
public void setCountOfNickels(String nickels) { this.mCountOfNickels = (nickels == null || nickels.equals ("")) ? 0 : Integer.parseInt (nickels); }
public int getCountOfDimes () { return mCountOfDimes; }
public void setCountOfDimes (int countOfDimes) { this.mCountOfDimes = Math.max(0, countOfDimes); }
public void setCountOfDimes(String dimes) { this.mCountOfDimes = (dimes == null || dimes.equals ("")) ? 0 : Integer.parseInt (dimes); }
public int getCountOfQuarters () { return mCountOfQuarters; }
public void setCountOfQuarters (int countOfQuarters) { this.mCountOfQuarters = Math.max(0, countOfQuarters); }
public void setCountOfQuarters(String quarters) { this.mCountOfQuarters = (quarters == null || quarters.equals ("")) ? 0 : Integer.parseInt (quarters); }
public static String getJSONStringFrom (CoinCounter currentCC) { return new Gson ().toJson (currentCC); }
public String getJSONStringFromThis() { return new Gson ().toJson (this); }
public static CoinCounter getCoinCounterObjectFromJSONString(String currentCC) { return new Gson ().fromJson (currentCC, CoinCounter.class); }
}
need to know how to use android studio to do it (using Java and xml)
Android Emulator - Square_API_29:5556 Android Emulator - Square_API_29:5556 10:33 @ 10:34 Coin Counter CLEAR ALL Coin Counter CLEAR ALL PLEASE ENTER IN THE COIN COUNT: PLEASE ENTER IN THE COIN COUNT: 0 0 0 0 8 6 1 3 SA Total in cents: 123Step by Step Solution
There are 3 Steps involved in it
Step: 1
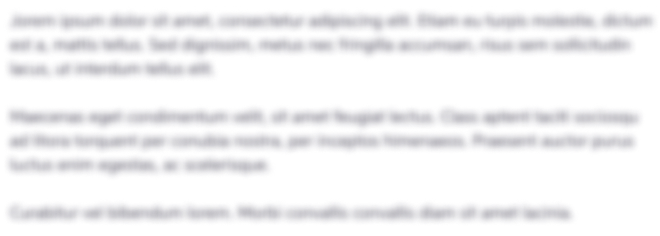
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started