Question
Please create one project for all the problems in this assignment. Recursive Sum of Numbers Write a recursive static method int recursiveSum(int num ) that
Please create one project for all the problems in this assignment.
Recursive Sum of Numbers
Write a recursive static method int recursiveSum(int num) that accepts a
positive integer argument and returns the sum of all the integers from 1 up to the number
passed as an argument. For example, if 50 was passed as an argument, the method will
return the sum: 1+2+3++50. Make the method throw IllegalArgumentException when
argument is less than 0.
Requirement:
*Your method must not use any global variables or static fields. Only local
variables can be used in the method implementation.
*Test the method in main(). Hardcode all your test cases in main().
*Add this new method to the project named Recursive.java
Recursive Sum of Array Elements
Write a recursive static method int recArraySum(int[] nums, int i) that
accepts an array of integers and an index i and returns the sum of all elements of that
array up to and including element with the index i.
Test the method in main(). Hardcode all your test cases in main().
Requirement:
*Your method must not use any global variables or static fields. Only local
variables can be used in the method implementation.
*Add this new method to the project named Recursive.java
Recursive Selection Sort
Convert the regular iterative selection sort method into the recursive static one. In order
to achieve that, replace the outer loop in the solution by recursive calls and leave the
second (inner) loop to be more or less as it is.
*Test your method in main().
*Make sure that your code works for arrays of size 1, 2, and 3 as well as a regular unsorted array. Do not use user input when testing the method hardcode all the test cases.
Requirement:
*Your method must not use any global variables or fields. Only local
variables can be used in the method implementation.
*Add this new method to the project named Recursive.java
Recursive Print
Implement method public static void printPattern(int n) that can
print a pattern of numbers in a shape of a pyramid, similar to the ones you see below.
n can be any positive integer number. Make the method throw IllegalArgumentException
when argument is less than or equal to 0.
Requirements:
1. There must be NO LOOP STRUCTURES used in your method implementation.
All repetitions must be implemented with the help of recursion. This means you
will need to write additional recursive as well as non-recursive methods to replace
nested loops needed in code.
2. Try having no more than 2 recursive methods in your implementation. The
number of non-recursive methods is unlimited.
3. Your methods must not use any global variables or static fields. Only local
variables can be used in the method implementations.
printPattern(5)
1
212
32123
4321234
543212345
printPattern(3)
1
212
32123
Test the method in main() by hardcoding all your test cases.
Add this new method to the project named Recursive.java
Step by Step Solution
There are 3 Steps involved in it
Step: 1
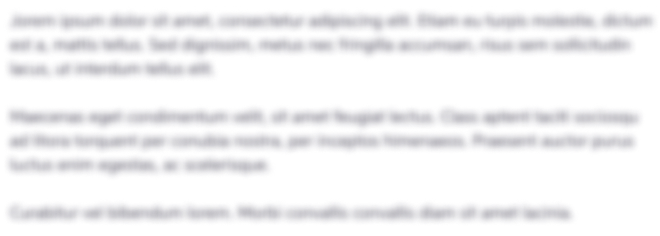
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started