Question
Please extend our Beetle Game and support both regular two-eyed beetles, four-eyed beetles, and four-eyed one-horn rhino beetles. Please 1) Design a class, named FourEyedBeetle,
Please extend our Beetle Game and support both regular two-eyed beetles, four-eyed beetles, and four-eyed one-horn rhino beetles.
Please
1) Design a class, named FourEyedBeetle, which extends the Beetle class.
Hints:
- Consider how to represent the inheritance relationship between Beetle class and the FourEyedBeetle class?
- Consider which properties and methods should be included in the FourEyedBeetle class?
2. adjust or implement the methods in the FourEyedBeetle class.
4) Adapt BeetleGame class for the FourEyedBeetle class
Hints:
- Consider how to create a specific beetle, based on condition (add one more constructor)
- Check whether we need to change play() and takeTurn() methods
5) Design a class, named FourEyedOneHornedRhinoBeetle, which extends the Beetle class.
Hints:
- Consider how to represent the inheritance relationship between FourEyedBeetle class and the FourEyedOneHornedRhinoBeetle class?
- Consider which properties and methods should be included in the FourEyedOneHornedRhinoBeetle class?
6) adjust or implement the methods in the FourEyedOneHornedRhinoBeetle class.
code
public class Beetle {
/** True if this Beetle has a body. */ protected boolean body;
/** Number of eyes this Beetle has, from 0-2. */ protected int eyes;
/** Number of feelers this Beetle has, from 0-2. */ protected int feelers;
/** True if this Beetle has a head. */ protected boolean head;
/** Number of legs this Beetle has, from 0-6. */ protected int legs;
/** True if this Beetle has a tail. */ protected boolean tail;
/** A new Beetle has no parts. */ public Beetle() { this.body=false; this.eyes=0; this.feelers=0; this.head=false; this.legs=0; this.tail=false; } /** Try to add a body and return whether this succeeded. */ public boolean addBody() { if(body){ return true; } else{ this.body=true; return true; } }
/** Try to add an eye and return whether this succeeded. */ public boolean addEye() { if(head&&(eyes<2)) { eyes++; return true; } else{ return false; } }
/** Try to add a head and return whether this succeeded. */ public boolean addHead() { if(body&&(head==false)) { head=true; return true; } else{ return false; } } /** Try to add a feeler and return whether this succeeded. */ public boolean addFeeler() { //TODO add a feeler if(head&&(feelers<2)) { feelers++; return true; } else { return false; } }
/** Try to add a leg and return whether this succeeded. */ public boolean addLeg() { if(body&&(legs<6)) { legs++; return true; } else{ return false; } } /** Try to add a tail and return whether this succeeded. */ public boolean addTail() { if(body&&(tail==false)) { tail=true; return true; } else{ return false; } }
/** Return true if that Beetle has the same parts as this one. */ public boolean equals(Object that) { if (this == that) { return true; } if (that == null) { return false; } if (getClass() != that.getClass()) { return false; } Beetle thatBeetle = (Beetle)that; return body == thatBeetle.body && eyes == thatBeetle.eyes && feelers == thatBeetle.feelers && head == thatBeetle.head && legs == thatBeetle.legs && tail == thatBeetle.tail; }
/** Return true if this Beetle has all of its parts. */ public boolean isComplete() { return(body&&(eyes==2)&&(feelers==2)&&head&&(legs==6)&&tail); } public String toString() { if (body) { String result = ""; if (feelers > 0) { result += "\\"; if (feelers == 2) { result += " /"; } result += " "; } if (head) { if (eyes > 0) { result += "o"; } else { result += " "; } result += "O"; if (eyes == 2) { result += "o"; } result += " "; } if (legs > 0) { result += "-"; } else { result += " "; } result += "#"; if (legs > 1) { result += "-"; } result += " "; if (legs > 2) { result += "-"; } else { result += " "; } result += "#"; if (legs > 3) { result += "-"; } result += " "; if (legs > 4) { result += "-"; } else { result += " "; } result += "#"; if (legs > 5) { result += "-"; } if (tail) { result += " v"; } return result; } else { return "(no parts yet)"; } }
}
-----------------
public class BeetleGame {
/** For reading from the console. */ public static final java.util.Scanner INPUT = new java.util.Scanner(System.in);
/** Player 1's Beetle. */ private Beetle bug1;
/** Player 2's Beetle. */ private Beetle bug2;
/** A die. */ private Die die;
/** Create the Die and Beetles. */ public BeetleGame() { bug1=new Beetle(); bug2=new Beetle(); die=new Die(6); }
/** Play until someone wins. */ public void play() { int player = 1; Beetle bug = bug1; while(bug.isComplete()==false) { if(takeTurn(player,bug)==false) { if(player==1){ player=2; bug=bug2; } else { player=1; bug=bug1; } } System.out.println(" Player " + player + " wins!"); System.out.println(bug); } }
/** * Take a turn for the current player. Return true if the player * earned a bonus turn. */ public boolean takeTurn(int player, Beetle bug) { System.out.println(" Player " + player + ", your beetle:"); System.out.println(bug); System.out.print("Hit return to roll: "); INPUT.nextLine(); die.roll(); System.out.print("You rolled a " + die.getTopFace()); //draw component according to the top value on the die switch (die.getTopFace()) { case 1: System.out.println(" (body)"); return bug.addBody(); case 2: System.out.println(" (head)"); return bug.addHead(); case 3: System.out.println(" (leg)"); return bug.addLeg(); case 4: System.out.println(" (eye)"); return bug.addEye(); case 5: System.out.println(" (feeler)"); return bug.addFeeler(); default: System.out.println(" (tail)"); return bug.addTail(); } }
/** Create and play the game. */ public static void main(String[] args) { System.out.println("Welcome to Beetle."); BeetleGame game = new BeetleGame(); game.play(); } } ----------------------------------
public class Die implements Comparable
/** The face of this Die that is showing. */ final static int FACE=6; private int topFace=1;
/** Initialize the top face to 1. */ public Die(int topFace) { this.topFace=topFace; }
private Die() { topFace=0; }
Die(Die die) { }
public int compareTo(Die that) { return topFace - that.topFace; } /** Return true if that Die has the same top face as this one. */ public boolean equals(Object that) { if (this == that) { return true; } if (that == null) { return false; } if (getClass() != that.getClass()) { return false; } Die thatDie = (Die)that; return topFace == thatDie.topFace; }
/** Return the top face of this Die. */ public int getTopFace() { return this.topFace; }
/** * Set the top face to a random integer between 1 and 6, inclusive. */ public void roll() { // roll the die this.topFace= ((int)(Math.random()*FACE))+1; }
/** Set the top face to the specified value. */ public void setTopFace(int topFace) { this.topFace = topFace; }
public String toString() { return "" + this.topFace; } /** Create a Die, print it, roll it, and print it again. */ public static void main(String[] args) { Die d = new Die(); System.out.println("Before rolling: " + d); d.roll(); System.out.println("After rolling: " + d); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
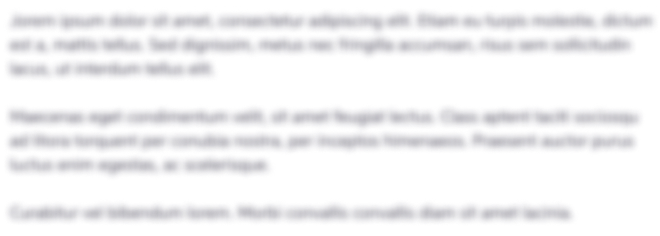
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started