Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please fill in the areas of JAVA, Do not edit any other code other than what is highlighted. import java.util.NoSuchElementException ; /** This program tests
Please fill in the areas of JAVA, Do not edit any other code other than what is highlighted.
import java.util.NoSuchElementException ; /** This program tests some methods of the LinkedList class, where the class is implemented with an inner class called Node. */ public class LinkedListTester { public static void main(String[] args) { LinkedList list = new LinkedList(); list.addFirst("C"); list.addFirst("A"); list.addFirst("T"); // print all elements System.out.println(list) ; System.out.println("-------------------Expected: [T, A, C]") ; System.out.println("" + list.getFirst() + list.getFirst()) ; System.out.println("-------------------Expected: TT") ; System.out.println("" + list.removeFirst() + list.removeFirst()) ; System.out.println("-------------------Expected: TA") ; try { list = new LinkedList() ; System.out.println(list.getFirst()) ; } catch (Exception e) { System.out.println(e) ; } System.out.println("-------------------Expected:") ; System.out.println("java.util.NoSuchElementException") ; System.out.println("-------------------") ; } } /** This is an example from Chapter 16, showing a partial implementation of the java.util.LinkedList class. A linked list is a sequence of links with efficient element insertion and removal. This class contains a subset of the methods of the standard java.util.LinkedList class. */ class LinkedList { private Node first ; private class Node { public Object data ; public Node next ; /** Initializes data and next to given values. @param data the given data @param next the given pointer */ public Node(Object data, Node next) { this.data = data ; this.next = next ; } } /** Adds an element to the front of the linked list. @param element the object to add */ public void addFirst(Object element) { //-----------Start below here. To do: approximate lines of code = 1 // 1. fill in this method //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } /** Returns the first element in the linked list. @return the first element in the linked list */ public Object getFirst() { //-----------Start below here. To do: approximate lines of code = 2 // 2-3. fill in this method, remembering NSEE //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } /** Removes the first element in the linked list. @return the removed element */ public Object removeFirst() { if (first == null) throw new NoSuchElementException() ; //-----------Start below here. To do: approximate lines of code = 3 // 4-6. fill in this method //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } /** Gives a string representation of the linked list. Format: [a, b, c, d] when the elements are a, b, c, d. [] when the list is empty. @return string representation of the linked list. */ public String toString() { String result = "[" ; Node current = first ; while (current != null) { if (current.next != null) result += current.data + ", " ; else result += current.data + "]" ; current = current.next ; } return result ; } /** Returns an iterator for iterating through this list. @return an iterator for iterating through this list */ public ListIterator listIterator() { return new LinkedListIterator() ; } /** Inner class implementation of the list iterator After a next(), position will point to the node whose data is returned and previous will point to the node prior to that, if there is one, and isAfterNext will be true, which allows for a remove() or a set(). */ private class LinkedListIterator implements ListIterator { private Node position ; private Node previous ; private boolean isAfterNext ; /** Constructs an iterator that is at the front of the linked list */ public LinkedListIterator() { position = null ; previous = null ; isAfterNext = false ; } /** Tests if there is an element after the iterator position. @return true if there is an element after the iterator position */ public boolean hasNext() { //stub return false ; } /** Moves the iterator position to the next element and returns the data of that element. If there is no element after the current position, then throw a NoSuchElementException @return the traversed element */ public Object next() { //stub return null ; } /** Adds an element before the iterator position and moves the iterator position to the inserted element. @param element the object to add */ public void add(Object element) { //stub } /** Removes the last traversed element. This method may only be called after a call to the next() method. */ public void remove() { //stub } /** Sets the last traversed element to a different value @param element the object to set */ public void set(Object element) { //stub } } } /** A list iterator allows access of a position in a linked list. This interface contains a subset of the methods of the standard java.util.ListIterator interface. The methods for backward traversal are not included. */ interface ListIterator { /** Moves the iterator past the next element. @return the traversed element */ Object next(); /** Tests if there is an element after the iterator position. @return true if there is an element after the iterator position */ boolean hasNext(); /** Adds an element before the iterator position and moves the iterator past the inserted element. @param obj the object to add */ void add(Object obj); /** Removes the last traversed element. This method may only be called after a call to the next() method. */ void remove(); /** Sets the last traversed element to a different value. @param obj the object to set */ void set(Object obj); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
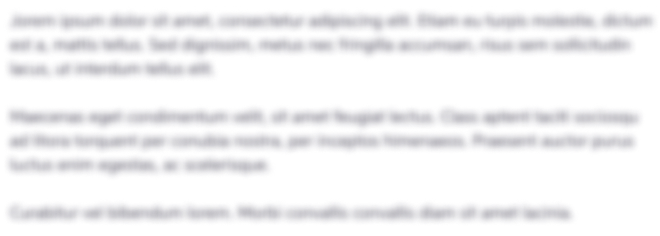
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started