Question
Please finish this code. Thank you! import java.util.Arrays; import java.util.Comparator; import java.util.Random; public class KthLargest { /** * Suppose you have a group of N
Please finish this code. Thank you!
import java.util.Arrays;
import java.util.Comparator;
import java.util.Random;
public class KthLargest {
/**
* Suppose you have a group of N numbers and would like to
* determine the kth largest. This is known as the selection problem.
*
* One way to solve this problem would be to read the N numbers into an
* array, sort the array in decreasing order by some simple algorithm
* such as bubblesort, and then return the element in position k.
*/
public static int kthLargest1(int[] a, int k) {
assert a != null;
int n = a.length;
assert n > 0 && k <= n;
Integer[] b = new Integer[n];
for (int i = 0; i < n; i++)
b[i] = a[i];
Arrays.sort(b, new Comparator() {
public int compare(Integer x, Integer y) {
return y.compareTo(x);
}
});
return b[k - 1];
}
/**
* A somewhat better algorithm might be to read the first
* k elements into an array and sort them (in decreasing order). Next,
* each remaining element is read one by one. As a new element arrives,
* it is ignored if it is smaller that the kth element in the array.
* Otherwise, it is placed in its correct spot in the array, bumping
* one element out of the array. When the algorithm ends, the element
* in the kth position is returned as the answer.
*/
public static int kthLargest2(int[] a, int k) {
// TODO
return 0;
}
public static void main(String[] args) {
// If you've enabled asserts properly, the following will fail.
assert true == false; // Delete this once you know assert works.
int[] a, b;
a = new int[] { 8, 7, -3, 9, 2, 1, -5, 4, 12, -2 };
b = new int[] { 12, 9, 8, 7, 4, 2, 1, -2, -3, -5 };
for (int i = 0; i < a.length; i++)
assert kthLargest1(a, i + 1) == b[i];
System.out.println("kthLargest1 passes basic checks!");
for (int i = 0; i < a.length; i++)
assert kthLargest2(a, i + 1) == b[i];
System.out.println("kthLargest2 passes basic checks!");
// TODO: Conduct your timing experiments here.
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
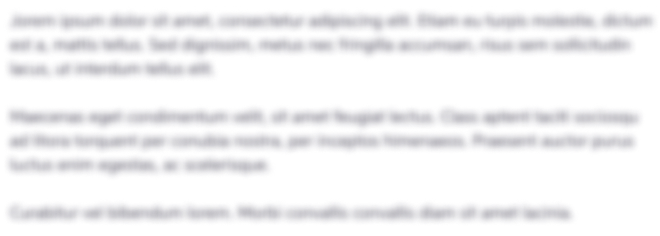
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started