Question
please fix the following program so it can be able to edit, delete and check the reservation anytime I want. please add the do while
please fix the following program so it can be able to edit, delete and check the reservation anytime I want. please add the do while loop to this program and catch any error.
public class TestHotelReservation {
private static Scanner input;
public static void main(String[] args) {
// display menu
displayMenu();
// take input from user
int option = takeInput();
// process user input
processInput(option);
}
public static void displayMenu() {
System.out.println();
System.out.println(" ::=======================================:: ");
System.out.println(" :::: WELCOME TO THE RITZ CARLTON HOTEL RESERVATION SYSTEM :::: ");
System.out.println(" :::: GRAND CAYMAN, CAYMAN ISLANDS :::: ");
System.out.println(" ::=======================================:: ");
System.out.println();
System.out.println(" We are recognized as the finest business hotel group for our elegance and courteous service..");
System.out.println();
System.out.println(" ::::: RULES & REGULATIONS ::::: ");
System.out.println(" Check-in is at 3:00 PM check-out is at 11:00 AM, If check-out time is exceeded, additional charges will apply. ");
System.out.println(" When making the reservation, please present your ID proof. ");
System.out.println(" We only accept cash or credit cards for the payment of bills; personal checks will not be accepted. ");
System.out.println();
System.out.println(" Would you like to book a room in our hotel? ");
System.out.print(" Enter Your Choice : ");
System.out.println();
System.out.println("1. Enter reservation");
System.out.println("2. View reservation");
System.out.println("3. Modify reservation");
System.out.println("4. Room availability");
System.out.println("5. Delete reservation");
System.out.println("6. Save reservation");
System.out.println("7. Exit");
}
public static int takeInput() {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter an option: ");
int option = scanner.nextInt();
return option;
}
public static void processInput(int option) {
switch (option) {
case 1:
EnterReservation();
break;
case 2:
ViewReservation();
break;
case 3:
ModifyReservation();
break;
case 4:
RoomAvailability();
break;
case 5:
DeleteReservation();
break;
case 6:
Savereservation();
break;
case 7:
// exit();
System.out.println("Thank you for using Hotel Reservation System");
break;
default:
System.out.println("Invalid option");
}
}
private static void Savereservation() {
// TODO Auto-generated method stub
System.out.println("Save Reservation");
System.out.println("-------------------------------");
}
private static void ViewReservation() {
// TODO Auto-generated method stub
System.out.println("View reservation");
System.out.println("-------------------------------");
}
public static void ModifyReservation() {
System.out.println("Modify reservation");
System.out.println("-------------------------------");
}
public static void RoomAvailability() {
System.out.println("Room availability");
System.out.println("-------------------------------");
}
public static void DeleteReservation() {
System.out.println("Delete reservation");
System.out.println("-------------------------------");
}
private static void EnterReservation() {
// TODO Auto-generated method stub
System.out.println("Enter reservation");
System.out.println("-------------------------------");
Scanner input = new Scanner(System.in);
System.out.print("Name: ");
String name = input.nextLine();
System.out.print("Date of birth: ");
String dateOfBirth = input.nextLine();
System.out.print("Citizenship ID proof: ");
String citizenshipIdProof = input.nextLine();
System.out.print("Address: ");
String address = input.nextLine();
System.out.print("Start date: ");
String startDate = input.nextLine();
System.out.print("End date: ");
String endDate = input.nextLine();
System.out.print("Phone number: ");
String phoneNumber = input.nextLine();
System.out.print("Reservation was Successful: ");
System.out.println();
System.out.print("Thank You! ");
}
}
class Reservation {
// attributes
private String name;
private String dateOfBirth;
private String citizenshipIdProof;
private String address;
private String startDate;
private String endDate;
private String phoneNumber;
private String Savereservation;
// constructor
public Reservation(String name, String dateOfBirth, String citizenshipIdProof, String address, String startDate,
String endDate, String phoneNumber) {
super();
this.name = name;
this.dateOfBirth = dateOfBirth;
this.citizenshipIdProof = citizenshipIdProof;
this.address = address;
this.startDate = startDate;
this.endDate = endDate;
this.phoneNumber = phoneNumber;
}
// getters and setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(String dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public String getCitizenshipIdProof() {
return citizenshipIdProof;
}
public void setCitizenshipIdProof(String citizenshipIdProof) {
this.citizenshipIdProof = citizenshipIdProof;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getStartDate() {
return startDate;
}
public void setStartDate(String startDate) {
this.startDate = startDate;
}
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
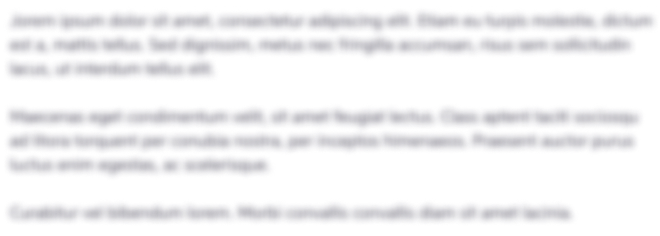
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started