Question
Please follow the instructions below: 1. Please add whats listed below to the code that will be provided under this 2. Add a new class
Please follow the instructions below:
1. Please add whats listed below to the code that will be provided under this
2. Add a new class called PayrollSystem_Phase2.
3. Add the main method provided below.
4. Add another method called parseEmployeePaychecks that has two parameters: one of type int and one of type String. The method returns an ArrayList of Paycheck elements.The int parameter represents an employee id, whereas the String parameter represents the list of paychecks the employee has received and which the method converts to an ArrayList of Paycheck elements.
5. You may assume that the String parameter has the following format:
periodBeginDate1:periodEndDate1:grossAmount1:taxAmount1:bonusAmount1# periodBeginDate2:periodEndDate2:grossAmount2:taxAmount2:bonusAmount2#...
6. Im providing you with an algorithm for the method below:
Below find original code:
Company.java
package payrollsystem_phase1;
import java.util.ArrayList;
/** * The Company class represents a company having multiple departments. * * @author Mayelin */ public class Company { // instance variables private String companyName; private ArrayList
/** * The getCompanyName method returns the company's name. * @return The company's name. */ public String getCompanyName() { return companyName; }
/** * The getDepartmentList method returns the company's list of departments. * @return The company's list of departments as an ArrayList of Department elements. */ public ArrayList
/** * The setCompanyName method sets the name for this company. * @param name The value to store in the name field for this company. */ public void setCompanyName(String name) { companyName = name; }
/** * The setDepartmentList method sets the list of departments for this company. * @param departments The value, as an ArrayList of Department elements, to store * in the list of departments field for this company. */ public void setDepartmentList(ArrayList
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Department.java
package payrollsystem_phase1;
import java.util.ArrayList;
/** * The Department class represents a department within a company. Departments have a * list of employees and a manager. * * @author Mayelin */ public class Department { // instance variables private int departmentID; private String departmentName; private Manager departmentManager; private ArrayList
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Employee.java
package payrollsystem_phase1;
import java.util.ArrayList;
/** * The Employee class is an abstract class that holds general data about a company's * employee. Classes representing more specific types of employees should inherit * from this class. * * @author Mayelin */ public abstract class Employee { // instance variables private int employeeID; private String firstName; private String lastName; private ArrayList
}
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Hourly Employee.java
package payrollsystem_phase1;
import java.util.*;
/** * The HourlyEmployee class is a subclass of the Employee class. It represents * employees that get paid by the hour. * * @author Mayelin */ public class HourlyEmployee extends Employee { // instance variables private double hourlyRate; private double periodHours; /** * This constructor sets the hourly employee's id, name, list of paychecks * received, hourly rate, and period hours. * @param id The employee's identification number. * @param first The employee's first name. * @param last The employee's last name. * @param paychecks The list of paychecks the employee has received so far. * @param rate The employee's hourly rate. * @param periodHrs The number of hours the employee works during a pay period. */ public HourlyEmployee(int id, String first, String last, ArrayList
hourlyRate = rate; periodHours = periodHrs; } /** * This is a copy constructor. It initializes the fields of the object being * created to the same values as the fields in the object passed as an argument. * @param hourlyEmpObj The object being copied. */ public HourlyEmployee(HourlyEmployee hourlyEmpObj) { super(hourlyEmpObj); if( hourlyEmpObj != null ) { hourlyRate = hourlyEmpObj.hourlyRate; periodHours = hourlyEmpObj.periodHours; } } /** * The getHourlyRate method returns the rate that the employee gets paid per hour. * @return The employee's hourly rate. */ public double getHourlyRate() { return hourlyRate; } /** * The getPeriodHours method returns the number of hours the employee works * during a pay period. * @return The employee's period hours. */ public double getPeriodHours() { return periodHours; } /** * The setHourlyRate method sets the rate that the employee gets paid per hour. * @param rate The value to store in the hourly rate field. */ public final void setHourlyRate(double rate) { hourlyRate = rate; } /** * The setPeriodHours method sets the number of hours the employee works * during a pay period. * @param periodHrs The value to store in the period hours field. */ public final void setPeriodHours(double periodHrs) { periodHours = periodHrs; } /** * The toString method returns a string containing the state of an HourlyEmployee object. * @return A string containing the employee's information: id, first name, * last name, list of paychecks received, hourly rate, and period hours. */ @Override public String toString() { return super.toString() + String.format( "%5s %-24s %-20s %5s %-24s %-20s", "", "Hourly Rate:", hourlyRate, "", "Period Hours:", periodHours ); } }
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Manager.java
package payrollsystem_phase1;
import java.util.ArrayList;
/** * The Manager class is a subclass of the SalariedEmployee class. It represents * salaried employees who also manage a department. * * @author Mayelin */ public class Manager extends SalariedEmployee { // instance variable private double bonus; /** * This constructor sets the manager's id, name, list of paychecks received, * annual salary, and weekly bonus. * @param id The manager's identification number. * @param first The manager's first name. * @param last The manager's last name. * @param paychecks The list of paychecks the manager has received so far. * @param salary The manager's annual salary. * @param bonus The manager's weekly bonus. */ public Manager(int id, String first, String last, ArrayList
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Paycheck.java
package payrollsystem_phase1;
/** * The Paycheck class represents a paycheck paid to an employee for a pay period. * * @author Mayelin */ public class Paycheck { // instance variables private int employeeID; private String periodBeginDate; private String periodEndDate; private double grossAmount; private double taxAmount; private double bonusAmount; private double netAmount; /** * The constructor sets the paycheck's employee id, begin and end dates for * the pay period, as well as the gross, tax, bonus and net amounts. * @param empID Identification number of the employee receiving this paycheck. * @param beginDate Begin date for this paycheck's pay period. * @param endDate End date for this paycheck's pay period. * @param grossAmt Gross amount paid in this paycheck. * @param taxAmt Tax amount deducted in this paycheck. * @param bonusAmt Bonus amount paid in this paycheck. * @param netAmt Net amount paid in this paycheck. */ public Paycheck(int empID, String beginDate, String endDate, double grossAmt, double taxAmt, double bonusAmt, double netAmt) { employeeID = empID; periodBeginDate = beginDate; periodEndDate = endDate; grossAmount = grossAmt; taxAmount = taxAmt; bonusAmount = bonusAmt; netAmount = netAmt; }
/** * This is a copy constructor. It initializes the fields of the object being * created to the same values as the fields in the object passed as an argument. * @param paycheckObj The object being copied. */ public Paycheck(Paycheck paycheckObj) { if( paycheckObj != null ) { employeeID = paycheckObj.employeeID; periodBeginDate = paycheckObj.periodBeginDate; periodEndDate = paycheckObj.periodEndDate; grossAmount = paycheckObj.grossAmount; taxAmount = paycheckObj.taxAmount; bonusAmount = paycheckObj.bonusAmount; netAmount = paycheckObj.netAmount; } } /** * The getEmployeeID method returns the identification number of the employee * receiving this paycheck. * @return The employee's id. */ public int getEmployeeID() { return employeeID; }
/** * The getPeriodBeginDate method returns the begin date for this paycheck's pay period. * @return The pay period's begin date. */ public String getPeriodBeginDate() { return periodBeginDate; }
/** * The getPeriodEndDate method returns the end date for this paycheck's pay period. * @return The pay period's end date. */ public String getPeriodEndDate() { return periodEndDate; }
/** * The getGrossAmount method returns the gross amount paid in this paycheck. * @return The paycheck's gross amount. */ public double getGrossAmount() { return grossAmount; }
/** * The getTaxAmount method returns the tax amount deducted in this paycheck. * @return The paycheck's tax amount. */ public double getTaxAmount() { return taxAmount; }
/** * The getBonusAmount method returns the bonus amount paid in this paycheck. * @return The paycheck's bonus amount. */ public double getBonusAmount() { return bonusAmount; }
/** * The getNetAmount method returns the net amount that the employee is getting paid in this paycheck. * @return The paycheck's net amount. */ public double getNetAmount() { return netAmount; }
/** * The setEmployeeID method sets the identification number of the employee * receiving this paycheck. * @param empID The value to store in the employee ID field. */ public void setEmployeeID(int empID) { employeeID = empID; }
/** * The setPeriodBeginDate method sets the begin date for this paycheck's pay period. * @param beginDate The value to store in the pay period begin date field for this paycheck. */ public void setPeriodBeginDate(String beginDate) { periodBeginDate = beginDate; }
/** * The setPeriodEndDate method sets the end date for this paycheck's pay period. * @param endDate The value to store in the pay period end date field for this paycheck. */ public void setPeriodEndDate(String endDate) { periodEndDate = endDate; }
/** * The setGrossAmount method sets the gross amount for this paycheck. * @param payAmt The value to store in the gross amount field for this paycheck. */ public void setGrossAmount(double payAmt) { grossAmount = payAmt; }
/** * The setTaxAmount method sets the tax amount for this paycheck. * @param taxAmount The value to store in the tax amount field for this paycheck. */ public void setTaxAmount(double taxAmount) { this.taxAmount = taxAmount; }
/** * The setBonusAmount method sets the bonus amount for this paycheck. * @param bonusAmount The value to store in the bonus amount field for this paycheck. */ public void setBonusAmount(double bonusAmount) { this.bonusAmount = bonusAmount; }
/** * The setNetAmount method sets the net amount for this paycheck. * @param netAmount The value to store in the net amount field for this paycheck. */ public void setNetAmount(double netAmount) { this.netAmount = netAmount; } /** * The toString method returns a string containing the paycheck's data. * @return A String containing the Paycheck's information: employee id, * pay period begin and end dates, as well as gross, tax, bonus, and net amounts. */ @Override public String toString() { String separator = String.format(" %30s %031d", "", 0).replace('0', '-'); return String.format( " %30s %-20s %10s %30s %-20s %10s %30s %-20s %10s " + " %30s %-20s %10s %30s %-20s %10s %30s %-20s %10s " + " %30s %-20s %10s", "", "Employee ID:", employeeID, "", "Period Begin Date:", periodBeginDate, "", "Period End Date:", periodEndDate, "", "Gross Amount:", grossAmount, "", "Tax Amount:", taxAmount, "", "Bonus Amount:", bonusAmount, "", "Net Amount:", netAmount) + separator; }
}
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PayrollSystem_Phase1.java
package payrollsystem_phase1;
import java.util.*;
public class PayrollSystem_Phase1 { public static void main(String[] args) { // We cannot instantiate the Employee class since it is abstract System.out.println("/*********************************************************************/"); System.out.println("/* Testing the HourlyEmployee class */"); System.out.println("/*********************************************************************/"); // Creating an HourlyEmployee object using the first constructor HourlyEmployee employee_1 = new HourlyEmployee(1, "Janette", "Hernandez", null, 14.75, 30); // Creating an HourlyEmployee object using the copy constructor HourlyEmployee employee_2 = new HourlyEmployee(employee_1); // Calling some of the setter methods in the HourlyEmployee class. employee_2.setEmployeeID(2); employee_2.setFirstName("Marcela"); employee_2.setLastName("Brown"); employee_2.setHourlyRate(25); employee_2.setPeriodHours(40); System.out.println(" Calling some of the getter methods in the HourlyEmployee class..."); System.out.println( String.format("%-30s%s", "Employee ID:", employee_1.getEmployeeID()) ); System.out.println( String.format("%-30s%s", "First Name:", employee_1.getFirstName()) ); System.out.println( String.format("%-30s%s", "Last Name:", employee_1.getLastName()) ); System.out.println( String.format("%-30s%s", "Hourly Rate:", employee_1.getHourlyRate()) ); System.out.println( String.format("%-30s%s", "Period Hours:", employee_1.getPeriodHours()) ); System.out.println("/*********************************************************************/"); System.out.println("/* Testing the Paycheck class */"); System.out.println("/*********************************************************************/"); // create two Paycheck objects and add them to an ArrayList Paycheck janettesPaycheck_1 = new Paycheck(1, "01/01/2018", "01/07/2018", 442.50, 66.38, 0.0, 376.12); Paycheck janettesPaycheck_2 = new Paycheck(janettesPaycheck_1); janettesPaycheck_2.setPeriodBeginDate("01/08/2018"); janettesPaycheck_2.setPeriodEndDate("01/14/2018");
ArrayList
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
SalariedEmployee.java
package payrollsystem_phase1;
import java.util.ArrayList;
/** * The SalariedEmployee class is a subclass of the Employee class. It represents * employees that get paid an annual salary. * * @author Mayelin */ public class SalariedEmployee extends Employee { // instance variable private double annualSalary; /** * This constructor sets the salaried employee's id, name, date of birth, list of * paychecks received, and annual salary. * @param id The employee's identification number. * @param first The employee's first name. * @param last The employee's last name. * @param paychecks The list of paychecks the employee has received so far. * @param salary The employee's annual salary. */ public SalariedEmployee(int id, String first, String last, ArrayList
annualSalary = salary; } /** * This is a copy constructor. It initializes the fields of the object being * created to the same values as the fields in the object passed as an argument. * @param salariedEmpObj The object being copied. */ public SalariedEmployee(SalariedEmployee salariedEmpObj) { super(salariedEmpObj); if( salariedEmpObj != null ) { annualSalary = salariedEmpObj.annualSalary; } } /** * The getAnnualSalary method returns the salary that the employee gets paid * per year. * @return The employee's annual salary. */ public double getAnnualSalary() { return annualSalary; } /** * The setAnnualSalary method sets the salary that the employee gets paid * per year. * @param salary The value to store in the annual salary field. */ public final void setAnnualSalary(double salary) { annualSalary = salary; } /** * The toString method returns a string containing the state of a SalariedEmployee * object. * @return A string containing the employee's information: id, first name, * last name, list of paychecks received, and annual salary. */ @Override public String toString() { return super.toString() + String.format("%5s %-24s %-20s ", "", "Annual Salary:", annualSalary ); } }
public static ArrayListStep by Step Solution
There are 3 Steps involved in it
Step: 1
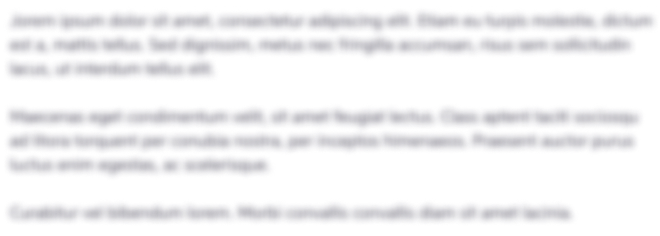
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started